You’ve probably seen it on Twitter, Google+, or Facebook. You’ve got a text box, where you write your status/message and then click a button to submit it. But, if you’re lazy like me, you don’t like to switch to the mouse to click the button. These services help us out by allowing us to press control + enter
to submit. Let’s recreate this scenario for our own projects.
Prefer Video?
Of course, the reason we can’t submit on just enter
is because we’ll be using a textarea
, so that the user can include line breaks. Normally, the browser will just ignore the control
key and add another line break when we hit control + enter
, but we’ll intercept this and perform our magic.
Step 1: The Template
We’re not here to talk about HTML and CSS so much, so here’s the “template” we start with:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Text Box Enter</title> <style> body { font: 16px/1.5 helvetica-neue, helvetica, arial, san-serif; } textarea { border: 1px solid #ccc; display:block; width: 250px; height: 100px; } p { border: 1px solid #ccc; background: #ececec; padding: 10px; margin: 10px 0; width: 230px; } button { border: 1px solid #ccc; background: #ececec; -webkit-border-radius: 3px; padding: 5px 20px; margin-top:10px; } </style> </head> <body> </body> </html>
Step 2: The HTML
We need a few element to work with here, so let’s add them:
<textarea id="msg"></textarea> <button type="submit">Post</button> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.min.js"></script> <script> </script>
I’m really simplifying this here: we only have a textarea
and a button
. If this were the real deal, we would want an official form
here, that would work and submit your message without JavaScript. We’re also including jQuery and an empty script tag that we will take advantage of next.
Step 3: The JavaScript
We’re going to make this as a jQuery plugin that we’ll call ctrlEnter
. Here’s what we start with:
$.fn.ctrlEnter = function (btns, fn) { var thiz = $(this); btns = $(btns); };
We’re taking two parameters. We will call this plugin function on the textarea
, so we already have that element. The first parameter is a string of one or more selectors that will be passed to jQuery. These are elements that must have the same functionality when clicked. The second parameter is the function that will be executed when control + enter
is pressed. Then, we’re creating variables: the jQueryified textarea
and the jQueryified btns
.
function performAction (e) { fn.call(thiz, e); }
Next, we create a function that wraps the function we passed in. We do this so that we can make sure the function is called with the textarea
element as this
within the function. We’re also passing it the event object from the event.
thiz.bind("keydown", function (e) { if (e.keyCode === 13 && e.ctrlKey) { performAction(e); e.preventDefault(); } }); btns.bind("click", performAction);
Next, we have the actual event handlers. The first wires a function to the keydown
event on the textarea
element. e.keyCode === 13
means the enter key is being pressed. If e.ctrlKey
is true, that means the user was pressing the control key when the enter key was pressed. If the enter key and control key are both being pressed, we’ll call that performAction
function. Then, we’ll call e.preventDefault
, which will prevent the newline that the enter key would normally write from happening.
And now, let’s wire up the event handlers to the buttons; we'll simply take the text, replace all occurances of \n
with <br />
, put it in a paragraph, and prepend it to the body:
$("#msg").ctrlEnter("button", function () { $("<p></p>").append(this.val().replace(/\n/g, "<br />")).prependTo(document.body); this.val(""); });
Now, let’s test it:
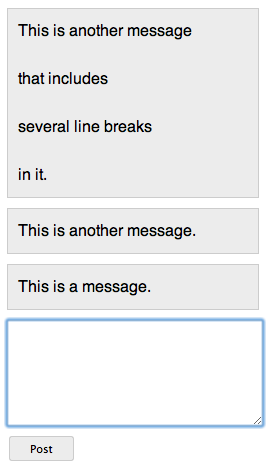
Conclusion: The End
We’ll that’s your quick tip for the day. Have another method of doing this? Hit the comments!
Comments