Twitter made several changes when they launched 1.1 of their API. One of the most notable changes is the introduction of authentication. That is, applications need to be authenticated before they are allowed to send requests to the API.
Authentication is powered by OAuth - an open protocol to allow secure authorization in a simple and standard method that allows users to approve application to act on their behalf without sharing their password.
In this tutorial, we will learn how to programmatically interact with Twitter's API as we develop a Twitter timeline WordPress widget that displays a list of latest tweets made by a Twitter user.
Here is a preview of the Twitter timeline widget to be built at the end of this tutorial.
To successfully send requests to the Twitter API, you need to create an application with OAuth authorization because unauthorized requests won't be allowed.
To create a Twitter application, you need to login to the Twitter developer dashboard using your Twitter account. The point of creating an application is to give yourself (and Twitter) a set of keys.
These include:
- the consumer key
- the consumer secret
- the access token
- the access token secret
Follow the steps below to create a Twitter application and to generate the keys.
- Login to Twitter developer account using your Twitter account and navigate to the Application Management console.
- Click on the Create New App button to initiate the Twitter application creation.
- Fill the form and click the submit button to create the application.
- Click on the application, navigate to the Permissions tab and change the Access level to Read and Write.
If you want to make any decent use of this API, you'll need to change your settings to Read and Write if you're doing anything other than standard data retrieval using GET requests.
To get your application consumer key and secret, navigate to the API Keys tab.
The API keys and API secret is the consumer key and consumer secret, respectively.
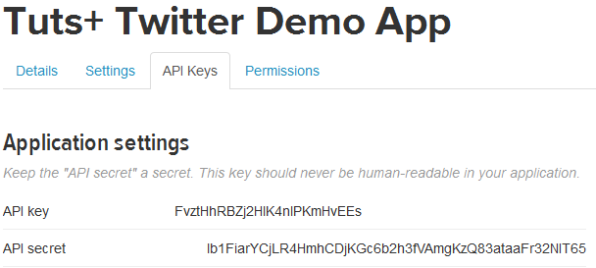
To get the application access token and access token secrets, still in the API Keys tab, scroll downward and click on the Create my access token button to create the access tokens.
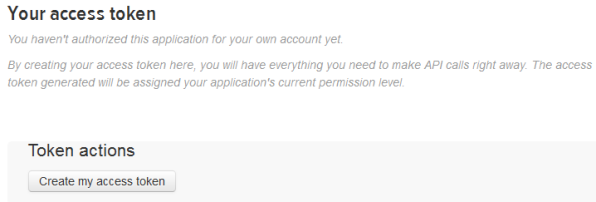
Refresh the page and your application Access tokens will be shown to you.
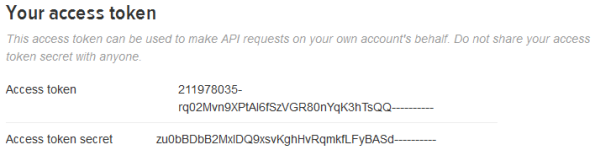
We now have the consumer key and secret and also the access token and secret keys. These OAuth credentials are authenticated by Twitter when sending requests to the API.
The widget settings of the Twitter timeline widget we are coding will consist of form fields that will collect and save these OAuth credentials to the database for reuse by the widget.
Let's get started coding the Twitter timeline widget plugin.
Twitter Timeline Widget Development
The header is first thing to go into the plugin file is the plugin header.
<?php /* Plugin Name: Twitter Tweets Widget Plugin URI: http://code.tutsplus.com Description: Displays latest tweets from Twitter. Author: Agbonghama Collins Author URI: http://tech4sky.com */
Create a class extending the WP_Widget
parent class.
class Twitter_Tweets_Widget extends WP_Widget { // ...
Give the widget a name and description via the __construct()
magic method.
function __construct() { parent::__construct( 'twitter-tweets-widget', __( 'Twitter Tweets Widget', 'twitter_tweets_widget' ), array( 'description' => __( 'Displays latest tweets from Twitter.', 'twitter_tweets_widget' ) ) ); }
The form()
method below create the widget settings form that will save the OAuth credentials to the database for reuse later by the widget.
public function form( $instance ) { if ( empty( $instance ) ) { $twitter_username = ''; $update_count = ''; $oauth_access_token = ''; $oauth_access_token_secret = ''; $consumer_key = ''; $consumer_secret = ''; $title = ''; } else { $twitter_username = $instance['twitter_username']; $update_count = isset( $instance['update_count'] ) ? $instance['update_count'] : 5; $oauth_access_token = $instance['oauth_access_token']; $oauth_access_token_secret = $instance['oauth_access_token_secret']; $consumer_key = $instance['consumer_key']; $consumer_secret = $instance['consumer_secret']; if ( isset( $instance['title'] ) ) { $title = $instance['title']; } else { $title = __( 'Twitter feed', 'twitter_tweets_widget' ); } } ?> <p> <label for="<?php echo $this->get_field_id( 'title' ); ?>"> <?php echo __( 'Title', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'title' ); ?>" name="<?php echo $this->get_field_name( 'title' ); ?>" type="text" value="<?php echo esc_attr( $title ); ?>" /> </p> <p> <label for="<?php echo $this->get_field_id( 'twitter_username' ); ?>"> <?php echo __( 'Twitter Username (without @)', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'twitter_username' ); ?>" name="<?php echo $this->get_field_name( 'twitter_username' ); ?>" type="text" value="<?php echo esc_attr( $twitter_username ); ?>" /> </p> <p> <label for="<?php echo $this->get_field_id( 'update_count' ); ?>"> <?php echo __( 'Number of Tweets to Display', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'update_count' ); ?>" name="<?php echo $this->get_field_name( 'update_count' ); ?>" type="number" value="<?php echo esc_attr( $update_count ); ?>" /> </p> <p> <label for="<?php echo $this->get_field_id( 'oauth_access_token' ); ?>"> <?php echo __( 'OAuth Access Token', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'oauth_access_token' ); ?>" name="<?php echo $this->get_field_name( 'oauth_access_token' ); ?>" type="text" value="<?php echo esc_attr( $oauth_access_token ); ?>" /> </p> <p> <label for="<?php echo $this->get_field_id( 'oauth_access_token_secret' ); ?>"> <?php echo __( 'OAuth Access Token Secret', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'oauth_access_token_secret' ); ?>" name="<?php echo $this->get_field_name( 'oauth_access_token_secret' ); ?>" type="text" value="<?php echo esc_attr( $oauth_access_token_secret ); ?>" /> </p> <p> <label for="<?php echo $this->get_field_id( 'consumer_key' ); ?>"> <?php echo __( 'Consumer Key', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'consumer_key' ); ?>" name="<?php echo $this->get_field_name( 'consumer_key' ); ?>" type="text" value="<?php echo esc_attr( $consumer_key ); ?>" /> </p> <p> <label for="<?php echo $this->get_field_id( 'consumer_secret' ); ?>"> <?php echo __( 'Consumer Secret', 'twitter_tweets_widget' ) . ':'; ?> </label> <input class="widefat" id="<?php echo $this->get_field_id( 'consumer_secret' ); ?>" name="<?php echo $this->get_field_name( 'consumer_secret' ); ?>" type="text" value="<?php echo esc_attr( $consumer_secret ); ?>" /> </p> <?php }
Below is a screenshot of the widget settings created by the form()
method above.
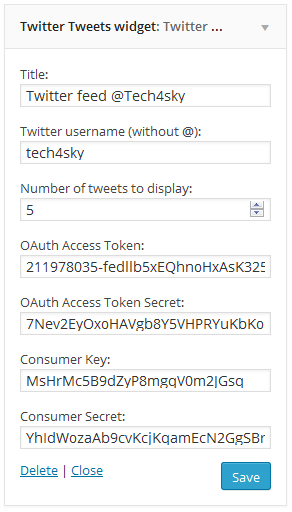
When values are entered into the settings form field, they need to be saved to the database. The update()
method sanitize the widget form values by stripping out malicious data and then save the sanitized data to the database.
public function update( $new_instance, $old_instance ) { $instance = array(); $instance['title'] = ( ! empty( $new_instance['title'] ) ) ? strip_tags( $new_instance['title'] ) : ''; $instance['title'] = ( ! empty( $new_instance['title'] ) ) ? strip_tags( $new_instance['title'] ) : ''; $instance['twitter_username'] = ( ! empty( $new_instance['twitter_username'] ) ) ? strip_tags( $new_instance['twitter_username'] ) : ''; $instance['update_count'] = ( ! empty( $new_instance['update_count'] ) ) ? strip_tags( $new_instance['update_count'] ) : ''; $instance['oauth_access_token'] = ( ! empty( $new_instance['oauth_access_token'] ) ) ? strip_tags( $new_instance['oauth_access_token'] ) : ''; $instance['oauth_access_token_secret'] = ( ! empty( $new_instance['oauth_access_token_secret'] ) ) ? strip_tags( $new_instance['oauth_access_token_secret'] ) : ''; $instance['consumer_key'] = ( ! empty( $new_instance['consumer_key'] ) ) ? strip_tags( $new_instance['consumer_key'] ) : ''; $instance['consumer_secret'] = ( ! empty( $new_instance['consumer_secret'] ) ) ? strip_tags( $new_instance['consumer_secret'] ) : ''; return $instance; }
I found a very useful Simple PHP Wrapper for Twitter API that make sending request and receiving response from the API painless which will be used by our widget.
Download the PHP wrapper in zip archive from it GitHub repo, extract and include the TwitterAPIExchange.php
file which contain the wrapper class.
The twitter_timeline()
method below accept the following as its arguments which comes in handy when making request to Twitter API.
-
$username:
Twitter username
-
$limit:
Number of tweets to be displayed by the widget
-
$oauth_access_token:
Twitter application OAuth access token.
-
$oauth_access_token_secret:
Application OAuth access token secrete.
-
$consumer_key:
Twitter application Consumer Key.
-
$consumer_secret:
Application Consumer secrete.
public function twitter_timeline( $username, $limit, $oauth_access_token, $oauth_access_token_secret, $consumer_key, $consumer_secret ) { require_once 'TwitterAPIExchange.php'; /** Set access tokens here - see: https://dev.twitter.com/apps/ */ $settings = array( 'oauth_access_token' => $oauth_access_token, 'oauth_access_token_secret' => $oauth_access_token_secret, 'consumer_key' => $consumer_key, 'consumer_secret' => $consumer_secret ); $url = 'https://api.twitter.com/1.1/statuses/user_timeline.json'; $getfield = '?screen_name=' . $username . '&count=' . $limit; $request_method = 'GET'; $twitter_instance = new TwitterAPIExchange( $settings ); $query = $twitter_instance ->setGetfield( $getfield ) ->buildOauth( $url, $request_method ) ->performRequest(); $timeline = json_decode($query); return $timeline; }
The method uses the PHP Wrapper for Twitter API to send request to the Twitter API, save and return the response (JSON data of the user timeline).
The time tweets were created or made is saved by the API in an English textual datetime. E.g. Thu Jun 26 08:47:24 +0000 2014
To make the tweet time more user-friendly, I created the method tweet_time()
that displays the time in the following ways.
- If the time is less than three seconds, it returns right now.
- Less than minute, returns x seconds ago.
- Less than two minutes, returns about 1 minute ago.
- Less than an hour, returns n minutes ago and so on.
Here is the code for the tweet_time()
method.
public function tweet_time( $time ) { // Get current timestamp. $now = strtotime( 'now' ); // Get timestamp when tweet created. $created = strtotime( $time ); // Get difference. $difference = $now - $created; // Calculate different time values. $minute = 60; $hour = $minute * 60; $day = $hour * 24; $week = $day * 7; if ( is_numeric( $difference ) && $difference > 0 ) { // If less than 3 seconds. if ( $difference < 3 ) { return __( 'right now', 'twitter_tweets_widget' ); } // If less than minute. if ( $difference < $minute ) { return floor( $difference ) . ' ' . __( 'seconds ago', 'twitter_tweets_widget' );; } // If less than 2 minutes. if ( $difference < $minute * 2 ) { return __( 'about 1 minute ago', 'twitter_tweets_widget' ); } // If less than hour. if ( $difference < $hour ) { return floor( $difference / $minute ) . ' ' . __( 'minutes ago', 'twitter_tweets_widget' ); } // If less than 2 hours. if ( $difference < $hour * 2 ) { return __( 'about 1 hour ago', 'twitter_tweets_widget' ); } // If less than day. if ( $difference < $day ) { return floor( $difference / $hour ) . ' ' . __( 'hours ago', 'twitter_tweets_widget' ); } // If more than day, but less than 2 days. if ( $difference > $day && $difference < $day * 2 ) { return __( 'yesterday', 'twitter_tweets_widget' );; } // If less than year. if ( $difference < $day * 365 ) { return floor( $difference / $day ) . ' ' . __( 'days ago', 'twitter_tweets_widget' ); } // Else return more than a year. return __( 'over a year ago', 'twitter_tweets_widget' ); } }
Next is the widget()
method that displays the Twitter timeline in WordPress front-end.
public function widget( $args, $instance ) { $title = apply_filters( 'widget_title', $instance['title'] ); $username = $instance['twitter_username']; $limit = $instance['update_count']; $oauth_access_token = $instance['oauth_access_token']; $oauth_access_token_secret = $instance['oauth_access_token_secret']; $consumer_key = $instance['consumer_key']; $consumer_secret = $instance['consumer_secret']; echo $args['before_widget']; if ( ! empty( $title ) ) { echo $args['before_title'] . $title . $args['after_title']; } // Get the tweets. $timelines = $this->twitter_timeline( $username, $limit, $oauth_access_token, $oauth_access_token_secret, $consumer_key, $consumer_secret ); if ( $timelines ) { // Add links to URL and username mention in tweets. $patterns = array( '@(https?://([-\w\.]+)+(:\d+)?(/([\w/_\.]*(\?\S+)?)?)?)@', '/@([A-Za-z0-9_]{1,15})/' ); $replace = array( '<a href="$1">$1</a>', '<a href="http://twitter.com/$1">@$1</a>' ); foreach ( $timelines as $timeline ) { $result = preg_replace( $patterns, $replace, $timeline->text ); echo '<div>'; echo $result . '<br/>'; echo $this->tweet_time( $timeline->created_at ); echo '</div>'; echo '<br/>'; } } else { _e( 'Error fetching feeds. Please verify the Twitter settings in the widget.', 'twitter_tweets_widget' ); } echo $args['after_widget']; }
The widget class Twitter_Tweets_Widget
is finally registered using the widgets_init
hook so it is recognizable by WordPress. Close out your class with a closing }
and then add the code below to initialize the
function register_twitter_widget() { register_widget( 'Twitter_Tweets_Widget' ); } add_action( 'widgets_init', 'register_twitter_widget' );
Finally, we are done coding the Twitter timeline widget.
Summary
In this article, we learned how to use the Twitter API in a real-world project in order to build our own Twitter timeline WordPress widget. Although the tutorial should be relatively straightforward, we covered topics such as OAuth, keys, and other topics that may be new to those of you working with an API.
If you have any questions, or suggestions for code improvements, let me know in the comments.
Comments