With the release of iOS 5, a small yet powerful framework is available for developers to quickly to integrate Twitter within an application. This tutorial will teach you how to make life much easier by using the Twitter framework!
In this two-part tutorial series, I will talk about the Twitter framework that was introduced with iOS 5. The first part covers TWTweetComposeViewController
, which makes composing and sending tweets as simple as presenting a modal view controller. The second part is more advanced as we take a closer look at TWRequest, a class designed to interact with the Twitter API in a reliable and straightforward manner. One of the features that most of you will enjoy is the built-in authentication handling. It completely removes the headaches associated with authentication. If OAuth has been giving you nightmares and headaches, then you are going to love the Twitter framework!
Project Summary
In this tutorial, I will show you how you can integrate the Twitter framework into an existing application and how to use TWTweetComposeViewController
to compose and send tweets from within your applications. We will build an application from scratch and add the capability to send tweets from within the application with surprisingly little effort.
Before We Start
Since iOS 5, Twitter is tightly integrated with the operating system and this is one of the reasons that interacting with Twitter has become very easy. The operating system now stores your Twitter account and this means that the user does not need to re-enter his Twitter credentials for every application that wants to talk to Twitter. As a developer, you only need to request access to the user's Twitter account, which results in a much better user experience. Another advantage for the developer is that authentication is handled by the operating system.
This also means that there has to be a Twitter account installed on your device (or iOS Simulator) if you wish to complete this tutorial. You can verify this by opening the Settings application on your device (or iOS Simulator) and selecting Twitter from the list. This will show you which accounts are associated with your device. To add an account, tap Add Account and enter your Twitter credentials. With that out of the way, let's start building our application.
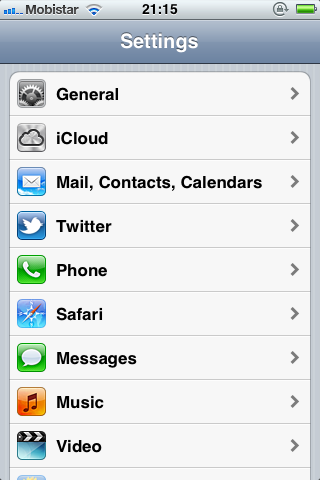
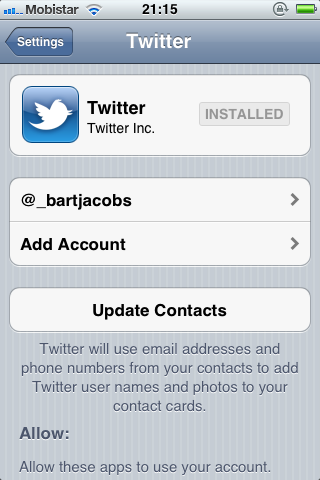
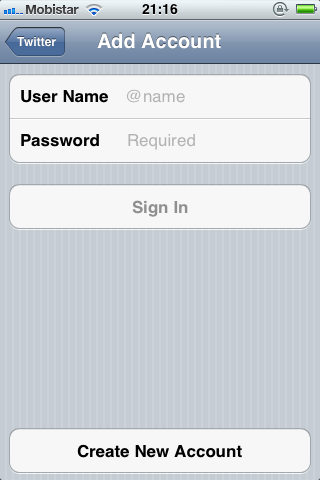
Project Setup
Create a new project in Xcode by selecting the Single View Application template from the list of templates. Name your application Twitter, enter a company identifier, set iPhone for the device family, and check Use Automatic Reference Counting. Make sure to uncheck the remaining checkboxes for this project. Tell Xcode where you want to save your project and hit Create.
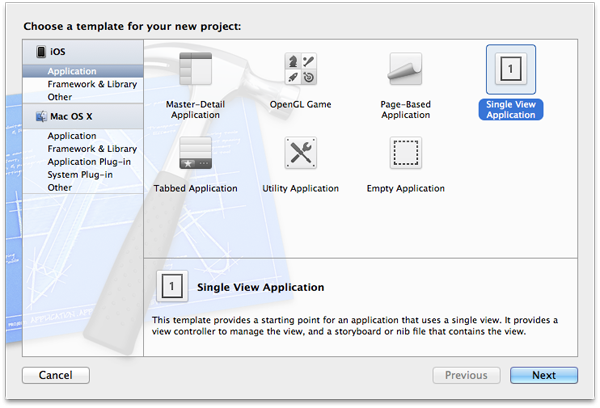
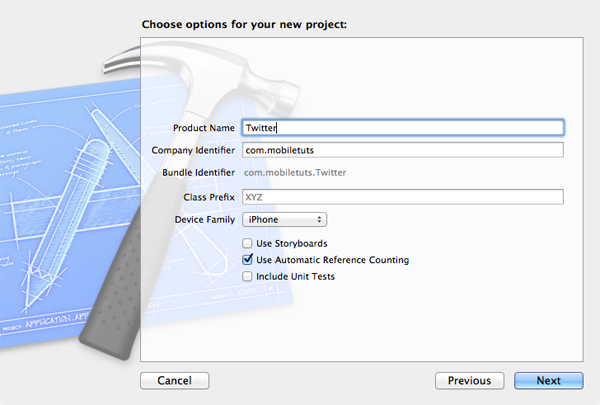
Setting Up the User Interface
Our application is going to be very simple in terms of user interface, we only need to create one action in our view controller's header file. Let's do that right now.
Head over to ViewController.h and create an IBAction
named tweet:. The action will be triggered by a button that we will add to our view controller's xib file. Don't forget to add an empty implementation of our action to ViewController.m to prevent any compiler warnings.
#import <UIKit/UIKit.h> @interface ViewController : UIViewController - (IBAction)tweet:(id)sender; @end
- (IBAction)tweet:(id)sender { }
Setting up the user interface in our view controller's xib file will take only a few seconds. Drag a UIButton
instance to the view controller's view and give it a title of Tweet. All that is left for us to do next is wire up our action and button. Control drag from the button to the File's Owner and select our action from the menu that pops up. This will link our action to the button's UIControlEventTouchUpInside
, which is what we want. That's all we need to do in our xib file for this tutorial.
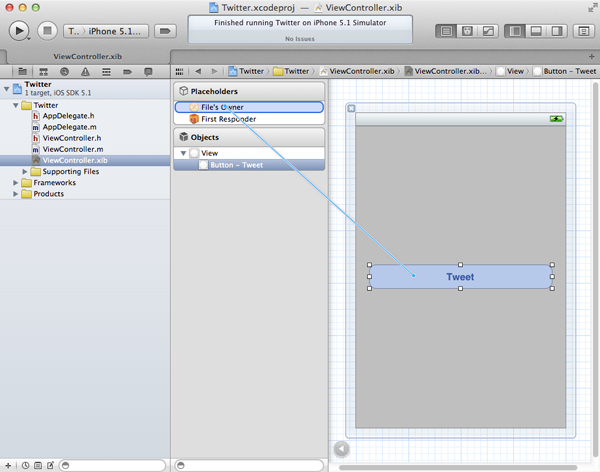
Adding the Twitter Framework
Before we can start using the Twitter framework, we need to add it to our project. Select our project in the Project Navigator and select our target from the targets list. Open the Build Phases tab at the top, open the Link Binary With Libraries drawer, click the plus button, and choose Twitter.framework from the list. At the top of our view controller's header file, we also need to add an import statement that imports the headers of the Twitter framework. We are now ready to start tweeting.
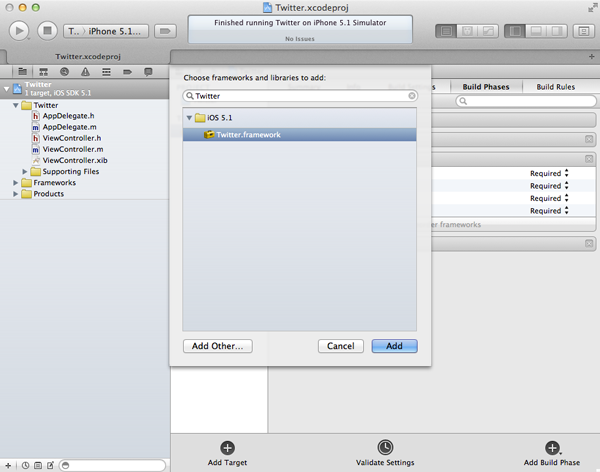
#import <Twitter/Twitter.h>
Head over to our view controller's implementation file and let's implement our tweet: method. I will walk you through the implementation step-by-step.
The first thing we need to do is check whether it is possible to send a tweet from the current device. We do this by sending TWTweetComposeViewController
the message canSendTweet. Note that this is a class method. Behind the scenes, the Twitter framework will check (1) if Twitter is accessible and (2) whether a Twitter account (or more) is available on the device. To give you an example, if the user has no network connection then this method will return NO, because it cannot access Twitter. Of course, we are good developers and we display an appropriate alert view when it is not possible to send a tweet. Build and run your application to make sure that we can send tweets.
if ([TWTweetComposeViewController canSendTweet]) { NSLog(@"I can send tweets."); } else { // Show Alert View When The Application Cannot Send Tweets NSString *message = @"The application cannot send a tweet at the moment. This is because it cannot reach Twitter or you don't have a Twitter account associated with this device."; UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Oops" message:message delegate:nil cancelButtonTitle:@"Dismiss" otherButtonTitles:nil]; [alertView show]; }
If we can send a tweet then we initialize our TWTweetComposeViewController
. TWTweetComposeViewController
is a subclass of UIViewController
and is designed to present the tweet compose view and send a tweet to Twitter. We can configure the TWTweetComposeViewController
to some degree by setting an initial text and adding an image or URL to the tweet. Let's set an initial text.
// Initialize Tweet Compose View Controller TWTweetComposeViewController *vc = [[TWTweetComposeViewController alloc] init]; // Settin The Initial Text [vc setInitialText:@"This tweet was sent using the new Twitter framework available in iOS 5."];
Finally, we present the tweet compose view controller modally. Build and run your application and have a look at what we've got so far.
// Display Tweet Compose View Controller Modally [self presentViewController:vc animated:YES completion:nil];
As you can see, the beauty of sending tweets using the TWTweetComposeViewController
is that you have very little overhead as a developer. You don't need to worry about the user interface and sending tweets takes place in the background. The user can also add their location to the tweet by tapping the Add Location button in the lower left of the tweet compose view.
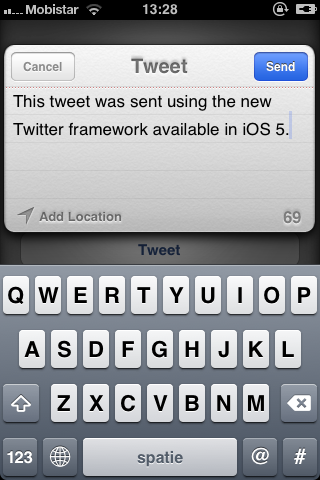
I want to show you a few more things about TWTweetComposeViewController
. Jump back to the implemenation of our tweet: method and let's add an image to our tweet. Before doing so, drag an image you want to share with the world into your project and make sure to copy it to your project and to add it to your target.
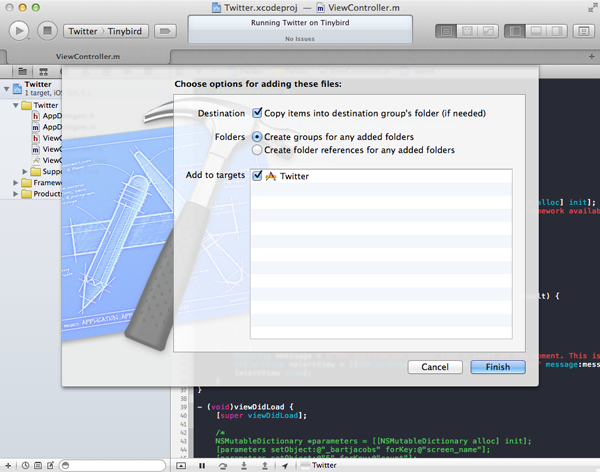
Adding the image to your tweet is very easy and requires only two lines of code. Build and run your application once again and see how the image is now attached to your tweet with a paperclip. As I mentioned earlier, you can add URLs as well. Even though the tweet compose view displays the image and URLs as attachments of your tweet, it is important to note that images and URLs reduce the character count of your tweet, which is limited to 140 characters max.
// Adding an Image UIImage *image = [UIImage imageNamed:@"sample.jpg"]; [vc addImage:image];
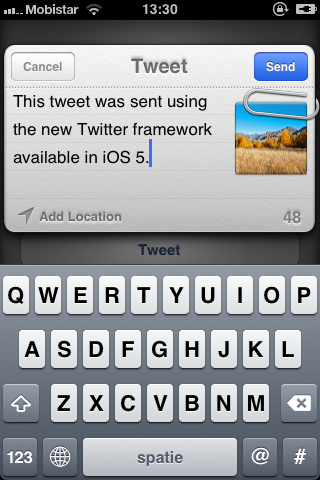
If you want to remove an image or URL from a tweet, you call removeAllImages and removeAllURLs, respectively, on your instance of TWTweetComposeViewController
. You can add multiple URLs to a tweet, but it seems that you can only add one image per tweet. It is unclear whether this is a deliberate choice made by Apple or a bug in the Twitter framework. As you saw earlier, the method to remove images from a tweet is removeAllImages, that is, plural.
To end this tutorial, I'd like to talk about one other aspect of TWTweetComposeViewController
, that is, the option to declare a completion handler. This is a block that is executed when the user tapped the cancel button or when the tweet was sent successfully to Twitter. The completion handler takes one argument, a flag that informs you whether posting the tweet was cancelled (TWTweetComposeViewControllerResultCancelled) or whether the tweet was sent to Twitter (TWTweetComposeViewControllerResultDone). The completion handler is a good place to dismiss the tweet compose view controller. Take a look at the complete implementation of our tweet: method.
- (IBAction)tweet:(id)sender { if ([TWTweetComposeViewController canSendTweet]) { // Initialize Tweet Compose View Controller TWTweetComposeViewController *vc = [[TWTweetComposeViewController alloc] init]; // Settin The Initial Text [vc setInitialText:@"This tweet was sent using the new Twitter framework available in iOS 5."]; // Adding an Image UIImage *image = [UIImage imageNamed:@"sample.jpg"]; [vc addImage:image]; // Adding a URL NSURL *url = [NSURL URLWithString:@"http://mobile.tutsplus.com"]; [vc addURL:url]; // Setting a Completing Handler [vc setCompletionHandler:^(TWTweetComposeViewControllerResult result) { [self dismissModalViewControllerAnimated:YES]; }]; // Display Tweet Compose View Controller Modally [self presentViewController:vc animated:YES completion:nil]; } else { // Show Alert View When The Application Cannot Send Tweets NSString *message = @"The application cannot send a tweet at the moment. This is because it cannot reach Twitter or you don't have a Twitter account associated with this device."; UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Oops" message:message delegate:nil cancelButtonTitle:@"Dismiss" otherButtonTitles:nil]; [alertView show]; } }
Conclusion
Sending tweets from within your application has become incredibly easy thanks to the tight integration of Twitter and the operating system. Yet TWTweetComposeViewController
may not be giving you not enough flexibility. Or perhaps you want to talk to the Twitter API directly? The TWRequest
class is the solution that you are looking for. I will talk about TWRequest
in the second part of this two-part series. Stay tuned!
Comments