A good understanding of the iOS SDK is key when developing native iOS applications. Not only will it help you choose the right tools to tackle a particular problem, it will also make sure that you don't get lost in the dozens of frameworks that are included in the iOS SDK. In this article, we zoom in on the iOS architecture and find out what powers iOS applications under the hood.
What is the iOS SDK?
I am pretty sure that even a few experienced iOS developers would have a hard time defining the iOS SDK in one sentence. The acronym SDK stands for Software Development Kit. The iOS SDK contains the tools and resources to develop native iOS applications, which means that the SDK allows developers to develop, install, run, and test applications in the iOS Simulator and on physical devices.
The two driving forces powering native iOS applications are Objective-C and the native iOS system frameworks. In the previous article, we explored the Objective-C programming language. In this article, I want to explore the frameworks that power native iOS applications.
This includes high level frameworks, such as the UIKit and Map Kit frameworks, but also frameworks that are closely tied to the hardware, such as the Accelerate and Core Location frameworks.
What is a native iOS application?
You now know what the iOS SDK is, but what makes an application qualify as a native iOS application? The simple answer is that an iOS application is an application that runs on an iOS device. However, this is only half the truth. What about web applications that run in the Safari browser?
An iOS application is a Cocoa application developed for the iOS platform. Great. What is a Cocoa application? A Cocoa application is a bit harder to define. Is it the language in which the application is written? Not really. Is it the tools with which a Cocoa application is built? No. It is possible to develop a Cocoa application without the help of Xcode.
Apple defines a Cocoa application as an application that's composed of objects that ultimately inherit from NSObject
, a root class declared in the Foundation framework, and that's based upon the Objective-C runtime.
In this article, I want to focus on the frameworks that are used to create native iOS applications. If you're interested in learning more about the Objective-C runtime, I recommend taking a look at Apple's Objective-C Runtime Reference or read the Objective-C Runtime Programming Guide.
The Foundation framework provides a second root class, NSProxy
. However, you will rarely, if ever, use it in any of your projects.
The iOS Architecture
Another difference with web applications running in the Safari browser is that native applications interact directly with the iOS operating system and the native iOS system frameworks. The operating system acts as a mediator between the application and the underlying hardware. A key advantage of this mediation or abstraction is that native applications don't need to worry about future hardware changes or device specifications.
The operating system provides native applications with the necessary information about the hardware capabilities (Does the device have a camera?) and device specifications (Is the application running on an iPhone or an iPad?).
The iOS architecture can be broken down into four distinct layers:
- Cocoa Touch
- Media
- Core Services
- Core OS
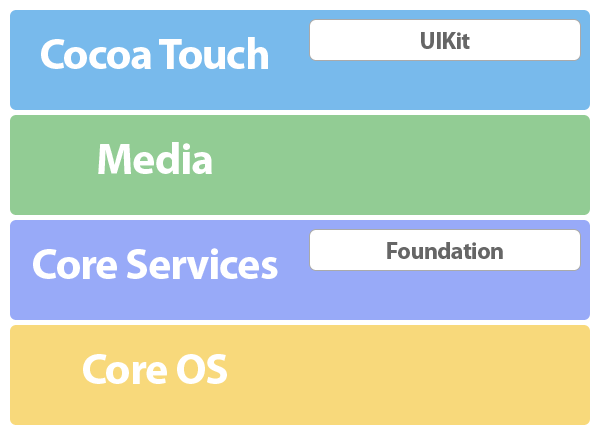
This layered architecture illustrates that level of abstraction, with the higher level layers more abstracted and the lower level layers more fundamental and closely tied to the hardware. It goes without saying that the higher level layers rely on the lower level layers for some of their functionality.
Apple recommends using the higher level frameworks as much as possible, because they are often object oriented abstractions of the lower level frameworks. In other words, the higher level layers interact indirectly with the hardware through the lower level layers, which are inherently more complex. Of course, it's still possible to fall back to the lower level frameworks if the higher level frameworks don't meet your needs.
As a reminder, a framework is a directory that contains a dynamic shared library and the resources associated with it, such as header files, images, etc. Frameworks are the access points to various system interfaces, such as the iOS address book, the device's camera roll, and the music library.
Cocoa Touch Layer
In the previous article, I wrote about Cocoa Touch and its relation to Objective-C. In this article, I would like to discuss Cocoa Touch from a more functional standpoint, how applications rely on the Cocoa Touch layer and what its role is in the iOS architecture.
The Cocoa Touch layer is the topmost layer of the iOS architecture. It contains some of the key frameworks which native iOS applications rely upon, with the most prominent being the UIKit framework.
The Cocoa Touch layer defines the basic application infrastructure and provides a number of vital technologies, such as multitasking and touch-based input.
As I mentioned, iOS applications rely heavily on the UIKit framework. Native iOS applications cannot operate if they are not linked against the UIKit and the Foundation frameworks.
The UIKit framework or UIKit is tailored to the iOS platform. There is an equivalent framework for the OS X platform named the Application Kit or AppKit framework. UIKit provides the infrastructure for graphical, event driven iOS applications. It also takes care of other core aspects that are specific to the iOS platform like multitasking, push notifications, and accessibility.
The Cocoa Touch layer provides developers with a large number of high level features, such as auto layout, printing, gesture recognizers, and document support. In addition to UIKit, it contains the Map Kit, Event Kit, and Message UI frameworks, among others.
For a complete list of all the frameworks of the Cocoa Touch layer, I'd like to refer to Apple's iOS Technology Overview guide.
Media Layer
Graphics, audio, and video are handled by the Media layer. This layer contains a number of key technologies, such as Core Graphics, OpenGL ES and OpenAL, AV Foundation, and Core Media.
The Media layer contains a large number of frameworks including the Assets Library framework to access a device's photos and videos, the Core Image framework for image manipulation through filters, and the Core Graphics framework for 2D drawing.
Take a look at Apple's iOS Technology Overview guide for a complete list of all the frameworks of the Media layer.
Core Services Layer
The Core Services layer is in charge of managing the fundamental system services that native iOS applications use. The Cocoa Touch layer relies heavily on the Core Services layer for some of its functionality. The Core Services layer also provides a number of indispensable features, such as block objects, Grand Central Dispatch, In-App Purchase, and iCloud Storage.
One of the most welcomed additions to the Core Services layer is ARC or Automatic Reference Counting. ARC is a compiler-level feature, introduced in 2011 with the release of iOS 5. ARC simplifies the process of memory management in Objective-C.
Memory management is a topic we haven't covered in this series, but it's important that you understand the basics of memory management when developing Cocoa applications. Automatic Reference Counting is a great addition, but it's important that you know what ARC is doing for you.
You can read more about memory management in The Objective-C Programming Language guide and I strongly recommend that you do.
The Foundation framework or Foundation is another essential framework for iOS and OS X applications. In the next article, we will explore this framework in more detail. The Foundation framework is more than a collection of useful classes, such as NSArray
, NSDictionary
, and NSDate
.
Foundation provides the NSObject
root class, which provides a basic interface to the Objective-C runtime, and it also introduces several paradigms, such as policies for object ownership. Like Core Foundation (see below), Foundation makes it possible for the different libraries and frameworks to easily share data and code.
Another important framework of the Core Services layer and closely tied to the Foundation framework is the C-based Core Foundation framework or Core Foundation. Like the Foundation framework, it enables the various libraries and frameworks to share data and code.
Core Foundation has a feature referred to as toll-free bridging, which enables the substitution of Cocoa objects for Core Foundation objects in function parameters and vice versa.
For a complete list of all the frameworks of the Core Services layer, I'd like to refer to the iOS Technology Overview guide.
Core OS Layer
Most of the functionality provided by the three higher level layers is built upon the Core OS layer and its low level features. The Core OS layer provides a handful of frameworks that your application can use directly, such as the Accelerate and the Security frameworks.
The Core OS layer also encapsulates the kernel environment and low level UNIX interfaces to which your application has no access for obvious security reasons. However, through the libSystem library, which is C-based, many low level features can be accessed directly, such as BSD sockets, POSIX threads, and DNS services.
Documentation
Your closest ally when developing native iOS applications is the documentation included with the iOS SDK. For the most part, the documentation is outstanding and it will help you get up to speed with a new technology or framework with little effort.
Even though the documentation is available online, most developers use the documentation browser that's included in Xcode. In Xcode 5, You can find the documentation browser by selecting Documentation and API Reference from Xcode's Window menu.
Since you'll be using the documentation extensively, you might want to learn a few shortcuts to find what you are looking for in the documentation. As I mentioned in the previous paragraph, the documentation browser provides easy access to the documentation. To quickly access the documentation, press Shift + Command + 0
in Xcode 5.
While writing code in Xcode's code editor, it can quickly become cumbersome to switch back and forth between the code editor and the documentation browser every time you need to look up a class or method.
There are two solutions for efficiently browsing the documentation. Whenever you place your cursor on a class or method name in Xcode's code editor, the Quick Help Inspector in the right sidebar shows a summary of the respective class or method. The summary contains several useful links that take you to the documentation browser.
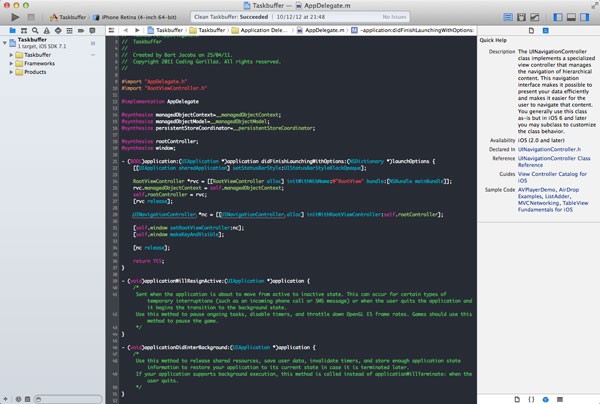
Because I usually hide the right sidebar when I'm working in the code editor, I use an alternative method to show the documentation of a class or method. Whenever you press the Option
key and hover over a class or method name, the cursors changes to a question mark and the class or method name is highlighted. By clicking a class or method name with the question mark, a new window pops up containing the same summary as the Quick Help Inspector. Clicking one of the links in the window takes you to the documentation browser. This is a fast and convenient way to switch between the code editor and the documentation browser, especially when working with two monitors.
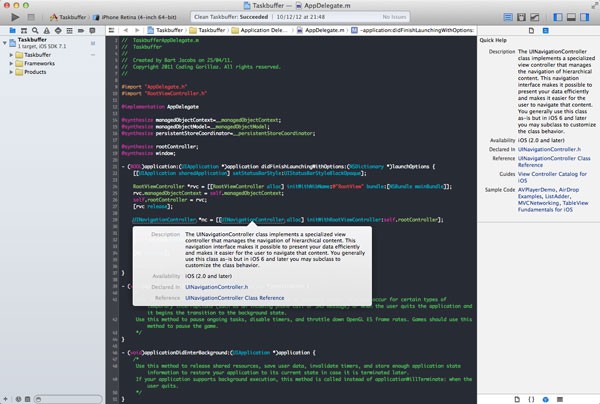
Conclusion
You should now have a good understanding of the iOS SDK and the various layers of the iOS architecture. The two core frameworks of an iOS application, UIKit and Foundation, are the focus of the next two installments of this series.
Not only are these frameworks indispensable for every iOS application, they contain dozens of classes that you will find yourself using often when developing native iOS applications.
Comments