Even if you've only dipped your toes into the world of iOS development, you almost certainly know about UIAlertView
. The UIAlertView
class has a simple interface and is used to present modal alerts.
Apple has deprecated UIAlertView
in iOS 8 though. As of iOS 8, it is recommended to use the UIAlertController
class to present action sheets and modal alerts. In this quick tip, I will show you how easy it is to transition from UIAlertView
to UIAlertController
.
1. Project Setup
Launch Xcode 6.3+ and create a new project based on the Single View Application template.
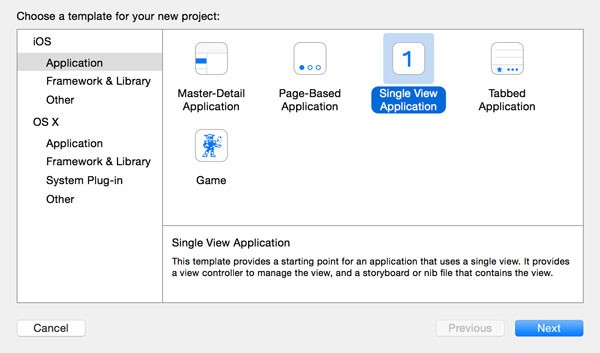
Name the project Alerts, set Language to Swift, and set Devices to iPhone. Tell Xcode where you'd like to store the project files and click Create.
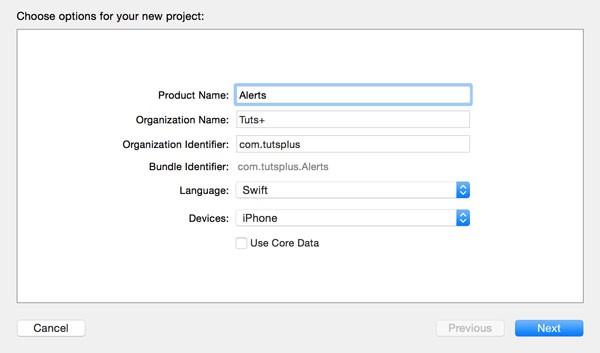
Let's start by adding a button to trigger an alert view. Open Main.storyboard and add a button to the view controller's view. Set the button's title to Show Alert and add the necessary constraints to the button to keep it in place.
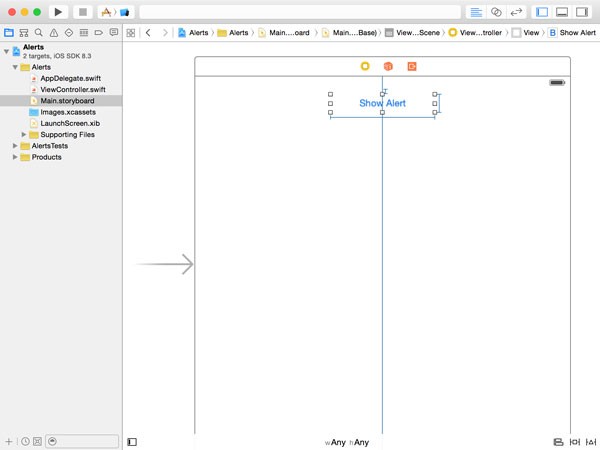
Open ViewController.swift and add an action to the class implementation. Leave the action's implementation empty for the time being. Revisit Main.storyboard and connect the view controller's showAlert
action with the button's Touch Up Inside event.
@IBAction func showAlert(sender: AnyObject) { }
2. UIAlertView
Let's start by showing an alert view using the UIAlertView
class. As I mentioned, the interface of the UIAlertView
class is very simple. The operating system takes care of the nitty gritty details. This is what the updated implementation of the showAlert
action looks like.
@IBAction func showAlert(sender: AnyObject) { // Initialize Alert View let alertView = UIAlertView(title: "Alert", message: "Are you okay?", delegate: self, cancelButtonTitle: "Yes", otherButtonTitles: "No") // Configure Alert View alertView.tag = 1 // Show Alert View alertView.show() }
The initialization is straightforward. We provide a title and a message, pass in a delegate object, a title for the cancel button, and titles for any other buttons we'd like to include.
The delegate object needs to conform to the UIAlertViewDelegate
protocol. Because the view controller will act as the alert view's delegate, the ViewController
class needs to conform to the UIAlertViewDelegate
protocol.
import UIKit class ViewController: UIViewController, UIAlertViewDelegate { ... }
The methods of the UIAlertViewDelegate
protocol are defined as optional. The method you'll use most often is alertView(_:clickedButtonAtIndex:)
. This method is invoked when the user taps one of the alert view's buttons. This is what the implementation of the alertView(_:clickedButtonAtIndex:)
method could look like.
func alertView(alertView: UIAlertView, clickedButtonAtIndex buttonIndex: Int) { if alertView.tag == 1 { if buttonIndex == 0 { println("The user is okay.") } else { println("The user is not okay.") } } }
Build and run the application in the iOS Simulator to see if everything is working as expected.
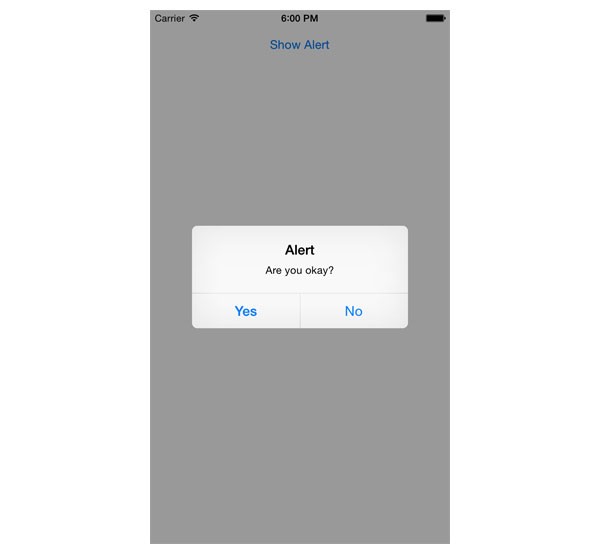
3. UIAlertController
The interface of UIAlertController
is very different from that of UIAlertView
, but Apple's motivation to transition to the UIAlertController
class makes sense once you've used it a few times. It's an elegant interface that will feel familiar.
The first benefit of using the UIAlertController
class is the absence of a delegate protocol to handle user interaction. This means that we only need to update the implementation of the showAlert
action. Take a look at the updated implementation below.
@IBAction func showAlert(sender: AnyObject) { // Initialize Alert Controller let alertController = UIAlertController(title: "Alert", message: "Are you okay?", preferredStyle: .Alert) // Initialize Actions let yesAction = UIAlertAction(title: "Yes", style: .Default) { (action) -> Void in println("The user is okay.") } let noAction = UIAlertAction(title: "No", style: .Default) { (action) -> Void in println("The user is not okay.") } // Add Actions alertController.addAction(yesAction) alertController.addAction(noAction) // Present Alert Controller self.presentViewController(alertController, animated: true, completion: nil) }
The initialization is pretty easy. We pass in a title, a message, and, most importantly, set the preferred style to UIAlertControllerStyle.Alert
or .Alert
for short. The preferred style tells the operating system if the alert controller needs to be presented as an action sheet, .ActionSheet
, or a modal alert, .Alert
.
Instead of providing titles for the buttons and handling user interaction through the UIAlertViewDelegate
protocol, we add actions to the alert controller. Every action is an instance of the UIAlertAction
class. Creating an UIAlertAction
is simple. The initializer accepts a title, a style, and a handler. The style argument is of type UIAlertActionStyle
. The handler is a closure, accepting the UIAlertAction
object as its only argument.
The use of handlers instead of a delegate protocol makes the implementation of a modal alert more elegant and easier to understand. There's no longer a need for tagging alert views if you're working with multiple modal alerts.
Before we present the alert controller to the user, we add the two actions by calling addAction(_:)
on the alertController
object. Note that the order of the buttons of the modal alert is determined by the order in which the actions are added to the alert controller.
Because the UIAlertController
class is a UIViewController
subclass, presenting the alert controller to the user is as simple as calling presentViewController(_:animated:completion:)
, passing in the alert controller as the first argument.
4. UIActionSheet
Unsurprisingly, Apple also deprecated the UIActionSheet
class and the UIActionSheetDelegate
protocol. As of iOS 8, it is recommended to use the UIAlertController
class to present an action sheet.
Presenting an action sheet is identical to presenting a modal alert. The only difference is the alert controller's preferredStyle
property, which needs to be set to UIAlertControllerStyle.ActionSheet
, or .ActionSheet
for short, for action sheets.
Conclusion
Even though UIAlertView
and UIActionSheet
are deprecated in iOS 8, you can continue using them for the foreseeable future. The interface of the UIAlertController
class, however, is a definite improvement. It adds simplicity and unifies the API for presenting modal alerts and action sheets. And because UIAlertController
is a UIViewController
subclass, the API will already feel familiar.
Comments