The best way to learn JavaScript is to check out our new and comprehensive guide. Whether you're just getting started as a web developer or you want to explore more advanced topics, check out:
Or jump right in and watch our JavaScript courses:
- JavaScriptJavaScript Fundamentals
- ES6JavaScript ES6 Fundamentals
- JavaScriptJavaScript for Web Designers
- Web DevelopmentHow to Become a Web Developer: jQuery and Bootstrap
Learning something new is scary. For me, the biggest issue with picking up a new skill is that I don’t know what I don’t know. Given that, it’s often useful to find a plan for learning whatever you’re interested in. That’s what this post is: your blueprint, your roadmap, your plan of action for learning JavaScript! You don’t have to worry about finding the best resources, sorting out the bad ones, and figuring out what to learn next. It’s all here. Just follow it, step by step.
And if, at any stage, you feel stuck, you can get help from one of the JavaScript experts on Envato Studio.
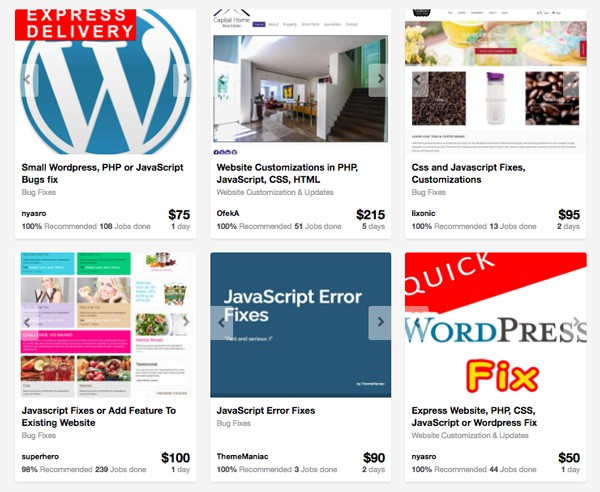
Assignment 0: Understand what JavaScript Is and Isn’t
JavaScript is the language of the browser.
Before you actually begin learning JavaScript, take a minute to understand what it is and does.
JavaScript is not jQuery, Flash, or Java. It’s a programming language separate from all of those.
JavaScript is the language of the browser (not exclusively these days, though). It’s primary purpose is to add interactivity to an otherwise static page. In the browser, it’s not going to replace PHP or Ruby for you. It’s not even going to replace your HTML or CSS; you’ll use it in conjunction to them. Also, it isn’t as terrible to learn as you might have thought or heard.
One more note: you’ve heard about jQuery, which is probably the most widely-used JavaScript library. Or maybe you’ve heard about one of the other popular JavaScript frameworks, like Mootools, YUI, Dojo, and others. Where do these fit into the picture? Consider them a collection of JavaScript helper utilities; you’re still writing JavaScript when using them, but it’s heavily abstracted JavaScript. It saves you a ton of work.
You might even have heard someone say that you should start with jQuery (or another library) and learn JavaScript after. I respectfully yet strongly disagree. Get a good handle on JavaScript first, and then use libraries. You’ll understand what you’re doing much better; and, consequently, you’ll be writing much better JavaScript.
Assignment 1: Work Through the Courses at Codecademy.com
Codecademy is a relatively new website that bills itself as “the easiest way to learn how to code.” You’ll be the judge of that! Currently, there are only two course: “Getting Started with Programming” and “JavaScript Quick Start Guide.” This is an awesome way to dip your toes in the JavaScript pool. Very similar to the Try Ruby exercises, you’ll follow short lessons, writing code inside the browser and watching the results. All while earning points and unlocking achievement badges.
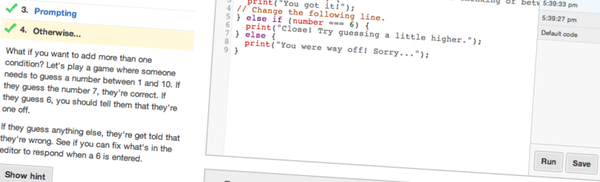
If you’re already familiar with another programming language, you can probably start with the “JavaScript Quick Start Guide”; if this is your first time taking up programming (beyond HTML and CSS), then you’ll find the “Getting Started with Programming” course immensely helpful. Codecademy is free, but signing up is required.
Assignment 2: appendTo's Screencasts
The folks at appendTo have a fantastic set of screencasts geared specifically for beginners. If you want to learn JavaScript the right (and easy) way, definitely work along with these lessons. Visual training is always a plus!
"Level up your skills with our on demand, pragmatic training solution. No signup required. No catch. No kidding."
Assignment 3: Read A Good JavaScript Introduction
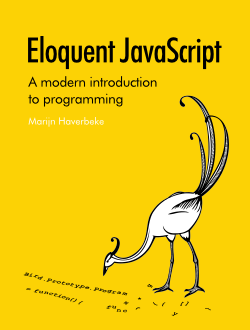
Once you work through the courses at the Codecademy, you’ll want to get a more thorough introduction to JavaScript - an introduction that will introduce you to the all the types, operators, control structures, and more.
A handful of good introductions, if I may:
- A Re-introduction to JavaScript - This introduction is on the Mozilla Developers Network, and certainly does the language justice. It’s a dense work, with almost as many code examples as paragraphs.
- Eloquent JavaScript - This book, by Marijn Haverbeke, is available freely online, but you can also get it on Amazon if you’d like a hard copy. It goes beyond the MDN intro, because it covers not only the JavaScript language, but also coding style and using JavaScript in the browser. Also, “eloquent” isn’t an overstatement.
- Getting Good with JavaScript - Okay, yes, this is my own book, but there’s another reason I’m including it here. It’s really pretty different from the other two intros I’ve listed here; I only cover what you’ll need to know to get up and running as quickly as possible. Also, it comes with over 6 hours of screencasts, so if that you’re thing, check it out. (And yes, this one costs.)
Assignment 4: Install and Learn Firebug (or the Developer Tools)
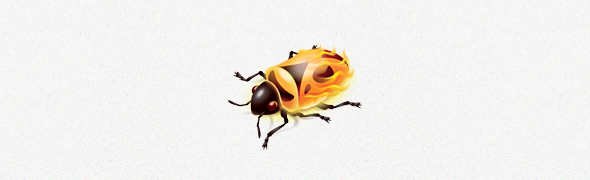
Once you start working with JavaScript in the browser, you’ll want to install Firebug and get familiar with it. Firebug is a plugin for Firefox that assists you in building and debugging your web pages: think of it as the surgeon’s knife for web developers. Don’t use Firefox? Like Safari or Chrome better? No problem: check out the built-in developer tools, which are very similar to Firebug.
You can open the developer tools panel by pressing
Option + Command + I
on the Mac, orControl + Shift + I
on the PC.
You’ll learn a lot by opening up your tool of choice and just clicking around while on one of your favourite websites. Here are a couple of resources that will get you up to speed:
- Firebug
- Developer Tools
Assignment 5: Read a Book
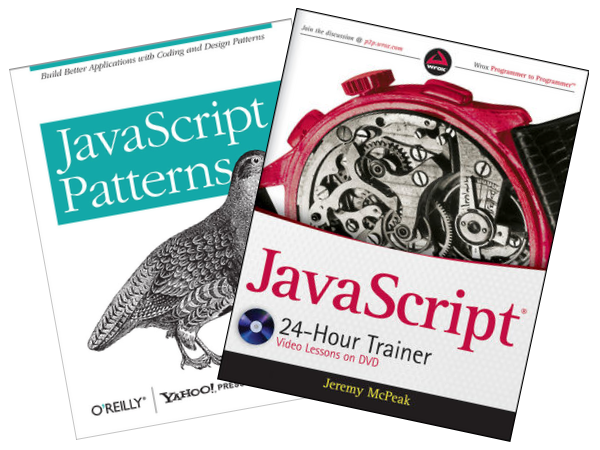
So now you’re familiar with the basics. However, there’s a lot more to learn. While I could recommend a list of books that would bankrupt you, I’ll keep it to four of the highest-quality books you’ll find anywhere:
These first two are general, in-depth JavaScript resources that take what you know from the introductions to a much deeper level; sure, they’ll be some overlap from the introductions, but not much: just enough to keep you comfy.
(Note: while all these books are available on Amazon, I’ve linked to the publishers’ websites, so you can check out the sample chapters available.)
- Professional JavaScript for Web Developers - Written by Nicolas C. Zakas, this book could hardly cover more than it does. If you’ve seen any of Zakas’ work before, you’ll know he’s incredibly thorough. Besides covering the JavaScript language, this book will give you a good handle on using JavaScript in the browser.
- JavaScript 24-hour Trainer - This awesome resource was put together by Jeremy McPeak, who writes for Nettuts+ as well. It’s not just a book: it comes with over 4 hours of video tutorials on DVD. There are 43 lessons, covering everything from syntax to coding guidelines and code optimization.
While those books are great for understanding the JavaScript language and how to use it in the browser, there’s a lot more to learn. And while those books do go into some patterns and practices, here are two books devoted to those subjects that I think you’ll find useful.
- JavaScript Patterns - Written by Stoyan Stefanov. I just finished reading this book, and, boy, do I wish I had read it sooner. After reading the resources above, you’ll know how to write JavaScript, but this book will teach you how to organize it, an important skill that isn’t as common as you’d think.
- JavaScript: The Good Parts - Written by Douglas Crockford. This little gem will explain what’s good and what’s bad about the JavaScript language.
Assignment 6: Build Something!
As you worked through the resources above, you should have been following along with the code samples: pulling them apart, and tweaking them to see what happens. But now it’s time to really strike out on your own. It’s time to actually build something.
So, what can you build? There’s a lot you can do. Here are a couple of ideas.
-
A Photo Gallery: Display a set of photo thumbnails and a main photo. When a user clicks a thumbnail, have the larger version of the thumbnail (not the thumbnail itself) replace the current main photo. Bonus points if you can overlay a caption coming from the thumbnail
alt
tag, or loop through the photos if the user hasn’t clicked one for a minute. - A To-do List: This might sound tougher than it is; but I’m not suggesting you build a full-fledged to-do application. Just have a text box with a button beside it; when you click the button, the entered text becomes an item in an unordered list below. Clicking on a list item removes it. It sounds simple enough, but there are several gotchas that it will be good for you to think about as a beginner.
-
An Animating Box: Animation is always tricky, but it doesn’t have to be complex. Have a
div
with some text in it, and several buttons above. One button can adjust the width; one, the height; and one, the background colour. The key is not to have the changes happen immediately, but over the course of, say, a second. Remember, Google is your friend, especially if you haven’t done any animation in JavaScript thus far.
I’m sure you can think of other projects that will be great practice. Of course, push yourself out of your comfort zone; that’s the only way to learn.
Also, be sure to refer to the JavaScript category here on Nettuts+ for a massive list of tutorials, at all skill levels.
Assignment 7: Begin Learning a JavaScript Library
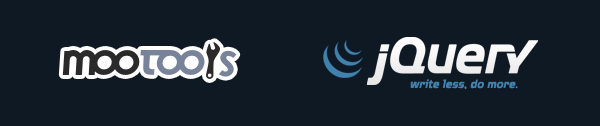
If you’ve come this far, you’ll probably realize that there are several things that are difficult to accomplish in a cross-browser way (or at all) in JavaScript. The biggest offenders are probably things like excessive DOM manipulation, AJAX, and animation. This is where a library comes it.
As I mentioned earlier, the point of a JavaScript library is to sugar down the painful stuff. Therefore, now might be the time to look at one. There are a ton to choose from, and I’ll let you decided which to experiment with. Whether it be jQuery or Mootools, YUI or Dojo, their respective sites will give you everything you need to get started. If you feel the need, give one a try.
Most Popular Libraries
While there's certainly a countless number of libraries available, you should try to stick with a popular choice - at least at first.
Assignment 8: Keep up with the Masters
Drop what you're doing, and subscribe/follow these developers. This is a requirement.
There are a ton of incredible JavaScript geniuses out there, who are always doing cool stuff that you don’t want to miss. Thankfully, our never-sleeping associate editor Siddharth has rounded up a list of ”33 Developers you MUST Subscribe to as a JavaScript Junkie. Drop what you're doing, and subscribe/follow these developers. This is a requirement.
But you can do more. This fair website is often posting about JavaScript, so don’t go away. Also, check out the JavaScript Show, a podcast about the latest and greatest in the world of JavaScript. You might also want to sign up to the JavaScript Weekly email newsletter.
Optional Extra: Check Out the Premium Items
Envato Market has hundreds of popular JavaScript items, from sliders to news tickers, and from calendars to shopping carts.
So take a look at what's out there. Even if you don't buy anything, seeing what other people have created can inspire you with a sense of what's possible with JavaScript.
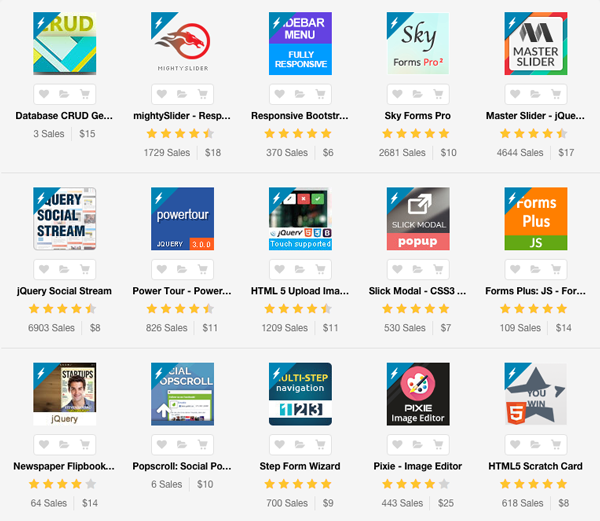
Conclusion
Thanks for reading! Hopefully, this plan can help you become a JavaScript fanatic. If you’re already familiar with JavaScript, can you recommend any other resources in the comments?
Comments