Categories provide the ability to add functionality to an object without subclassing or changing the actual object. A handy tool, they are often used to add methods to existing classes, such as NSString
or your own custom objects.
Step 1: Set Up Your Project
Launch Xcode and click File > New > Project. Choose an iOS Single View Application from the window and click "Next." Name your product "Categories" and enter a name for your Company Identifier, such as "com.companyName.categories." Choose the iPhone device family and click "Next." Choose a location to store your project and click "Create."
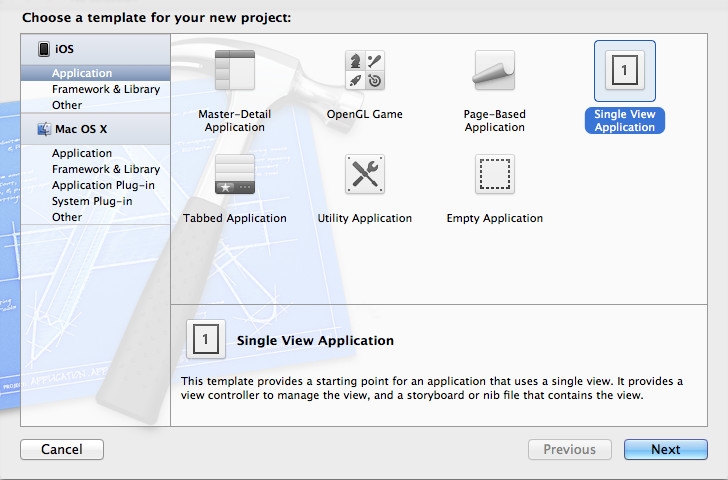
Step 2: Create the Category
Now that your project is set up, let's create a category that adds additional functionality to the NSString
class. Click File > New > File and choose a Cocoa Touch Objective-C category from the window. Click "Next." Name your category "RemoveNums" and select NSString
from the "Category on" drop down menu (you may need to type this in manually). Click "Next" followed by "Create."
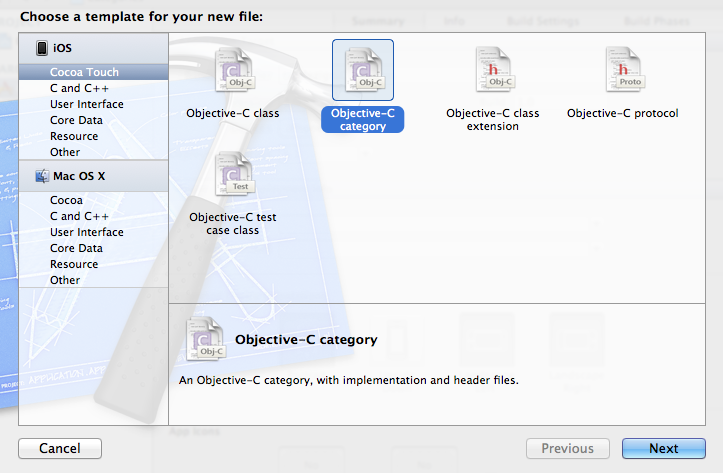
Declare the Category Method
Back in your Xcode project, click "NSString+RemoveNums.h" to view the new category's header file. Add the following code to the interface to declare the method.
@interface NSString (RemoveNums) - (NSString *)removeNumbersFromString:(NSString *)string; @end
Implement the Category Method
Click "NSString+RemoveNums.m" to view the category's implementation file. Add the following code to create a method that will remove all the numbers from an NSString
. First we define an NSCharacterSet
of the numbers zero through nine which we'll use as a reference to compare against the original input string. In this case, the original string "ABC 123" will have the numbers "123" removed from the string because the category method uses the NSString
method stringByTrimmingCharactersInSet:
.
- (NSString *)removeNumbersFromString:(NSString *)string { NSString *trimmedString = nil; NSCharacterSet *numbersSet = [NSCharacterSet characterSetWithCharactersInString:@"0123456789"]; trimmedString = [string stringByTrimmingCharactersInSet:numbersSet]; return trimmedString; }
Step 3: Import the Category
Click "ViewController.h" and import the category by adding the following code.
#import "NSString+RemoveNums.h"
Step 4: Test the Category
Click "ViewController.m" and add the following code to the viewDidLoad
method. The local variable stringWithNums
contains a combination of letters and numbers. The next line takes the string variable and runs it through the category method removeNumbersFromString
. Finally, NSLog
outputs the returned value of the newly trimmed string without any numbers.
NSString *stringWithNums = @"ABC 123"; NSLog(@"stringWithNums --> %@",stringWithNums); stringWithNums = [stringWithNums removeNumbersFromString:stringWithNums]; NSLog(@"trimmed stringWithNums --> %@",stringWithNums);
Step 5: Use the Category Method
Click Product > Run, or click the "Run" arrow in the top left corner, to test the code. Notice the console shows the original input string, "ABC 123," as well as the string after it has been altered by the category method and the numbers have been removed.

Conclusion
Subclassing is one way to add functionality to an object, but avoiding unnecessary subclassing by using a category will help reduce the amount of code and keep your projects more organized. There are a number of scenarios where using a category is beneficial. Share your category scenarios in the comments below.
Comments