Google Analytics is a service provided by Google that makes it easy to track what users do. In this tutorial, learn how to track Android application events like screen loads and button clicks in order to determine what your application's users are doing—and what they're not!
The Google Analytics SDK for Android provides helpful classes and methods to track user activity and generate useful statistics about your Android app activities. Here is a typical custom dashboard for some application behavior.
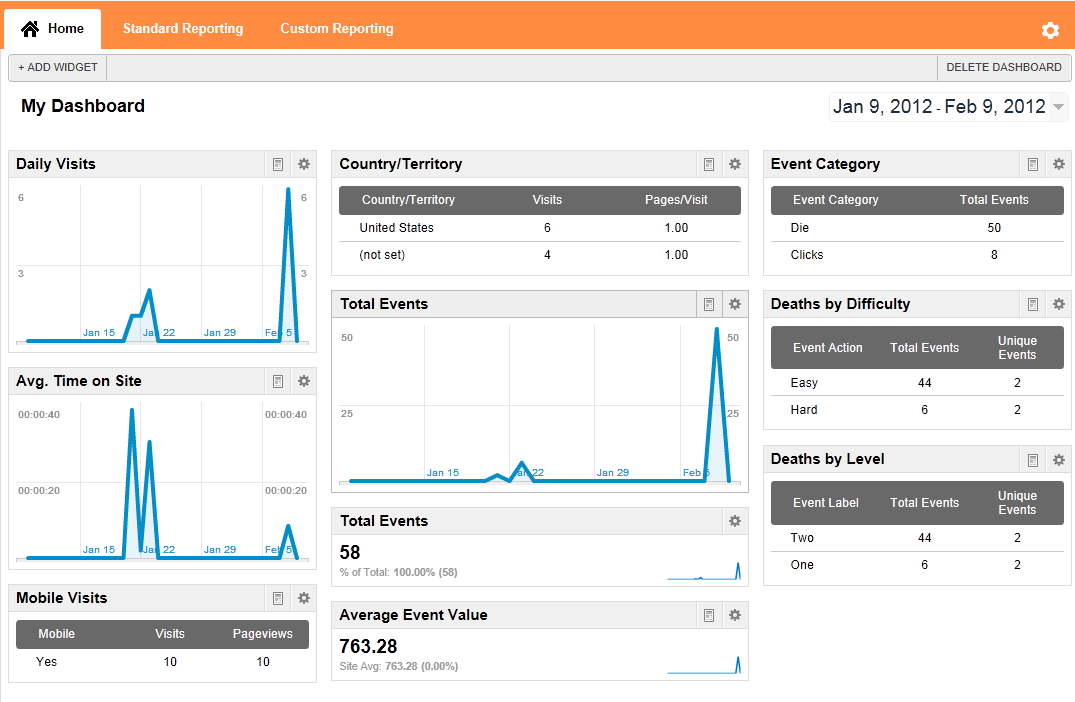
Step 1: Getting Started
There’s no point in hooking up Google Analytics unless you’ve already designed an Android application. Therefore, you’ll want to start with an application that is already pretty complete, in order to add statistics gathering in the appropriate code locations. This tutorial assumes you have an existing Android project in Eclipse to work from, that you have properly installed the Google Analytics SDK for Android, as described in "Android App Publication: Enabling Google Analytics to Gather App Statistics", and that you have signed up for a Google Analytics account.
Note: This tutorial is based upon the latest version of the Google Analytics for Android SDK Release 2 (in the Android SDK Manager), with Version 1.4.2 listed in the ReadMe.txt file and on the website download link.
Step 2: Starting a Tracking Session
In order to collect statistics, your application must be running a tracking session. All tracking must occur during this session. Typically, you'll start your tracker somewhere like your Activity class onCreate() or onResume() .
To start a tracking session, you'll need to import the tracker:
import com.google.android.apps.analytics.GoogleAnalyticsTracker;
Get an instance of the tracker:
GoogleAnalyticsTracker tracker = GoogleAnalyticsTracker.getInstance();
Start the tracker with a valid Google Analytics user account token. Here we configure the tracker to send data to the Google Analytics servers every 30 seconds:
tracker.startNewSession("UA-12345678-9", 30, this);
Step 3: Tracking Application Activity – An Overview
Once you have a tracking session up and running, tracking events is relatively straightforward. Here are some tips for good tracking:
- Understand that tracking is only effective if you put the statistics collection hooks in the right places in your app. This usually means understanding the callbacks associated with the user events in your application and dropping the even tracking code in at the precise moment intended. For example, you typically wouldn't want to track Button hover events, but you might want to track clicks.
- Once you have identified the right place for the hook, make sure you send the right (unique) data to the Google Analytics servers. All tracking methods have developer-defined parameters, mostly Strings, which can be used to provide details about the event being tracked. You will want to play around with the details you send to the server and what sort of reports you can generate with that data. There's no one right answer here.
- Tracking is like logging—it will affect performance, so use it wisely. Collect events and send them to the server in batches. If possible, trigger the upload during a time when your application is awake and using the network anyway.
- If you use Google Analytics tracking in your published applications you MUST inform the user that you are collecting their data. Collect only the information you need. Consider statistics collection like an anonymous survey – generic data that cannot be tied back to a specific user.
Step 4: Tracking Activity or Screen Hits
During a valid tracking session, you can track screen views by supplying the name of the Activity or screen using the trackPageView() method:
tracker.trackPageView("/Splash-Screen");
This method takes a simple developer-defined String value and logs the "view" to the Google Analytics server. You'll want to ensure that you define unique names for each item you want to track using this method. We recommend defining all Strings used by this method as constants in a single location, so that it is also straightforward to determine what screens or Activities are not receiving hits, as this information is just as valuable as those screens that receive a lot of traffic.
Step 5: Tracking User Events
During a valid tracking session, you can track user events of any type using the trackEvent() method:
tracker.trackEvent("Clicks", "Button", "Easy", 0); tracker.trackEvent("Completions", "Game-Deaths", "Hard-Level-One", 15); tracker.trackEvent("Die", "Easy", " Two", someNum);
Again, this method takes a flexible set of developer-defined parameters, all of which can be used to create interesting drill-down reports on the Google Analytics dashboard. How you organize your statistics is up to you, but the parameters are basically in hierarchical order.
The trackEvent() method takes four parameters:
- A category (required) – this String defines the event category. You might define event categories based on the class of user actions, like clicks or gestures or voice commands, or you might define them based upon the features available in your application (play, pause, fast forward, etc.).
- An action (required) – this String defines the specific event action within the category specified. In the example, we are basically saying that the category of the event is user clicks, and the action is a button click.
- A label (optional)– this String defines a label associated with the event. For example, if you have multiple Button controls on a screen, you might use the label to specify the specific View control identifier that was clicked.
- A value (optional) – this integer defines a numeric value associated with the event. For example, if you were tracking "Buy" button clicks, you might log the number of items being purchased, or their total cost.
While these are what the reports call the values, you really can map them however you'd like. It's best to be consistent within a particular application. For example, the second two log events shown above are equivalent, but organized differently. We logged a bunch of the last one; see the figure below.
This report is a good example of how the value field shows up in the report. It's both accumulated into a total across all events as well as averaged. You have to decide for yourself if you want or need the value to be meaningful for each view of categories, actions, and labels. The screenshot shown demonstrates that the value we used may only be meaningful when view labels. This value could represent time to completion. It could represent score. It could represent a count of something. It's yours to define. But, define it well up front or, if you change it, change the tracking code and move to new reports. You can't fix up old data.
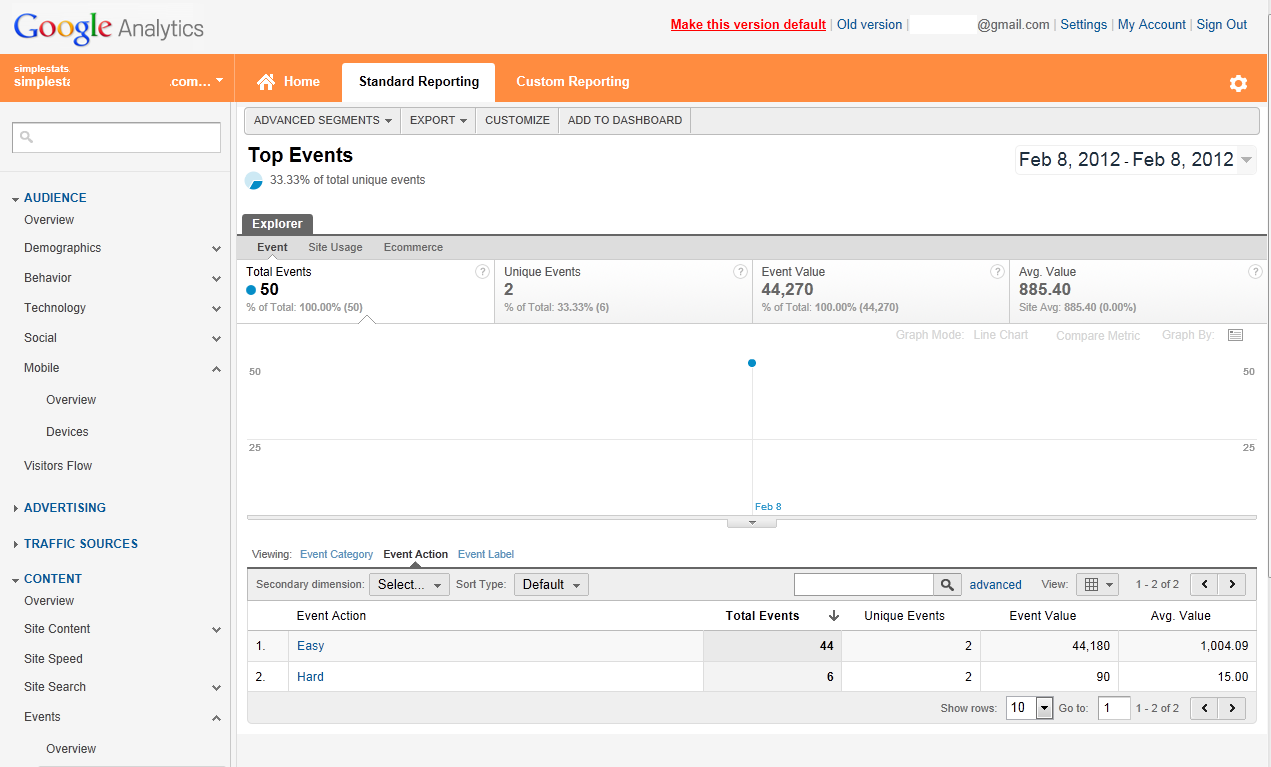
Step 6: Ending a Tracking Session
Typically, you'll terminate the session in your Actvitiy's onPause() or onDestroy() methods, like this:
tracker. stopSession();
Conclusion
The Google Analytics SDK for Android is an easy way to help determine how your users are using your Android applications. There are several different event tracking methods which can be used to determine what parts of the application are being used, as well as what features of the application your users use routinely or rarely. The data being sent to the Google servers should be generic enough to protect users' privacy but specific enough to generate useful reports for the developer. This is a balancing act that usually requires some tweaking on an app-by-app basis.
As always, we look forward to your feedback.
About the Authors
Mobile developers Lauren Darcey and Shane Conder have coauthored several books on Android development: an in-depth programming book entitled Android Wireless Application Development, Second Edition and Sams Teach Yourself Android Application Development in 24 Hours, Second Edition. When not writing, they spend their time developing mobile software at their company and providing consulting services. They can be reached at via email to [email protected], via their blog at androidbook.blogspot.com, and on Twitter @androidwireless.
Comments