As outlined in the first post in this series, we're approaching the concept of object-oriented programming within the context of WordPress, and we're doing so for the very beginner.
That means that if you've never heard of OOP, of if you've been curious to learn it, and you're someone who is interested in WordPress and learning how to develop solutions on top of it, then this series is for you.
Over the next set of articles, we're going to be covering all of the major aspects of object-oriented programming. Once we do that, we're going to look at how we can apply what we've learned by building a working solution for WordPress.
But first, we've got to start with the basics.
What Are Classes?
If you were to ask 10 different developers for their definition of a class, you'd likely get many similar answers, but few of which were actually the same. In fact, the one I heard repeated the most often when I was younger went like this:
A class is a blueprint for creating an object.
In theory, it sounds great especially if you know what an object is. But that's the problem, isn't it? We're trying to learn about object-oriented programming, so there's no guarantee that we even know what an object is; therefore, how can we understand that a class serves as a blueprint for it?
In a sense, it's begging the question.
So let's back up a few steps and define what an object is in order to more clearly define what a class is.
Understanding Objects
The whole idea of the object-oriented programming paradigm is that we, as programmers, can more easily model the information that we see in the real world using constructs in a code.
For example, in the real world we have objects that can be described using adjectives, and these objects can perform actions. Though this may be a bit cliche, think for a moment, about a car:
- It has several adjectives such as size and color.
- It can drive and it can park.
Again, a simple example, but it proves the point that everything that we have in the real world can usually be reduced to a noun that can be described by its adjectives and the actions that it performs.
So let's generalize this idea to objects. In fact, let's substitute one word for another:
- A noun is an object.
- An adjective an an attribute (or a property).
- A verb is a method (or a function).
Easy enough, right? The short of it is that we should be able to describe the things that we see in the real world as objects within a programming paradigm. Note that some languages call attributes properties, and some call methods functions. It doesn't really matter, either - it's all the same. They simply refer to adjectives about the object and actions that it can take, respectively.
Poor Examples
Next, most programming courses or books always start off with an example about how objects are meant to model real world objects (similar to like I did with the car example above).
And to some degree, there is truth to that. For those who have been working in development, then you're likely familiar with how we can model people within the context of our application, but that's getting ahead of ourselves.
Though it's true that we can use object-oriented programming to model real world objects, I've found that, more often that not, I'm modeling a more generalized form of a real world object - such as a user rather than a person - and that the actions they perform are more unique to them.
To that end, the examples that I want to give throughout this article and those in the rest this series are going to be more geared towards practical applications in computer programming. No one is going to be writing a car plugin and no one is going to be creating an animal object (which is something that you also often see and hear in introductory programming courses).
Instead, we're going to try to focus a bit more on objects that are more likely to be seen in the realm of programming - not in the real world. Not because object-oriented programming is weak, but because the way we go about teaching is weak.
Good Examples
Of course, this raises the question of what constitutes a good example? The trouble with answering a question like this is that it can literally be a wide, wide variety of things.
This includes objects such as:
- a blog post,
- a document such as a resumé,
- an authenticator or authentication system,
- a product,
- a password generator,
- ...and so on.
And you see: Many of these things don't truly exist in the real world. For example, blog posts aren't tangible. They are things that we read on our screens. But does that mean they don't have properties such as a date, a time, and an author? Or does it mean that they don't have actions such as publish, and delete?
Of course not.
So as we progress throughout this series, we're going to be talking about object in terms of the things with which we're more likely to work.
I don't see us programming an animal - and certainly not so in WordPress - at any time during this series :).
Back to the Basics
Alright, now that we've taken a brief digression into what objects actually are, and good and bad examples of each, it's time to actually start talking about classes and how they truly serve as blueprints for objects.
In programming, an object is created from a class. That means that a class defines all of the properties an object has and the actions that it can take, and then the computer will create an object in memory.
When it comes to classes, you may hear developers discuss writing classes, defining classes, or building classes. Any and all of these terms are acceptable.
After that, you may hear developers talking about creating objects. The act of creating an object is called instantiation. Yeah - it's a big word for a relatively simple concept. But think of it this way: When you have a class, you have a definition out of which you can create multiple instances of an object.
If we need to draw an analogy to the real world, think first about a set of blueprints for a house. It lays out the floor plan, dimensions, walls, and so on that give construction workers information on how to build a house. Then, when it comes time to actually construct a house, then a team of construction workers erect the house based on the blueprint.
Such is the case with classes, instantiation, and objects. Classes are the blueprints, the computer is the team of construction workers, and the objects are the house. And just as multiple houses can be erected from a single blueprint, such is the case with objects and classes.
The Mental Models of Classes and Objects
When it comes to writing code, some people are able to picture the object in their heads - others, maybe not.
Personally, I think that this has more to do with how each of us tend to learn and process information, but I do believe that it's possible to begin to mentally picture how software systems work together the longer you write code.
Here, we're not going to look at any complex system; however, are going to take a look at an example of a class and then a visual representation of what that might look like in terms of code and in terms of a mental image.
A Class for a Blog Post
Since we're dealing with WordPress, perhaps an initial example for a class would be one that represents a blog post.
Granted, this will be a simple example to demonstrate the ideas of attributes and functions, but we'll cover various constructs in more details in future articles.
So, with that said, let's say that we're going to create a class for a blog post. Let's also say that our blog post will have an author, a date on which it was published, whether or not it is published, and the actions to publish and delete.
An example class definition for a blog post with these attributes and functions will look like this:
class Blog_Post { private $author; private $publish_date; private $is_published; public function publish() { // Publish the article here } public function delete() { // Delete the article here } }
For those who are familiar with object-oriented programming, you may recognize some of the information above, and you may also recognize that I've left out certain things (such as the constructor). Don't worry about that - we'll cover those concepts later.
For those who are completely new to programming, this is what a basic class definition looks like. For the time being, don't worry about the words private
and public
as we'll cover those later.
Instead, focus on $author
, $publish_date
, and $is_published
. These are the attributes. Notice that they sit above the function definitions in the class. These are analogous to adjectives that describe the Blog_Post
.
Next, we have the functions publish()
and delete()
. These two functions are the actions that can be taken on the Blog_Post
. Remember, just how objects in the real world can move (like a car can drive), a blog post can be published.
A Visual Representation
So what happens when you actually instantiate a blog post? That is to say, when we create a blog post in code, how can we mentally picture what's happening within the computer.
First, let's outline how a blog post is instantiated. Remember, instantiated is a word just like erected is for construction workers - it's how an object is created from a class.
Next, let's see how we can use the single class definition to create three different blog posts:
$first_post = new Blog_Post(); $second_post = new Blog_Post();
Easy enough to read, right? Above, we've created two variables that will reference two completely different Blog_Post
objects.
Note that although we'll talk about this more in a future article, the word new
is what instructs the computer to instantiate a Blog_Post
from us from the class definition.
So let's take a look at the first example where we get our first instance of Blog_Post
.
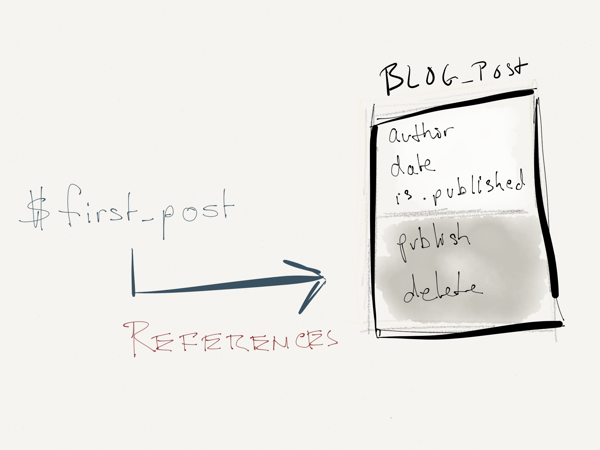
Notice in this illustration, we have the $first_post
variable that refers to the instance of the Blog_Post
that was created. The Blog_Post
exists in the computer's memory, it has its attributes that are available as well as its methods that are available.
We can access all of these through the $first_post
variable that references this object. We'll talk about that in more detail later in the series.
But what about the $second_post
? How does that look within the context of object-oriented programming?
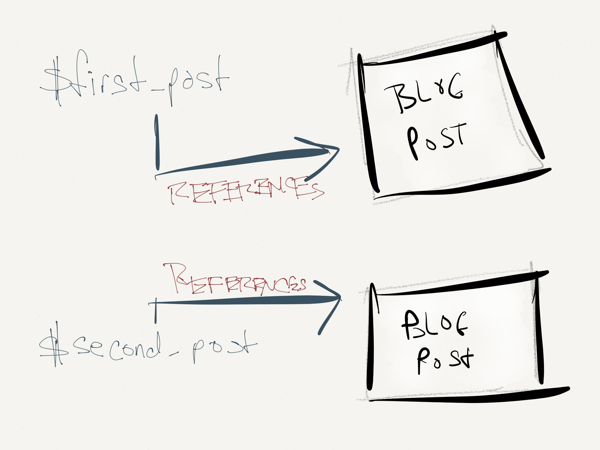
If you compare the two illustrations, they look pretty much the same, right? $first_post
references one instance of Blog_Post
, $second_post
references a second instance of Blog_Post
even though they both came from a single class.
Note that for those who are technically astute, it is possible for both variables to reference a single instance, but that's outside the scope of this article.
What's Up Next?
Anyway, at this point, you should have a high-level understanding of what a class is, the role it plays in object-oriented programming, and how we can create instances of objects that are accessible through variables.
This still doesn't show us how to interact with the classes though, does it? We'll get to that, but first we need to discuss some of the more primitive aspects of programming such as strings, arrays, loops, and conditionals.
Each of the aforementioned constructs will help us give life to our objects, and once we've taken a tour of each of those, we'll come back to make more mature classes that can actually do work.
Comments