In this tutorial, you will learn how to build an ASP.NET server control by creating a HTML5 video player control. Along the way, we'll review the fundamental process of server control development from scratch.
Introduction
ASP.NET comes with its own set of server-side controls, so why create our own?
By creating our own controls, we can then build powerful, reusable visual components for our Web application’s user interface.
This tutorial will introduce you to the process of ASP.NET server control development. You’ll also see how creating your own controls can simultaneously improve the quality of your Web applications, make you more productive and improve your user interfaces.
ASP.NET custom controls are more flexible than user controls. We can create a custom control that inherits from another server-side control and then extend that control. We can also share a custom control among projects. Typically, we will create our custom control in a web custom control library that is compiled separately from our web application. As a result, we can add that library to any project in order to use our custom control in that project.
HTML5 Video Overview
Until now, there has never been a native way to display video on a web page. Today, most videos are shown, via the use of a plugin (like Flash or Silverlight). However, not all browsers have the same plugins. HTML5 specifies a standard, native way to include video, with the video
element.
Currently, there are two widely supported video formats for the video element: Ogg
files [encoded with Theora and Vorbis for video and audio respectively] and MPEG 4
files [encoded with H.264 and AAC].
To show a video in HTML5, this is all we need:
<video width="320" height="240" controls="controls"> <source src="movie.ogg" type="video/ogg" /> <source src="movie.mp4" type="video/mp4" /> </video>
The controls
attribute is for adding play, pause and volume controls. Without this attribute, your video would appear to be only an image. It is also always a good idea to include both the width
and height
attributes. The following table shows all attributes of the <video>
element:
- autoplay: Specifies that the video will start playing as soon as it is ready
- controls: Specifies that controls will be displayed, such as a play button
- height: The height of the video player
- loop: Specifies that the media file will start over again, every time it is finished
- preload: Specifies that the video will be loaded at page load, and ready to run. Ignored if "autoplay" is present
- src: The URL of the video to play
- width: The width of the video player
- poster: The URL of the image to show while no video data is available
Step 0: Getting Started
All that is required to get started is a copy of Visual Studio of Visual Web Developer Express. If you don't have the full version of Visual Studio, you can grab the free Express Edition.
The HTML5 video player that we will create here is only a simple video player that will render whatever native interface the browser provides. Browsers that support HTML5 video have video players built in, including a set of controls (play/pause etc.), so you will see a different interface for each browser when running this control.
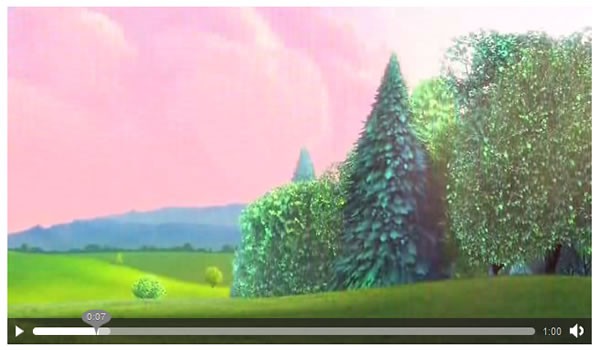
Step 1: Creating a Custom Control Project
First, we need to create a new class library project to hold our custom controls. By creating the custom control in a separate class library, we can compile the project into a separate DLL and use the custom control in any application that requires it.
Open your ASP.NET project with Visual Studio or Visual Web Developer. In Solution Explorer, right click the solution name, and select Add New Project
from the context menu. In the Add New Project dialog box, choose the project type to be a Web
project, and select ASP.NET Server Control
as the template, like so:
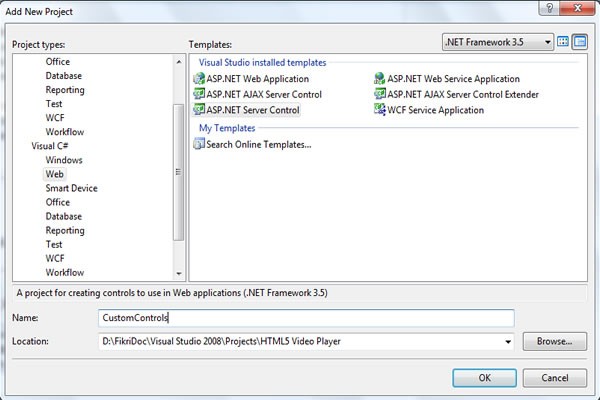
Name the project CustomControls
. Click OK
. The new ASP.NET Server Control project is created, and Visual Studio also provides you with a simple Web control to start with. Delete this custom control because we don't need it.
Step 2: Adding a Web Custom Control to the Project
In Solution Explorer, right click the CustomControls
project, and select Add New Item
from the context menu. In the Add New Item
dialog box, choose the category type to be a Web
category, and select ASP.NET Server Control
in the templates.
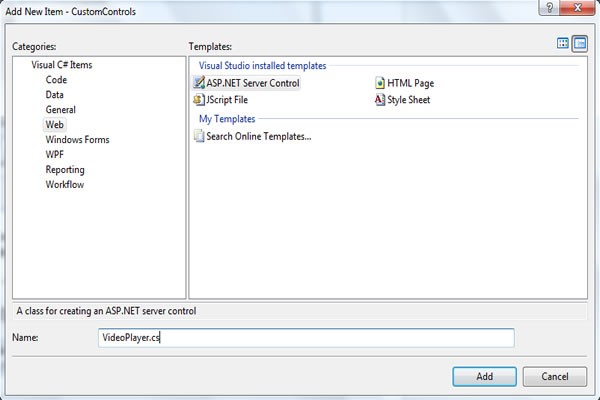
Name the new custom control VideoPlayer
. Click Add
. The new custom control (VideoPlayer.cs
) is created and added to the CustomControls project.
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace CustomControls { [DefaultProperty("Text")] [ToolboxData("<{0}:VideoPlayer runat=server></{0}:VideoPlayer>")] public class VideoPlayer : WebControl { [Bindable(true)] [Category("Appearance")] [DefaultValue("")] [Localizable(true)] public string Text { get { String s = (String)ViewState["Text"]; return ((s == null) ? "[" + this.ID + "]" : s); } set { ViewState["Text"] = value; } } protected override void RenderContents(HtmlTextWriter output) { output.Write(Text); } } }
The code above is the default code generated by Visual Studio for a web control library. To start working with VideoPlayer.cs
, we need to modify the code above. The first thing that we should do is delete everything between the class declaration line and the end of the class. That leaves us with this code:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace CustomControls { [DefaultProperty("Text")] [ToolboxData("<{0}:VideoPlayer runat=server></{0}:VideoPlayer>")] public class VideoPlayer : WebControl { } }
As you see above, the VideoPlayer
class derives from the System.Web.UI.WebControl
class. In fact, all ASP.NET server-side controls derive from the WebControl
class.
Step 3: Modifying the Class Declaration Line
The class declaration line in the default code also specifies the default property for the VideoPlayer
control as the Text
property. The VideoPlayer
control that we create here doesn't have a property called Text
. So, delete the reference to Text
as the default property. After all the modifications, the VideoPlayer.cs
code file should look like this:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace CustomControls { [ToolboxData("<{0}:VideoPlayer runat=server></{0}:VideoPlayer>")] public class VideoPlayer : WebControl { } }
Step 4: Adding Properties
In this step, we will add some properties to the VideoPlayer control to handle the control's behaviour. The following is the list of properties that we will add to the VideoPlayer.cs
code file:
-
VideoUrl
: A string property which specifies the URL of the video to play. -
PosterUrl
: A string property which specifies the address of an image file to show while no video data is available. -
AutoPlay
: A boolean property to specify whether the video should automatically start playing or not, when the webpage is opened. -
DisplayControlButtons
: A boolean property that specifies whether the player navigation buttons are displayed or not. -
Loop
: A boolean property that specifies whether the video will start over again or not, every time it is finished.
Add the following code to the VideoPlayer class:
private string _Mp4Url; public string Mp4Url { get { return _Mp4Url; } set { _Mp4Url = value; } } private string _OggUrl = null; public string OggUrl { get { return _OggUrl; } set { _OggUrl = value; } } private string _Poster = null; public string PosterUrl { get { return _Poster; } set { _Poster = value; } } private bool _AutoPlay = false; public bool AutoPlay { get { return _AutoPlay; } set { _AutoPlay = value; } } private bool _Controls = true; public bool DisplayControlButtons { get { return _Controls; } set { _Controls = value; } } private bool _Loop = false; public bool Loop { get { return _Loop; } set { _Loop = value; } }
After we have added the above properties, the VideoPlayer class should look like
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace CustomControls { [ToolboxData("<{0}:VideoPlayer runat=server></{0}:VideoPlayer>")] public class VideoPlayer : WebControl { private string _Mp4Url; public string Mp4Url { get { return _Mp4Url; } set { _Mp4Url = value; } } private string _OggUrl = null; public string OggUrl { get { return _OggUrl; } set { _OggUrl = value; } } private string _Poster = null; public string PosterUrl { get { return _Poster; } set { _Poster = value; } } private bool _AutoPlay = false; public bool AutoPlay { get { return _AutoPlay; } set { _AutoPlay = value; } } private bool _Controls = true; public bool DisplayControlButtons { get { return _Controls; } set { _Controls = value; } } private bool _Loop = false; public bool Loop { get { return _Loop; } set { _Loop = value; } } } }
Step 5: Creating the RenderContents Method
The primary job of a server control is to render some type of markup language to the HTTP output stream, which is returned to and displayed by the client. It is our responsibility as the control developer to tell the server control what markup to render. The overridden RenderContents
method is the primary location where we tell the control what we want to render to the client.
Add the following override RenderContents
method to the VideoPlayer
class:
protected override void RenderContents(HtmlTextWriter output) { }
Notice that the RenderContents
method has one method parameter called output
. This parameter is an HtmlTextWriter
object, which is what the control uses to render HTML to the client. The HtmlTextwriter
class has a number of methods you can use to render your HTML, including AddAttribute
and RenderBeginTag
.
Step 6: Adding Tag Attributes
Before we write the code to render the <video>
element, the first thing to do is add some attributes for it. We can use the AddAttribute
method of the HtmlTextWriter
object to add attributes for HTML tags.
Append the following code into the RenderContents
method:
output.AddAttribute(HtmlTextWriterAttribute.Id, this.ID); output.AddAttribute(HtmlTextWriterAttribute.Width, this.Width.ToString()); output.AddAttribute(HtmlTextWriterAttribute.Height, this.Height.ToString()); if (DisplayControlButtons == true) { output.AddAttribute("controls", "controls"); } if (PosterUrl != null) { output.AddAttribute("poster", PosterUrl); } if (AutoPlay == true) { output.AddAttribute("autoplay", "autoplay"); } if (Loop == true) { output.AddAttribute("loop", "loop"); }
You can see that, by using the AddAttribute
method, we have added seven attributes to the tag. Also notice that we are using an enumeration, HtmlTextWriterAttribute
, to select the attribute we want to add to the tag.
After we have added the code above, the RenderContents
method should look like so:
protected override void RenderContents(HtmlTextWriter output) { output.AddAttribute(HtmlTextWriterAttribute.Id, this.ID); output.AddAttribute(HtmlTextWriterAttribute.Width, this.Width.ToString()); output.AddAttribute(HtmlTextWriterAttribute.Height, this.Height.ToString()); if (DisplayControlButtons == true) { output.AddAttribute("controls", "controls"); } if (PosterUrl != null) { output.AddAttribute("poster", PosterUrl); } if (AutoPlay == true) { output.AddAttribute("autoplay", "autoplay"); } if (Loop == true) { output.AddAttribute("loop", "loop"); } }
Step 7: Rendering the <video> Element
After adding some tag attributes for the video element, it's time to render the <video>
tag with its attributes onto the HTML document. Add the following code into the RenderContents
method:
output.RenderBeginTag("video"); if (OggUrl != null) { output.AddAttribute("src", OggUrl); output.AddAttribute("type", "video/ogg"); output.RenderBeginTag("source"); output.RenderEndTag(); } if (Mp4Url != null) { output.AddAttribute("src", Mp4Url); output.AddAttribute("type", "video/mp4"); output.RenderBeginTag("source"); output.RenderEndTag(); } output.RenderEndTag();
We use the RenderBeginTag
method of output
object to render the opening tag of the video element, and RenderEndTag
to render its closing tag. We also added the <source>
element between the <video>
element. The video element allows multiple source elements. Source
elements can link to different video files. The browser will use the first recognized format.
The RenderContents
method should look like this after we have added the code above:
protected override void RenderContents(HtmlTextWriter output) { output.AddAttribute(HtmlTextWriterAttribute.Id, this.ID); output.AddAttribute(HtmlTextWriterAttribute.Width, this.Width.ToString()); output.AddAttribute(HtmlTextWriterAttribute.Height, this.Height.ToString()); if (DisplayControlButtons == true) { output.AddAttribute("controls", "controls"); } if (PosterUrl != null) { output.AddAttribute("poster", PosterUrl); } if (AutoPlay == true) { output.AddAttribute("autoplay", "autoplay"); } if (Loop == true) { output.AddAttribute("loop", "loop"); } output.RenderBeginTag("video"); if (OggUrl != null) { output.AddAttribute("src", OggUrl); output.AddAttribute("type", "video/ogg"); output.RenderBeginTag("source"); output.RenderEndTag(); } if (Mp4Url != null) { output.AddAttribute("src", Mp4Url); output.AddAttribute("type", "video/mp4"); output.RenderBeginTag("source"); output.RenderEndTag(); } output.RenderEndTag(); }
Notice that the order in which we place the AddAttributes
methods is important. We place the AddAttributes
methods directly before the RenderBeginTag
method in the code. The AddAttributes
method associates the attributes with the next HTML tag that is rendered by the RenderBeginTag
method, in this case the video
tag.
Step 8: Removing the Span Tag
By default, ASP.NET will surround the control tag with a <span>
element when rendering the control's HTML markup. If we have provided an ID
value for our control, then the Span
tag will also, by default, render an ID
attribute. Having the tags can sometimes be problematic, so if we want to prevent this in ASP.NET, we can simply override the Render
method and call the RenderContents
method directly. Here's how to do that:
protected override void Render(HtmlTextWriter writer) { this.RenderContents(writer); }
After we have added the code above, the VideoPlayer class should look like this:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace CustomControls { [ToolboxData("<{0}:VideoPlayer runat=server></{0}:VideoPlayer>")] public class VideoPlayer : WebControl { private string _Mp4Url; public string Mp4Url { get { return _Mp4Url; } set { _Mp4Url = value; } } private string _OggUrl = null; public string OggUrl { get { return _OggUrl; } set { _OggUrl = value; } } private string _Poster = null; public string PosterUrl { get { return _Poster; } set { _Poster = value; } } private bool _AutoPlay = false; public bool AutoPlay { get { return _AutoPlay; } set { _AutoPlay = value; } } private bool _Controls = true; public bool DisplayControlButtons { get { return _Controls; } set { _Controls = value; } } private bool _Loop = false; public bool Loop { get { return _Loop; } set { _Loop = value; } } protected override void RenderContents(HtmlTextWriter output) { output.AddAttribute(HtmlTextWriterAttribute.Id, this.ID); output.AddAttribute(HtmlTextWriterAttribute.Width, this.Width.ToString()); output.AddAttribute(HtmlTextWriterAttribute.Height, this.Height.ToString()); if (DisplayControlButtons == true) { output.AddAttribute("controls", "controls"); } if (PosterUrl != null) { output.AddAttribute("poster", PosterUrl); } if (AutoPlay == true) { output.AddAttribute("autoplay", "autoplay"); } if (Loop == true) { output.AddAttribute("loop", "loop"); } output.RenderBeginTag("video"); if (OggUrl != null) { output.AddAttribute("src", OggUrl); output.AddAttribute("type", "video/ogg"); output.RenderBeginTag("source"); output.RenderEndTag(); } if (Mp4Url != null) { output.AddAttribute("src", Mp4Url); output.AddAttribute("type", "video/mp4"); output.RenderBeginTag("source"); output.RenderEndTag(); } output.RenderEndTag(); } protected override void Render(HtmlTextWriter writer) { this.RenderContents(writer); } } }
Our control is now finished! All we have left to do is build the project before we use it on a ASP.NET web page.
Step 9: Building the Project
It's time to build the project. Select Build
, and then click Build Solution
from the main menu.
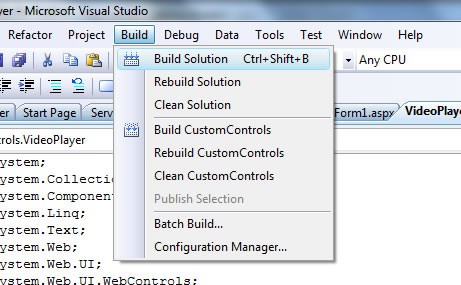
After building the project, the next step is to add the VideoPlayer control into the Toolbox Explorer
.
Step 10: Adding VideoPlayer Control to the Visual Studio Toolbox
- To add the VideoPlayer control to the Toolbox, right click in the
Toolbox Explorer
-
Choose Items
from the context menu - Click the
Browse
button in the Choose Toolbox Items dialog box - Navigate to the ASP.NET project directory
- Go to the
CustomControls
directory - Open the
Bin\Debug
directory (Visual Studio builds debug versions by default.) - Select the
CustomControls.DLL
assembly and click on theOpen
button
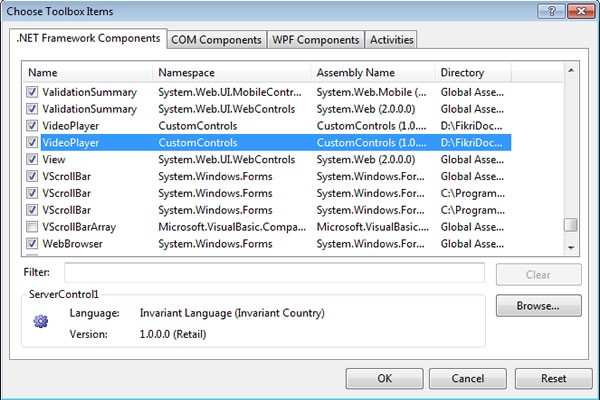
VideoPlayer
will appear in the Choose Toolbox Items dialog box as shown in the image above. The check box will show it as selected. As soon as you click the OK
button in the Choose Toolbox Items dialog box, the new VideoPlayer control will appear in the toolbox.
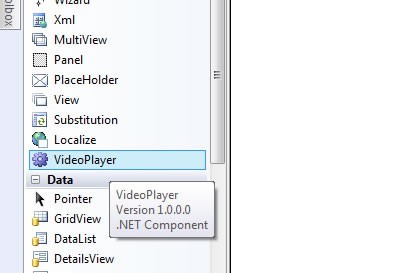
Step 11: Placing the VideoPlayer Control on ASP.NET Web Page
To see how the control works, we need to give it a home. Add a new page to the website. Right click the ASP.NET project from the Solution Explorer. Select Add New Item
, and add a Web Form
. Name the Web Form VideoPlayerTest.aspx
.
To place the control on the page, switch to Design
mode. Drag the VideoPlayer
control from the Toolbox and drop it onto the VideoPlayerTest.aspx
design view.
The following Listing shows how the control is declared on the page:
<cc1:VideoPlayer ID="VideoPlayer1" runat="server" Mp4Url="videos/movie.mp4" OggUrl="videos/movie.ogg" Width="400" Height="300" />
The following line of code is what Visual Studio added to the ASPX
file to accommodate the control. You can see it by selecting the Source
tab from the bottom of the code window in Visual Studio. The Register
directive tells the ASP.NET runtime where to find the custom control (which assembly) and maps it to a tag prefix.
<%@ Register assembly="CustomControls" namespace="CustomControls" tagprefix="cc1" %>
We can now test the control.
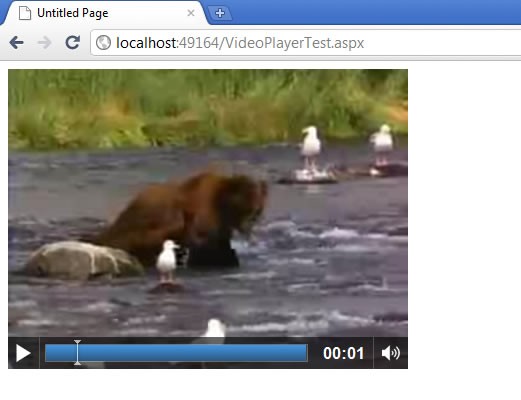
Summary
In this tutorial, you learned how to create your own ASP.NET custom server control from scratch. You now know every step of the process - from how to create a web custom control library project, how to add properties to a custom control, how to render the HTML markup of the control to the client, and, finally, how to use the ASP.NET custom control in a web form.
Hopefully, you now have the skills to create custom controls that have all the functionality of the standard ASP.NET server-side controls. Thank you so much for reading!
Comments