In the previous part of this tutorial series, we added the Ladda loading indicator into our application to make it more interactive. We also saw how to filter the data result while fetching data from firebase. In this part of the tutorial, we'll add the logout functionality and fix a couple of issues.
Getting Started
Let's start by cloning the previous part of the tutorial from GitHub.
git clone https://github.com/jay3dec/AngularJS_Firebase_Part7.git
After cloning the source code, navigate to the project directory and install the required dependencies.
cd AngularJS_Firebase_Part7 npm install
Once the dependencies are installed, start the server.
npm start
Point your browser to http://localhost:8000/app/#/home and you should have the application running.
Implementing the Logout Functionality
Adding the Logout Button
We'll start by adding the logout button to the addPost.html
and welcome.html
views. Open both pages and add a new anchor tag to the nav element.
<nav class="blog-nav"> <a class="blog-nav-item " href="#/welcome">Home</a> <a class="blog-nav-item active" href="#/addPost">Add Post</a> <a class="blog-nav-item " style="cursor:pointer;" ng-click="logout();">Logout</a> </nav>
Implementing Logout
When we want to log a particular user out, we can call the $unauth
firebase API. We'll create a method in our CommonProp
AngularJS service so that it's available across controllers. Open home.js
and modify the CommonProp
service to include a new function called logoutUser
.
logoutUser:function(){ // logout the user }
Now inside the logoutUser
function, use the loginObj
to make the $unauth
API call and redirect to the home page. Here is the modified CommonProp
service.
.service('CommonProp',['$location','$firebaseAuth',function($location,$firebaseAuth) { var user = ''; var firebaseObj = new Firebase("https://blistering-heat-2473.firebaseio.com"); var loginObj = $firebaseAuth(firebaseObj); return { getUser: function() { return user; }, setUser: function(value) { user = value; }, logoutUser:function(){ loginObj.$unauth(); console.log('done logout'); $location.path('/home'); } }; }])
In the AddPostCtrl
and WelcomeCtrl
controllers, add a function called logout
which will make the logout call to the CommonProp
service.
$scope.logout = function(){ CommonProp.logoutUser(); }
Save the above changes and sign in using a valid email address and password. Once signed in, you should be able to view the Logout link in the top menu. Clicking on the Logout link should log you out of the application.
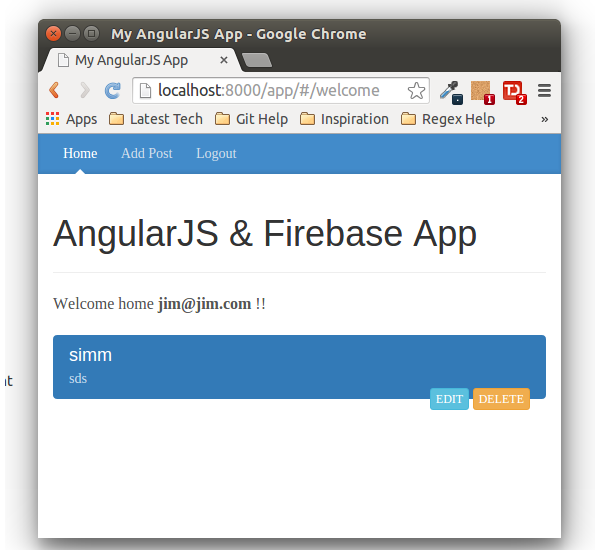
Maintaining Page Data on Refresh
Until now everything looks fine and works well. But after you have signed in to the application, if you try to refresh the page everything gets messed up. So, we need to find a way to maintain the page data. We'll make use of HTML 5 local storage to sustain important data.
Once the user has signed in successfully, we'll keep the email address in local storage. Modify the setUser
function in the CommonProp
service to store the user email address in local storage.
setUser: function(value) { localStorage.setItem("userEmail", value); user = value; }
Now, while getting the user email address from the CommonProp
service, it'll fetch the email address from the local storage and return.
getUser: function() { if(user == ''){ user = localStorage.getItem('userEmail'); } return user; }
Also make sure to remove the local storage data and the user variable from the CommonProp
service when the user logs out.
logoutUser:function(){ loginObj.$unauth(); user=''; localStorage.removeItem('userEmail'); $location.path('/home'); }
Save the above changes and sign in using a valid email address and password. Once signed in, try to refresh the page and the page should work as expected.
Preventing Unauthorized Page Access
Right now users are able to access all the pages, irrespective of whether they are logged in or not. In order to prevent unauthorized access, we'll check for user session.
In the WelcomeCtrl
controller, add the following code to get the current logged-in user. If the user doesn't exist then redirect the user to the home page.
$scope.username = CommonProp.getUser(); if(!$scope.username){ $location.path('/home'); }
Similarly add the following code checking to the AddPostCtrl
controller.
if(!CommonProp.getUser()){ $location.path('/home'); }
Save the above changes and try to load the Welcome page or the Add Post page without logging in, and you should be redirected to the sign-in page.
Auto Log In User on Valid Session
We'll add one more feature to auto log in the user when on the sign-in page. We'll make use of the $onAuth
API which will listen for changes in authentication state. When the user loads the sign-in page and an authentication state occurs due to a valid session, $onAuth
will be fired. Add the $onAuth
API call in the HomeCtrl
controller.
loginObj.$onAuth(function(authData) { //fire when authentication state occurs });
If the authData
is not null in the returned callback, then it's a valid session. So, add the code to save the user details in the CommonProp service and redirect to the welcome page.
loginObj.$onAuth(function(authData) { if(authData){ CommonProp.setUser(authData.password.email); $location.path('/welcome'); } });
Save the above changes and sign in to the application. Once logged in, close the application without logging out. Now, try to load the sign-in page and you should be automatically logged in to the application.
Wrapping It Up
In this part of the series, we implemented the logout functionality and also sorted out some of the issues like preventing unauthorized user access and maintaining data on page refresh.
In the coming tutorials, I'll try to implement a few features requested by users. I welcome any feature requests that you would like me to implement.
Watch out for the upcoming tutorial by checking my instructor page. It includes all the tutorials as soon as they are published.
Do let us know your thoughts, suggestions and corrections in the comments below. Source code from this tutorial is available on GitHub.
Comments