This Android quick tip will introduce you to the various data storage options available on the Android platform. A brief practical overview with considerations for each storage option will also be provided.
When you develop Android applications, you have a number of options in terms of how you store and manage your data. Depending on the app, you may find yourself using more than one of these for different data tasks.
Internal Storage
You can store data items to the internal storage on your users' devices. The advantage to this is that it does not rely on any external media, but the disadvantage is that some devices have extremely limited amounts of storage space available. Storing to internal memory essentially means saving data files within the internal device directory structure.
The following Java code demonstrates opening a file for data output:
//get a FileOutputStream object by passing the file-name FileOutputStream dataFileOutput = openFileOutput("datafile", Context.MODE_PRIVATE);
When you save files to the internal storage, other applications can't access them and, by default, neither can the user, so it offers good reliability. The FileOutputStream write method takes byte parameters, so you can only use this technique to store data items your program can convert to bytes.
SD Cards
Many Android users are dependent on external media storage such as SD cards due to insufficient internal storage. SD cards offer your apps an increased amount of storage space, but they impose additional considerations. For example, you cannot assume that a user will have an SD card or other external media resource. For this reason your programming code needs to carry out checks before storing data in this way. You also need to accommodate the possibility of the user changing their SD card.
Using the Environment class, your apps can check the availability of writeable external storage as follows:
if(Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) { //can use the external storage }
It's also worth bearing in mind that files saved to an SD card can be accessed by other apps and by the user directly, so the data is more vulnerable to corruption.
Databases
If your app uses more complex data items, you can create a SQLite database to store them using the relational model. When you create a SQLite database for your app, you can access it from any point within your Java code, and it will not be accessible from anywhere else. There are many advantages to using a database, such as the ability to store and execute structured queries.
To build a custom SQLite database from your Java code, you can extend the SQLiteOpenHelper class, then define and create your tables inside the "onCreate" method, as follows:
public void onCreate(SQLiteDatabase db) { db.execSQL("CREATE TABLE Item (ItemID INTEGER, ItemName TEXT);"); }
This statement creates a database table with two columns in it. The SQLiteDatabase class provides the means to manage the data, including query, insert and update methods. One potential downside to using an SQLite database in your Android apps is the amount of processing code required, and the necessary skill set. If you already know your way around SQL, you should have no problems.
Shared Preferences
If you need to store simple data items for your apps, the most straightforward approach is to use Shared Preferences. This is possibly the easiest data management option to implement, but it's only suitable for primitive type items such as numbers and text. Using Shared Preferences, you model your data items as key value pairs. The following code demonstrates acquiring a reference to the SharedPreferences object for an app and writing a value to it:
//get the preferences, then editor, set a data item SharedPreferences appPrefs = getSharedPreferences("MyAppPrefs", 0); SharedPreferences.Editor prefsEd = appPrefs.edit(); prefsEd.putString("dataString", "some string data"); prefsEd.commit();
Anything you save to Shared Preferences will still be available the next time your app runs, so it's ideal for saving items such as user preferences and settings. When your app starts up, you can check the Shared Preferences, then present your interface and functionality accordingly. The following code demonstrates retrieving the string data item:
//get the preferences then retrieve saved data, specifying a default value SharedPreferences appPrefs = getSharedPreferences("MyAppPrefs", 0); String savedData = appPrefs.getString("dataString", "");
Web Storage
Since Android devices provide Internet connectivity, your apps can of course use data stored on the Web. Naturally, you need to consider how your applications will cope with restricted or missing connectivity. As with a Web application, this option allows you to store data using virtually any platform or model you prefer. The Java language also benefits from a range of standard libraries for handling data fetched over a network, from sources such as SQL databases and XML documents. The packages java.net and android.net provide classes for managing networked data.
Conclusion
If you're developing in Eclipse, you can manually control aspects of data storage on virtual devices in the DDMS perspective.
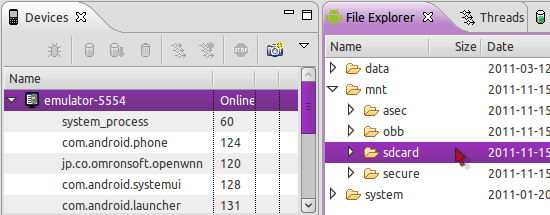
Inevitably, you need to tailor your data management approach to your own Android development projects. The platform is a flexible one, so there's plenty of choice available. When you do implement data management within your apps, make sure you test them on actual devices as well as in the emulator, as there's more potential for error when users access your functionality within their own unique contexts.
About the Author
Sue Smith
I'm a Web/Software Developer and technical/comedy writer - see BeNormal.info for details. The Nonsense apps are my first attempt at Android development. I've written for lots of different clients, including Smashing Magazine. My own sites include BrainDeadAir spoof arts magazine. Follow me on Twitter @BrainDeadAir or email me at [email protected].
Comments