In this series, I will show you how to create a simple Hangman game for Android. In every tutorial, you'll learn a number of fundamental skills for creating Android applications. The application will consist of two screens, include user interaction involving adapters, contain dialogs and an action bar, and leverage XML for data storage. Let's get started.
Introduction
If you're a beginning Android developer with one or two basic applications under your belt, then you should be able to complete these tutorials without much difficulty.
The game will have a simple, basic structure so that you can add your own enhancements to it when you've completed the tutorials. Throughout this series, I will point out optional steps you can include to add additional functionality and to improve support for a wider range of Android devices. The user interface, for example, will be simple, but I encourage you to give it more love to make the game more appealing. The following screenshot should give you an idea of what the final result will look like.
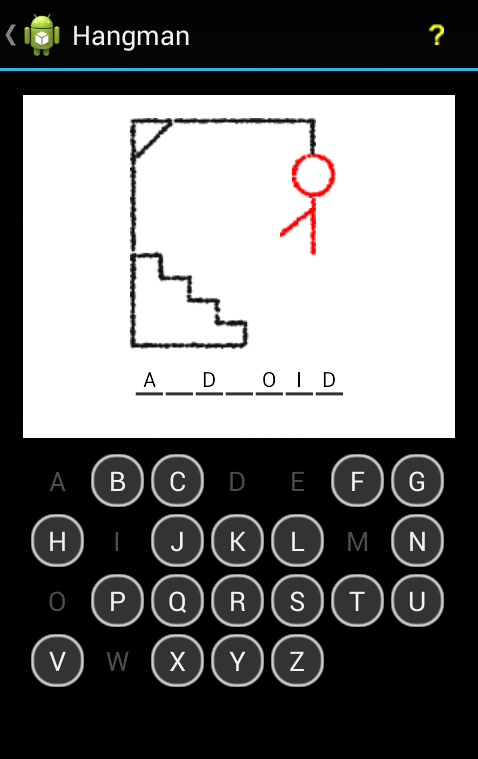
This tutorial is split up into three distinct parts.
- Project Setup
- User Interface
- Gameplay and Interaction
Our game will be a traditional Hangman game. It will choose a random word, presenting placeholders for each letter so that the user knows its length. The game will of course display a gallows area and buttons for each letter of the alphabet. Whenever the user taps a letter, the application will check whether the word includes the letter. If it does, the letter is revealed in the word. If the word doesn't contain the selected letter, one part of the body will be displayed in the gallows area, starting with the head. The user can make up to six wrong guesses and still win the game. However, if they make a seventh mistake, it's game over. If the player needs less than seven guesses, she wins the game. Are you still with me?
1. Create a New Android Project
Step 1
Create a new Android project in your IDE (Integrated Development Environment) of choice. In this tutorial, I will use Eclipse. In Eclipse, select New > Project from the File menu. In the Android section, select Android Application Project and click Next.
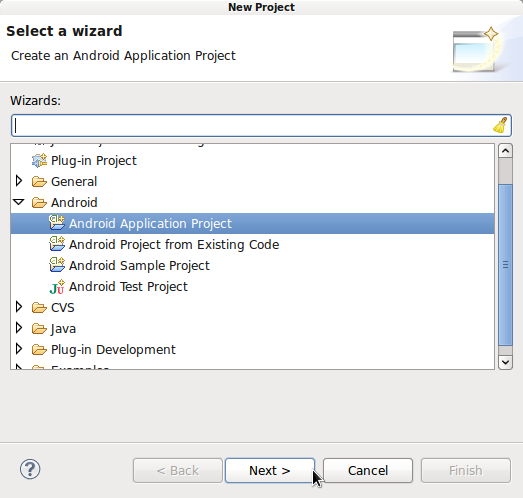
Enter an application, project, and package name. To keep things simple, the application targets API 16, but you may wish to support older versions. If you decide to do so, you'll need to carry out some additional processing. Click Next to continue.
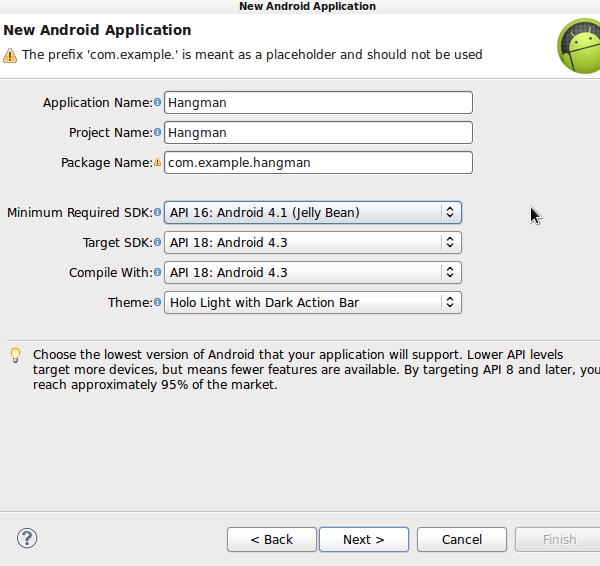
Step 2
In the Configure Project window, it is up to you to choose if you'd like to create a custom icon. However, make sure to check the Create activity and the Create Project in Workspace check boxes. Click Next.
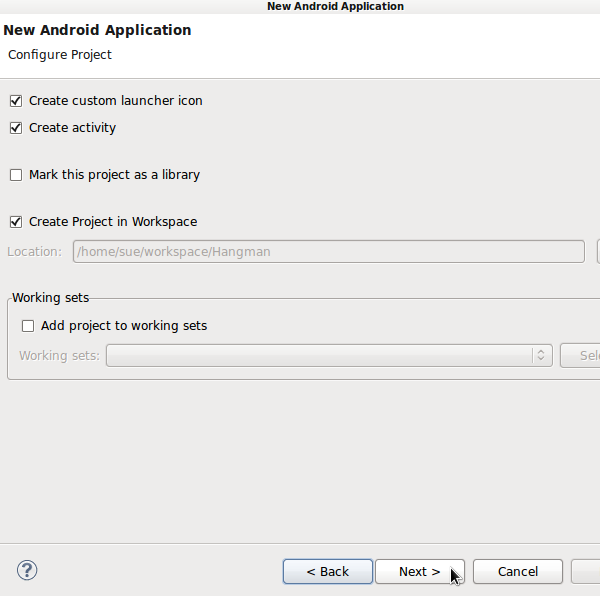
Set a launcher icon and click Next. In the Create Activity screen, select Blank Activity and click Next.
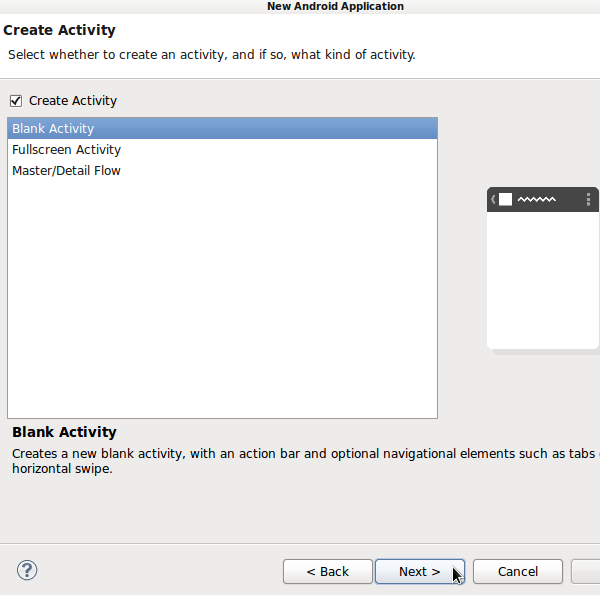
You can modify the activity class and layout names if you prefer, but the default names work just fine as well. Click Finish to create your new project.
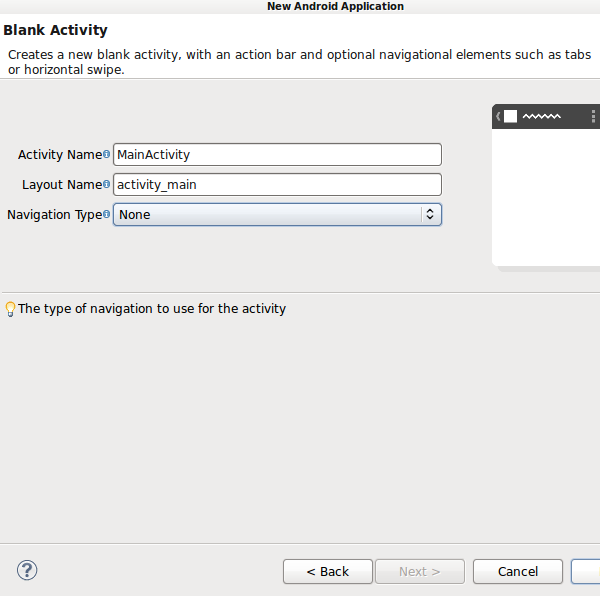
Now is a good time to configure the themes and styles associated with your application. To match the demo application, set the AppTheme
in your res/values/ styles XML file to android:Theme.Holo
. The default settings work fine, but your application's user interface will differ from the screenshots in this tutorial.
2. Create the User Interface Images
Step 1
Our application will present an intro screen with a play button to the user and a separate screen for the game itself. As you can see in the previous screenshot, the screen used for the game includes a number of images for the gallows and body parts. Beneath the gallows is the area for the word the user needs to guess and a grid with the letters of the alphabet. We will also use an action bar for navigating the game and it will also contain a help button.
We'll need quite a number of images for the gallows, the body parts (head, body, arms, and legs), the help button, and a background image for the intro screen. You can find these images in the source files of this tutorial, which you can find at the top of this tutorial. Keep in mind that you need to create additional images if you aim to target a broader set of devices with different screen sizes.
Download the images and add them to the drawable folders in your project directory. Make sure that you are using images suited for the various screen densities on Android.
Step 2
The application is also going to use some drawable resources that we can define as shapes in XML. These include the background for the letters in the word the user needs to guess and the background for the letters of the buttons (normal and highlighted state). These will also need to be tailored to the different screen densities that you want your application to support. The sample code of this tutorial gives you a good starting point.
Let's start with the background of the letters. Create a new file in your project drawables and name it letter_bg.xml.
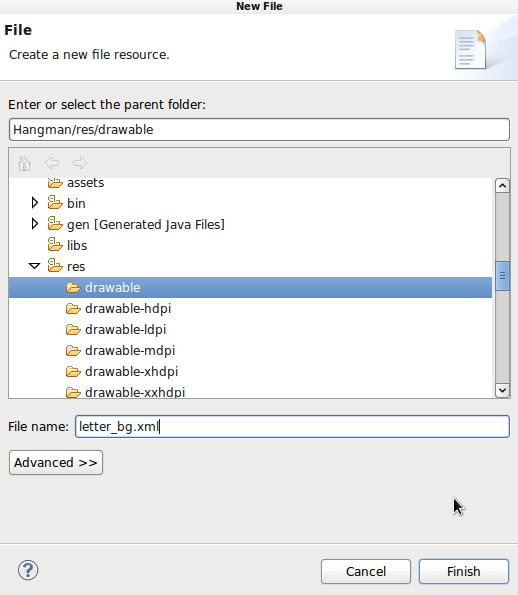
Enter the shape shown in the code snippet below.
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="line" > <stroke android:width="2dp" android:color="#FF333333" /> <solid android:color="#00000000" /> <padding android:bottom="20dp" /> <size android:height="20dp" android:width="20dp" /> </shape>
If you aren't familiar with creating shapes in XML, this may seem a little confusing. The result is an underline, which will be displayed as the background of each letter in the word the user needs to guess.
Next, create another file in your project's drawables for the letter buttons in their initial state and name it letter_up.xml. Add the following snippet to the file you've just created.
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:padding="5dp" android:shape="rectangle" > <solid android:color="#FF333333" /> <corners android:bottomLeftRadius="15dp" android:bottomRightRadius="15dp" android:topLeftRadius="15dp" android:topRightRadius="15dp" /> <stroke android:width="2dp" android:color="#FFCCCCCC" /> </shape>
Feel free to modify the above snippets to style the user interface elements to your liking. It is important to see the resources of this tutorial as a guideline. Create one more drawable and name it letter_down.xml. We'll use this drawable for the letter buttons in their highlighted state, that is, when the player taps the button.
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:padding="5dp" android:shape="rectangle" > <solid android:color="#FF000000" /> <corners android:bottomLeftRadius="15dp" android:bottomRightRadius="15dp" android:topLeftRadius="15dp" android:topRightRadius="15dp" /> </shape>
The user can choose only one letter in each round of the game. This means that each letter or button is disabled from the moment the user has chosen the letter. This is reflected by updating the button's background.
3. Create the Main Layout
Step 1
Let's create the main layout file for the intro screen. Open the main layout file of the project and update its contents to reflect the snippet below.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#FF000000" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" > <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:src="@drawable/android_hangman_bg" /> <Button android:id="@+id/playBtn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:text="Play" android:textColor="#FFFF0000" /> </RelativeLayout>
As you can see, the code refers to a drawable resource to display in the background. You can omit the ImageView
element if you like. If you are including the image, make sure the filename matches this code. The layout includes a single button to play the game, but you can add other buttons later if you like. For example, you could add levels or categories to the application rather than simply choosing a random word from a list as we will do in this tutorial.
Step 2
Let's create a few more files that we'll use in the next tutorials. First, add a second activity class to the project for the game itself. Select the package in your project's src directory and choose New > Class from the File menu. Name the class GameActivity and set android.app.Activity
as its superclass.
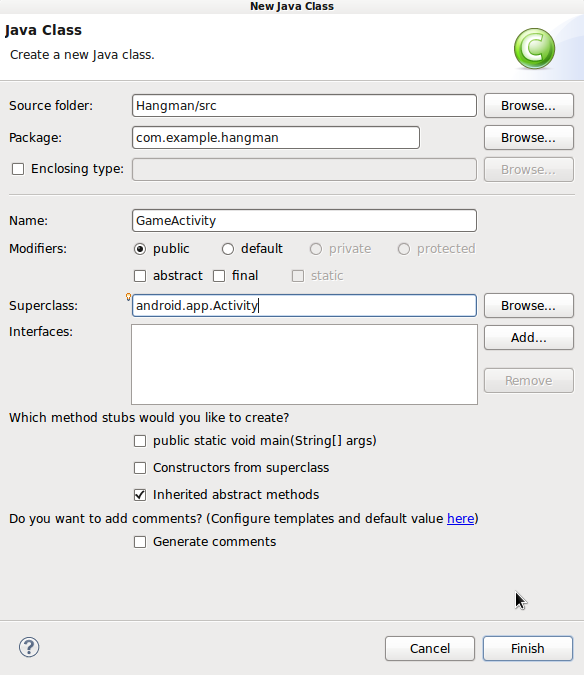
We will complete the GameActivity
class later in this series. Let's now create a layout file for the class. Add a new file to the project's layout folder and name it activity_game.xml. Replace its contents with the following snippet below.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="fill_parent" android:background="#FF000000" android:orientation="vertical" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" > </LinearLayout>
We will add items to the layout next time. Add the activity
to your project manifest file, after the element for the main activity
, inside the application
element:
<activity android:name="com.example.hangman.GameActivity" android:parentActivityName="com.example.hangman.MainActivity" android:screenOrientation="portrait" />
We set the screen's orientation to portrait. If you decide to also support a landscape orientation in your game, then you need to remove the orientation attribute. We also specify the main activity as parent, which means that the action bar can be used for navigating the game. We will talk more about this a bit later in this tutorial.
4. Start the Game Activity
Step 1
Let's finish this tutorial by starting the gameplay activity when the user taps the play button. Open the file of the MainActivity
class and begin by adding the following import statements.
import android.content.Intent; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button;
Eclipse should have pre-populated the MainActivity
class with an Activity
class outline, including an onCreate
method in which the content view is set. After the setContentView
line, retrieve a reference to the play button you added to the main layout and set the class to listen for clicks.
Button playBtn = (Button)findViewById(R.id.playBtn); playBtn.setOnClickListener(this);
We can find the button by using the identifier we set in the layout XML. Extend the opening line of the class declaration to implement the click listening interface as follows.
public class MainActivity extends Activity implements OnClickListener
Step 2
Add the onClick
method after the onCreate
method as shown below.
@Override public void onClick(View view) { //handle clicks }
Inside this method, we check if the play button was clicked and, if it was, start the gameplay activity.
if (view.getId() == R.id.playBtn) { Intent playIntent = new Intent(this, GameActivity.class); this.startActivity(playIntent); }
This won't have much of an effect at the moment since we haven't implemented the gameplay activity yet. That is something we'll do in the next tutorial.
Conclusion
In this tutorial, we've created the foundation for the Hangman application. In the next tutorial, we will build the game's interface elements and, in the final part, we will focus on the interactive aspects of the application. Along the way, we will explore adapters and the action bar. We will also store the word answers in XML, retrieving them when the app launches, and choosing one at random every time the user plays a game.
Comments