One of the hardest parts of writing a series for beginners on object-oriented programming is knowing where to stop.
There are so many topics to cover that we can slowly begin moving into the direction of advanced programming techniques ultimately forgoing exactly what our intended mission was: to arm beginners with a set of tools, strategies, and understanding of beginning concepts.
First, note that in in the last post in the series, we completed our first, full plugin using object-oriented techniques. For the most part, it encapsulated everything that we've covered up through this point in the series (except, obviously, inheritance).
To that end, be sure to catch up on the following articles:
- An Introduction
- Classes
- Types
- Control Structures: Conditional Statements
- Control Structures: Loops
- Functions and Attributes
- Scope
- Building the Plugin I
- Building the Plugin II
- Document the Plugin I
- Document the Plugin II
It's a lot, I know, but remember: The goal of the series is to prepare the absolute beginner with everything that's necessary to being working with PHP and writing WordPress plugins using object-oriented techniques.
To that end, I've decided to begin wrapping up this series with the a two-part article (with a third, final part serving as a summary) that offers a segue into the next topic of development for aspiring PHP programmers: Inheritance.
Again, for those who are more experienced programmers, then inheritance is not a target subject matter for you; however, if you're a beginner, then inheritance is one of the concepts that's easy to grasp, a little more challenging to implement (believe it or not), and that can cause even more confusion when it comes to topics such as polymorphism (which we'll talk about later)
Over the next two articles, I aim to cover all of the above along with sample code to back it up. But before we look at ay of code, I think it's important to understand the concepts of importance, to look at a few of the steps necessary to prepare object-oriented code for inheritance.
In this article, we're going to be defining inheritance, trying to form a conceptual model of what's actually going on, examining the nuances of what are known as base classes and subclasses, as well as some of the reserved keywords in the language that must be adjusted in order to support inheritance through out classes.
So with that set aside as our roadmap for the article, let's go ahead and get started.
Inheritance Defined
Unlike a number of other programming terms, inheritance is actually a word that describes it's concept pretty well. Straight from Wikipedia:
In object-oriented programming (OOP), inheritance is when an object or class is based on another object or class, using the same implementation. It is a mechanism for code reuse. The relationships of objects or classes through inheritance give rise to a hierarchy.
Relatively clear, isn't it? But I think we can do better.
Earlier in this series, we talked about how many of the more common programming languages use examples such as Animals
and Vehicles
as a way to demonstrate the concept of object-oriented programming.
After all, the idea behind object-oriented programming is that we should be modeling real-world objects? Well, sort of. But how many times have you seen a physical Blog_Post
?
Exactly.
As such, I always like to try to put things in the perspective of something that's far more tangible, more practical, and that's more closely related to the content that we'll actually be creating.
With that said, what's an adequate way we can describe inheritance within the context of object-oriented programming that doesn't wreck the concept through the use of trivial examples?
Let's try this:
Inheritance is when one class serves as the parent class for a child class that provides a number of attributes and methods common to both the parent and child; however, the child as the ability to introduce it's own attributes.
In the quote above, we're using a couple of terms like "parent class" and "child class" each of which we'll clarify in a little bit, but the point is that we can literally create a hierarchy of classes all of which inherit information from their parent classes.
Perhaps even neater, whenever you're working with a child class and you want to take advantage of attributes and functions defined in the parent class or the base class, you can easily do so with no extra code.
But we're getting ahead of ourselves. Before we do that, let's make sure that we can get a conceptual model of what inheritance looks like. After all, although we're writing code, we're trying to provide a model that not only represents an actual object, but creates a relationship between the objects, too.
Visualizing Inheritance
Before we go any further, let's take a look at a very simple class diagram as to how inheritance works.
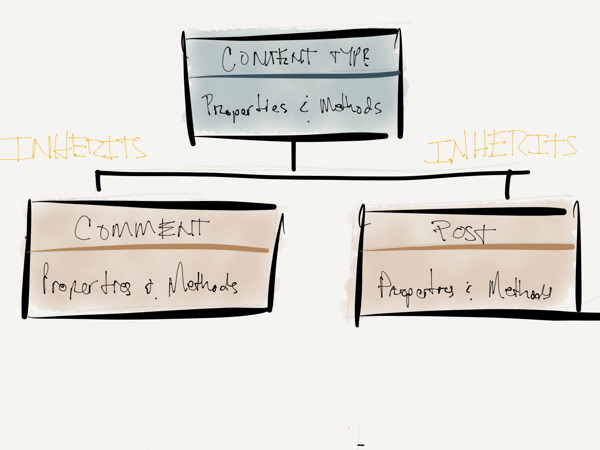
-
Content
which will serve as the base class and that represents a generic type of information that holds data for other, more specific types of content. -
Comment
which represents a comment on a blog post. This class contains information that it inherits fromContent
and that it defines within itself. -
Post
also inherits fromContent
which represents a single blog post. It contains generalContent
information, but also contains its own information specific to that class.
Now, this is clearly a simplified example of inheritance, but at its core this is how inheritance works. In a future article, we'll take a look at how instantiation, data access, and other features work, as well.
But first, we still need to clarify a few terms and make sure that we have all of the proper information.
Class Jargon
As we've touched on earlier in this series, there are a number of terms that we've used all of which are key to understanding how the various pieces of inheritance work together.
To that end, it's important to define some common definitions for the words that we're using not only because of how we're using them throughout this article because you're not only going to see them used here, but you're going to see them used elsewhere and you're going to see them used interchangeably in other articles throughout the web:
- A parent class, also referred to as a base class, is the class from which other classes inherit information as demonstrated in the illustration above. It maintains a set of properties and functions.
- Note that some of the functions that are available in the base class to third-party classes, the child classes, or only within the base class itself.
- The child class, typically called the subclass, is the class that inherits information, data, and functionality from its parent class.
- We'll look at code about this in the next post; however, note that access to data only goes one way. That is, subclasses can access information in their base class, but base classes are unaware of their subclasses.
This should clear up a lot of of the terminology around this; however, if not, think of it in terms of, say, a family tree where you have parents and children. Children inherit traits from their parents, but parents do not inherit traits from their children.
Note also that, in programming, some developers want to introduce what's called "multiple inheritance" which basically means that a single class can inherit properties and methods from multiple classes.
Not only is this restricted in PHP, but it's also outside the scope of this particular series.
An Aside for Abstract Classes
For those who are a little more familiar with the concept of inheritance, then you're likely also familiar with the concept of abstract classes.
Then again, if you're familiar with the concept of abstract classes, I'd argue that you're not a beginner and you aren't really the target audience for the content that we're reaching with this article.
So depending on the feedback on this article, this series, and if others are interested, perhaps we can do a follow-up article or a couple of articles that covers this exact topic.
Coming Up Next...
In the next article, we're going to continue our discussion on inheritance as we take a look at how to implement it within PHP. We're also going to be taking a look at how subclasses can access data from their parents, and how parent classes can secure information within themselves
In the meantime, be sure to leave any feedback, questions, and/or comments about inheritance in the comment feed and I'll look to address them here or in the next post.
Until then!
Comments