This tutorial will teach you all about the latest multitasking enhancements provided by the iOS 7 SDK. Specifically, you'll learn about the Background Fetch, Remote Notifications, and Background Transfer Service APIs. Read on!
Multitasking Overview
With iOS 7, Apple has made three new multitasking APIs available to developers:
- Background Fetch
- Remote Notifications
- Background Transfer Service
All these APIs give programmers the ability to develop multitasking applications which will make better use of the device hardware while providing users with a greater experience. This tutorial will teach you more about the above three enhancements and when to use them.
Background Fetch
In order to make the Background Fetch API more easily understood, imagine that you want to build a news app. Hypothetically, we make the assumption that this app's content is provided from the web, and one of the essential features is to get updates each time the user launches the app.
Prior to iOS 7, developers could make the app begin getting new content once it was launched. This works, but the downside is that users will need to wait a while every time the app starts. Also, the phone's connection speed becomes a significant factor. Imagine if the user is connecting via a cellular network after a week or two of inactivity! Of course, this is not what developers want, as it tends to degrade the user experience.
On iOS 7, things have drastically changed! With the Background Fetch API, our hypothetical application can actually download new content passively while still remaining in the background. iOS 7 will wake the app up, provide background execution time, and, when the app finishes updating, it goes back to sleep once again. Of course, users aren't notified when this happens. They simply find the new content ready to go upon application launch.
The update process can take place at either predefined intervals set by the programmer or in intervals specified by the operating system. If you set custom intervals, Apple recommends to set them wisely and not to fetch data on the background very often. Doing so will lead to battery drain and wasted system resources. If you simply let the operating system handle things, iOS 7 will make usage predictions. It does this by observing how often the app is launched and at what times. It then anticipates this behavior by going online and fetch content before it predicts the user will want it. For example, if a user of our hypothetic news app launches the app every afternoon, iOS observes this and decides that the app should fetch content sometime in the afternoon and before the usual launch time. Very cool!
The fetching process described above includes three steps:
- To enable the background fetch capability
- To set the minimum interval time
- To implement the new delegate method
application:performFetchWithCompletionHandler:
Let's examine things in greater detail.
As I just mentioned, the first step in the whole process is to enable the background fetch capability for the application in Xcode 5. This can be done in two ways. The first way is to use the .plist
file, where you need to add the Required background modes
key with the fetch
value, like this:

The following steps are an alternative approach:
- Click on the project name at the top left side in Xcode
- Click on the Capabilities tab in the middle window
- Under the Background Modes option, check the Background Fetch checkbox
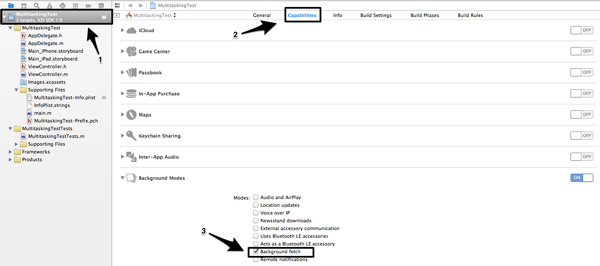
By default, the initial fetch interval value is set to the UIApplicationBackgroundFetchIntervalNever
mode, which means that the background fetching will never be performed. If this value doesn't get changed, the background fetch won't work, even if it is enabled with one of the two ways discussed above. To accomplish this, you need to go to the application:didFinishLaunchingWithOptions:
and set the minimum fetch interval value as follows:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { [application setMinimumBackgroundFetchInterval:UIApplicationBackgroundFetchIntervalMinimum]; return YES; }
The UIApplicationBackgroundFetchIntervalMinimum
is the minimum interval value allowed and managed by the system. Of course, you can set a custom time interval simply by providing the method above with a NSTimeInterval
value. However, unless it is necessary, it's generally better to let iOS decide when it should allow the app to work within the background and fetch new content. If you do set a minimum, it's important to realize that the UIApplicationBackgroundFetchIntervalMinimum
doesn't absolutely determine the timing. They are more like suggestions. iOS will try to follow the schedule if it can, but, depending on the available system resources, the fetching may happen more or less often than desired.
The last step required is to implement the newly added application:performFetchWithCompletionHandler:
delegate method. This one is called every time that a background fetch should be performed. The completion handler of the method accepts any of the following three values to let iOS know about the fetch results:
-
UIBackgroundFetchResultNewData
: If new data was found by the fetch -
UIBackgroundFetchResultNoData
: If no new data was found -
UIBackgroundFetchResultFailed
: If an error occurred during the fetch process
Let's see this in action:
-(void)application:(UIApplication *)application performFetchWithCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler{ // Call or write any code necessary to get new data, process it and update the UI. // The logic for informing iOS about the fetch results in plain language: if (/** NEW DATA EXISTS AND WAS SUCCESSFULLY PROCESSED **/) { completionHandler(UIBackgroundFetchResultNewData); } if (/** NO NEW DATA EXISTS **/) { completionHandler(UIBackgroundFetchResultNoData); } if (/** ANY ERROR OCCURS **/) { completionHandler(UIBackgroundFetchResultFailed); } }
In case new data is found, you must also make any necessary user interface updates. These must occur right away for two reasons: (1) users should see the content when the app is next launched, and (2) the app snapshot will remain updated.
There are a few more facts you should know. First, when the app launches in the background, it has about thirty seconds at its disposal to complete all of the required tasks and call the completion handler. If this time limit is exceeded, the app will become suspended again. For apps that need more than 30 seconds (perhaps because of media downloads), you should schedule a background fetch using the Background Transfer Service API. We'll discuss this option more later.
After you have your fetching mechanism set up and ready to work, Apple provides two ways to test the background fetch when using the Simulator.
The first approach follows these steps:
- Run the application as usual on the Simulator
- While the app is running, go back to Xcode
- Click on the Debug > Simulate Background Fetch menu
This way is useful to try the API while the app is running. The second way is more involved, but it allows you to test the app while it remains in the background. To try this, follow these steps:
- Go to the Schemes list and click on the Manage Schemes... option.
- Make sure that the current scheme is selected and then duplicate it by clicking on the gear button at the bottom side of the window and selecting Duplicate.
- Set a name for the duplicate if you'd like.
- Have the Run option selected at the left pane, then click on the Options tab.
- Check the Launch due to a background fetch event checkbox and click OK twice.
- Run the app using the duplicated scheme.
Background fetch is meant to be used for non-critical app updates since the time that it occurs may differ. For more critical updates, see the Remote Notifications section coming up next. Generally, the background fetch API is suitable for applications that want to seamlessly manage content. A few example app categories include news, social network, weather, and photo sharing.
Remote Notifications
Imagine that we build an application with both written and video tutorials. Let's suppose that users can download new tutorial videos 2-3 times per month. So, how would we make our users aware of new video releases? We can't adopt a solution that uses the background fetch API or something similar, as this would lead to both waste of device resources and pointless requests to the server. We also cannot know when a new video release is ready. Therefore, we need to use Push Notifications, so every time there is new content, we can let our users know about that simply by alerting them. We would expect our users to consequently launch the app and download new content. The problem is that this approach forces the user to wait for the video to download, and if the content is large enough, they may have to wait for a long time. So, how can users obtain new content in their application when this content is available but without the wait?
This is where Remote Notifications come in handy. Remote Notifications are actually silent push notifications, meaning push notifications that do not inform users about their existence. When a new remote notification arrives, the system silently wakes the app so it can handle the notification. The application is then responsible for initiating the download process for the new content. When it finishes downloading, a local notification is sent to the user. When the application is launched, the new content is there awaiting the user.
Just like Background Fetch, Remote Notifications aim to eliminate the waiting time that users face when downloading new content or uploading data to a server. Both of the APIs work within the background thread and users are notified after the job has been completed. However, Remote Notifications are suitable in cases where content is either infrequently updated or is otherwise critical to the user experience.
In order to use Remote Notifications within an application, you will first need to enable them. You can do this with either the *.plist file or the new Capabilities project tab. If you want to use the *.plist file, you need to add the Required Background key with the remote-notification value as follows:

To use the Capabilities tab, follow these steps:
- Click on the project name at the top left side in Xcode
- Click on the Capabilities tab in the middle window
- Under the Background Modes option, check the Remote Notifications checkbox
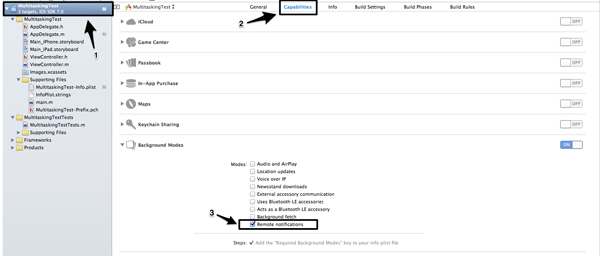
The next step is to implement the application:didReceiveRemoteNotification:fetchCompletionHandler:
delegate method in your AppDelegate file. This one is called when a new notification arrives. Use the completion handler to notify iOS about the result of the data fetch:
-(void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler{ // Call or write any code necessary to download new data. completionHandler(UIBackgroundFetchResultNewData); }
Making a notification silent is fairly easy. In the notification payload you just have to include the code-available: 1
flag and omit any alert or sound data that would notify the user.
aps{ content-available: 1 }
In general, everything that is valid when working with Background Fetch applies here too. For example, you have 30 seconds at maximum to download any new content and notify the system in the method above before the app goes to sleep again. If the new content needs time to be downloaded, then consider the use of the Background Transfer Service API.
Keep in mind that Apple controls the rate of the remote notification delivery (silent push notifications) that are sent from a push provider. If the rate is normal, then both normal and silent notifications are delivered right away. However, when silent notifications are being sent quite frequently, the Apple Push Service stores them for delivery at a later time. Make sure you send any remote notifications wisely.
Background Transfer Service
Another great feature of iOS 7 is the Background Transfer Service API. Traditionally, getting or sending big chunks of data has not been easy, mainly due to running time limitations and to transferred data management. Applications were required to finish most of the uploading or downloading process while they were in the foreground. However, with iOS 7, things are totally different. Applications can enjoy greater freedom when performing background tasks. First of all, with the background transfer service, applications are given as much time as they need to complete a transfer. Whether they are in the foreground or in the background is irrelevant. Time limitations no longer exist and the system is now responsible for managing the data that is being uploaded or downloaded. Even if an application is not running, iOS wakes it up to handle situations where decisions must be made. A few examples of this include when a file download completes or when more information should be provided to the server.
The background transfer service is based on the NSURLSession
class, which has just been introduced with iOS 7. This class is responsible for transferring data via HTTP or HTTPS, and it provides the ability for background network-related tasks. The whole idea behind it is simple and it is based on the use of a sessions, where a single session manages all related data transfer tasks. There are various session types available, but the background sessions is the one we care about here, and it always work on separate threads (processes) created by the system. Background sessions support both download (NSURLSessionDownloadTask
) and upload (NSURLSesssionUploadTask
) tasks, which are added to background NSURLSession
objects.
Another noteworthy fact related to background transfers is that a transfer can be discretionary or non-discretionary. Non-discretionary transfers can be initiated only when the application is in the foreground, but there is an option to set the discretionary state according to the application's needs. Discretionary transfers are mostly preferred, because these allow the operating system to achieve more efficient energy management on the device. There is also one limitation that applies on discretionary transfers, and that is that they should be performed only over WiFi networks. Background thread transfers are always initiated as discretionary transfers.
The delegate method application:handleEventsForBackgroundURLSession:completionHandler:
is called when a background transfer completes so the finished task can be handled. For example, if new content was downloaded, you should implement this delegate method to store it permanently and update the UI if needed. This method is also called when credentials are required from the app in order to continue the transfer. Generally, in this delegate method you apply all the necessary logic required by your application when a download or upload process is over and the application exists within the background thread. Of course, you use the completion handler to let the system know when you are done so it can put the app back to sleep.
The background transfer service is ideal for use in conjunction with the background fetch and the remote notification APIs. Granted the above, it becomes clear that the best practice is to use the delegate methods of the background fetch and the remote notifications to enqueue your transfers and let the system do the job. After all, unless the data that should be exchanged with the server has a small footprint, in most cases the 30 seconds provided to just the two mentioned delegate methods is enough to setup the background transfer and any related tasks. Use the NSURLSession
class to define all the transfers you want and to make sure that your app will fetch any new content. It will also send any data that should be processed, regardless of the time required.
Conclusion
Background Fetch, Remote Notifications, and the Background Transfer Service APIs are features that developers would love to have seen a long time ago. Thankfully, with the release of iOS 7 Apple has made it easier than ever to process application data. From iOS 7 onward, app content can virtually always be up-to-date, but it's ultimately up to each developer to take care of this and use the tools provided! Happy multitasking, and thanks for reading!
Comments