Before we can start creating iOS applications, we need to set up the development environment. In this tutorial, I show you how to register as an Apple developer and install the development tools you need to get started.
1. Requirements & Prerequisites
The primary focus of this tutorial is getting started with Xcode. Xcode is an outstanding Integrated Development Environment (IDE) developed by Apple. The vast majority of OS X, iOS, watchOS, and tvOS developers rely on Xcode for building applications.
Xcode is only compatible with Apple's OS X operating system and I am therefore assuming that you have a Mac capable of running Xcode. I will be using Xcode 7.1 throughout this series.
This particular version of Xcode requires OS X 10.10.5 or higher. Even though it is possible to create iOS applications with an older version of Xcode, I recommend that you also use Xcode 7.1 to make sure that you don't run into unexpected issues along the way.
2. Join the Apple Developer Program
Apple recently changed its Apple Developer Program. Members of the Apple Developer Program can now create and publish applications for OS X, iOS, watchOS, and tvOS. The Apple Developer Program is still paid, though.
If you're just testing the waters and don't want to enroll just yet, then you can still follow along as long as you have an Apple ID. As of 2015, it is possible to develop applications for Apple's platforms with nothing more than an Apple ID. As long as you're thirteen years or older, anyone can create an Apple ID and get started with Xcode.
If you plan to submit your applications to the App Store, then you're required to enroll for the paid Apple Developer Program. The paid Apple Developer Program has three enrollment types, individual, organization, and enterprise. Apple also has an iOS Developer University Program for educational institutions.
At the time of writing, the individual and organization enrollment types cost $99 per year. The enterprise program is more expensive at $299 per year. The enterprise program is aimed at companies and organizations that intend to deploy in-house applications, applications that are not distributed through Apple's App Store.
Step 1: Create an Apple ID
Before you can start developing iOS applications, you need an Apple ID. You also need one to enroll in the Apple Developer Program. Chances are that you already have an Apple ID. You can create one for free on Apple's website. It only takes a few minutes to create one.
Step 2: Enroll in Apple's Developer Program
You can complete this series without enrolling in the Apple Developer Program, but keep in mind that you won't be able to submit applications to the App Store. If you enroll in the Apple Developer Program, you can develop for OS X, iOS, watchOS, and tvOS. Visit Apple's developer website for more information about the Apple Developer Program.
If you decided to enroll in Apple's Developer Program, then head over to the Apple Developer Program website and click Enroll in the top right. Sign in with your Apple ID and follow the steps. The process can take a few days to complete since Apple manually approves each application. For more information, visit Apple's developer support center.
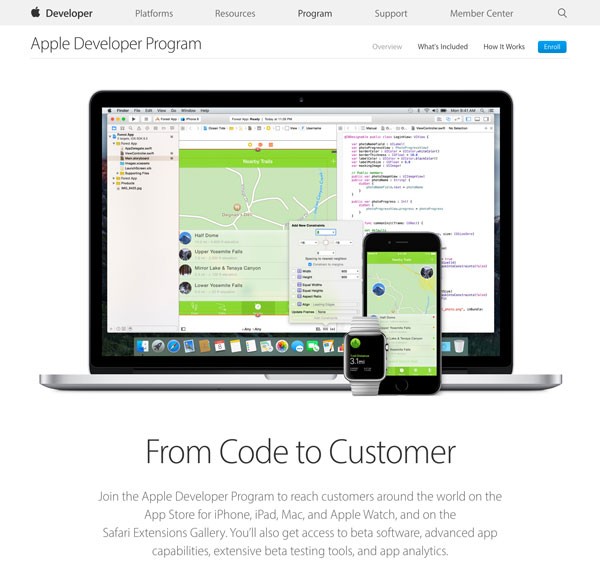
3. Install Xcode
The name Xcode can be somewhat confusing at first. The name is often used to refer to the entire toolset for OS X, iOS, watchOS, and tvOS development, and that toolset includes the Xcode IDE, the simulator for running applications, and the actual OS X, iOS, watchOS, and tvOS SDKs (Software Development Kit). However, it's important to understand that the Xcode application itself is just an IDE and when I use the name Xcode I'm typically referring to just that.
You can download Xcode in one of two ways, through Apple's developer website or through the App Store on OS X. The advantage of the App Store on OS X is that updating Xcode is easier and faster.
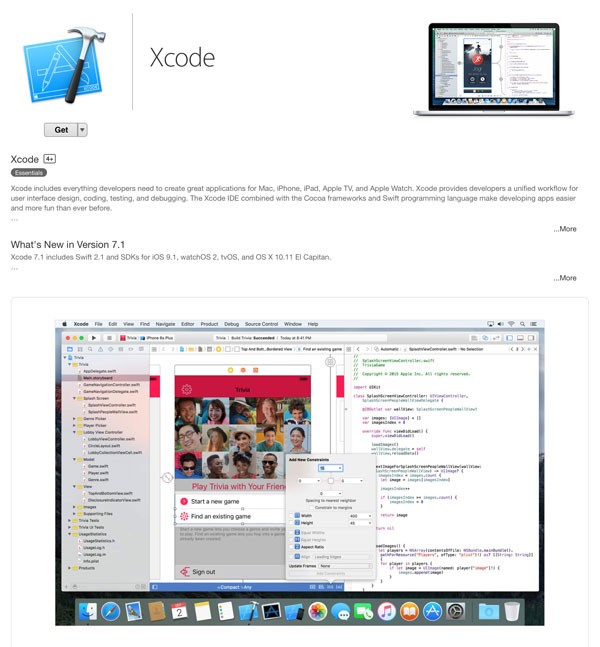
The advantage of downloading Xcode through Apple's developer website is that you can download developer previews. If you like living on the edge, then you'll like working with the developer previews. Since you are new to iOS development, I suggest you download and install Xcode through the App Store so you can work with the most recent stable release.
Open the App Store, search for Xcode, and start the installation process. Xcode is several gigabytes in size so you may want to grab a cup of coffee or, even better, go for a walk.
4. Create Your First Application
You've barely touched your computer and we are already set up and ready to create iOS applications. If you're familiar with the process of setting up the Android SDK, then I'm sure you can appreciate this simplicity.
With Xcode installed, it is time to launch it for the first time. If all went well, you should see the Welcome to Xcode window, which contains a few useful links and helps you create a new application. To create your first iOS application, select Create a new Xcode project from the list of options.
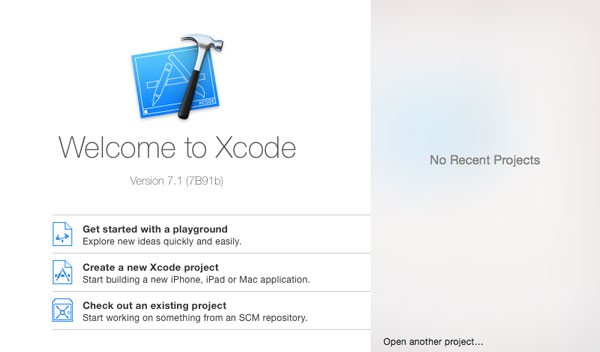
What is an Xcode project? An Xcode project is a folder or package that contains all the necessary files and components to manage and build your application. You'll become more familiar with Xcode projects during this series.
Xcode makes it easy to create a new Xcode project by offering a handful of useful project templates. The Single View Application template is a good choice for your first application. Select it from the list of iOS > Application templates and click Next.
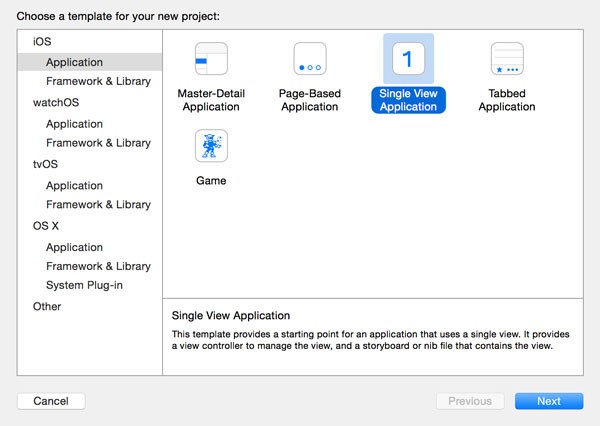
The next window lets you configure your Xcode project. Fill in the fields as shown in the screenshot below and click Next. In an upcoming article in this series, I will explain each of the configuration options in more detail. The focus of this article is getting your first application up and running in the simulator.
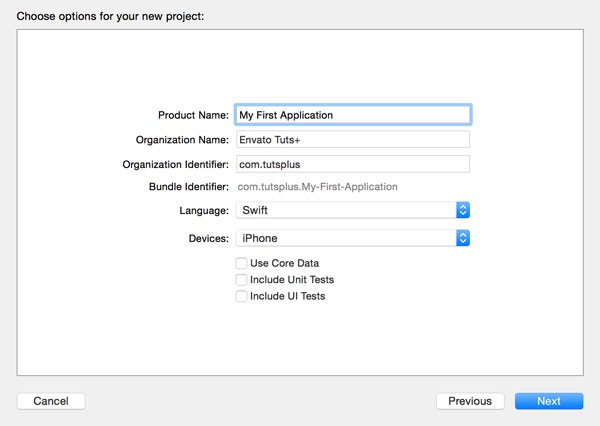
In the final step, Xcode asks you to specify a location for storing your new Xcode project. It doesn't really matter where you save the project as long as you can find it later. You'll also notice that Xcode offers the option to create a local Git repository for your project. I highly recommend that you use source control for any type of development. Git is an excellent choice and it is the most popular SCM (Source Control Management) system in the OS X and iOS community.
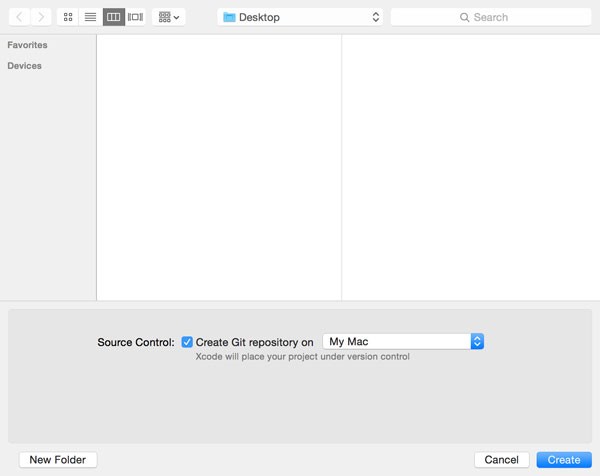
It's important to get familiar with source control management if this is new to you. Source control is indispensable in software development for various reasons. To begin using Git, you can visit Git's website or read its Wikipedia entry. There's also an excellent book by Scott Chacon that discusses Git in more detail.
Envato Tuts+ has two excellent courses on Git. In the first course, Git Essentials, Andrew Burgess covers the basics of Git. In the second course, Introduction to Git and GitHub, Dan Wellman goes into detail about Git and GitHub.
In the rest of this series on iOS development, I won't bother you with source control. Even though source control is important, I don't want to overcomplicate this series by adding an extra layer of complexity.
5. Build & Run
You have successfully set up your first Xcode project. Believe it or not, running your application in the simulator is only one click away. There's a large play button on the far left of the Xcode toolbar.
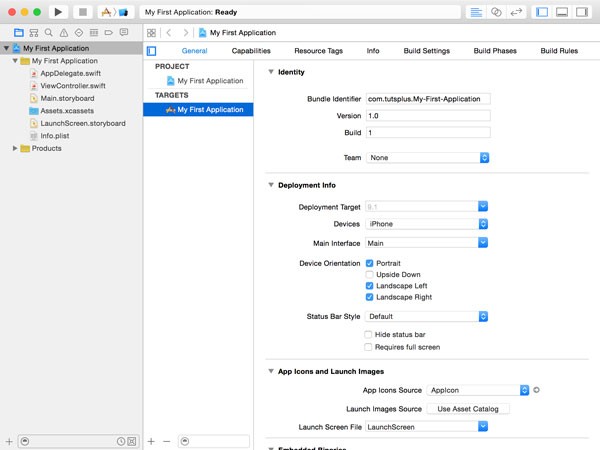
Before you run your application, make sure that the active scheme is set to iPhone 6 or one of the other iOS Simulators. We will cover schemes in more detail later on in the series. Take a look at the next screenshot to make sure you're on the same page.
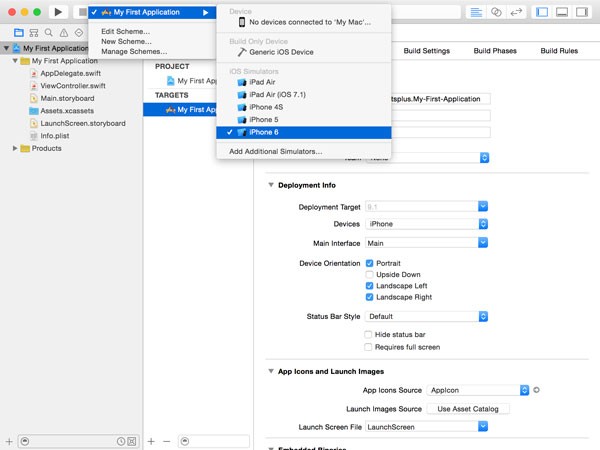
Are you ready to build and run your very first iOS application? Click the play button in the top right to build your project and run your application in the simulator. Alternatively, you can press Command + R or select Run from Xcode's Product menu. If all went well, you should see the simulator running your application. Of course, your application doesn't do anything useful yet. We'll fix that later in this series.
6. Simulator
The simulator is a valuable tool in your arsenal. Building and running an iOS application in the simulator is faster than deploying an application to a physical device. There is one caveat, however. For various reasons, the simulator doesn't perfectly mimic an iOS device.
For example, the simulator doesn't have a camera or an accelerometer, its GPS capabilities are limited to a list of predefined locations and routes, and user interaction is limited to gestures that require one or two fingers. Also, keep in mind that the simulator draws power and resources from the machine it runs on. This means that the simulator isn't suitable for testing application performance.
The point is that you should always test an application on a physical device before submitting it to the App Store or distributing it to testers. Even if you only have one device to test with, it is critical to test your applications on a physical device instead of relying solely on the simulator.
In spite of its shortcomings, the simulator is a very capable tool. Select the simulator and take a look at its menu and the various options it provides. The simulator is perfectly suited for testing user interfaces on different form factors, mimicking simple gestures, simulating memory warnings, or taking screenshots for the App Store. You'll find it a very useful tool for iOS development.
7. Physical Devices
As of 2015, running an application on a physical device is possible for every Apple developer, even if you don't enroll in the paid Apple Developer Program. That said, running an application on a physical device involves a few extra steps. These steps include configuring your project and setting up the device you plan to test with. Because this is a fairly complex topic, especially if you are new to iOS development, I will thoroughly explain how to do this in a separate article in this series.
8. Third Party Development Tools
I would like to end this article by listing a handful of third party tools that make iOS development easier and more enjoyable. Note that these applications are by no means required for iOS development, but knowing that they exist may save you a lot of frustration in the future.
- Dash: Dash is a superb documentation browser and code snippet manager that I use constantly during development. Dash is a great alternative for Xcode's built-in documentation browser. It supports dozens of languages and frameworks, which means that you can use it for almost any type of development.
- Tower: Tower is one of the best OS X applications for working with Git. Git is a command line tool, but some people prefer to use a graphical user interface instead. If you are not a command line superhero, then you'll definitely appreciate what Tower has to offer. Another great alternative is SourceTree, developed by Atlassian.
- TextExpander: TextExpander is a popular utility for managing text snippets linked to custom keyboard shortcuts. Many developers use it for development, but you can use it wherever you like. It also supports placeholders for even more customizability.
- Fabric: Fabric, owned by Twitter, is a suite of tools for mobile development. It allows developers to distribute test builds, collect crash reports, and integrate analytics. There are a number of alternatives that you may want to look into, such as HockeyApp and Apple's own TestFlight platform.
Conclusion
I hope you agree that setting up the development environment for iOS development is easy. With Xcode installed, we can start exploring the iOS SDK. That will be the focus of the rest of this series.
If you have any questions or comments, you can leave them in the comments below or reach out to me on Twitter.
Comments