In the first two articles of this series, I wrote about what welcome pages are and how they are helping products improve user experience by connecting the dots, after which I wrote about the WordPress Transients API that I intend to use while building the welcome page.
Coding a welcome page for your WordPress plugin can be a tricky process. The entire concept revolves around redirecting users to a particular page via setting transients and finally deleting them. Let's start building the welcome page.
WP Welcome Page Boilerplate
I am going to build a welcome page boilerplate for WordPress in the form of a plugin that could be used within your WordPress product. The final form of this plugin is hosted at GitHub at WP-Welcome-Page-Boilerplate-For-TutsPlus.
At this point, I’m assuming that you have the following set up:
- a local machine or a web server
- a demo WordPress website dashboard
Plugin Architecture
I'm going to create a simple plugin that displays a welcome page when a user installs and activates the plugin. Let's discuss the plugin architecture:
- WP-Welcome-Page-Boilerplate: Folder that contains our plugin.
- WP-Welcome-Page-Boilerplate/welcome: Folder for the welcome page related stuff.
-
WP-Welcome-Page-Boilerplate/welcome/img: Folder for images.
- wp-welcome-page-boilerplate.php: The main plugin file in the root. This file is responsible for defining the global constants and requiring the initializer file, i.e. welcome-init.php.
-
welcome-init.php: The initializer file which is responsible for three things: adding a transient when plugin gets activated, deleting it when the plugin gets deactivated, and finally adding the logic file, i.e. welcome-logic.php.
-
welcome-logic.php: The logic file which is responsible for safe welcome page redirection, creating the welcome page sub-menu, and adding the welcome page view file, i.e. welcome-view.php.
- welcome-view.php: The view file which is responsible for displaying the welcome page and is built with PHP and HTML.
- There are optional folders for images and CSS, and they are named in accordance with their purpose.
You can view the following screenshot to verify the architecture.
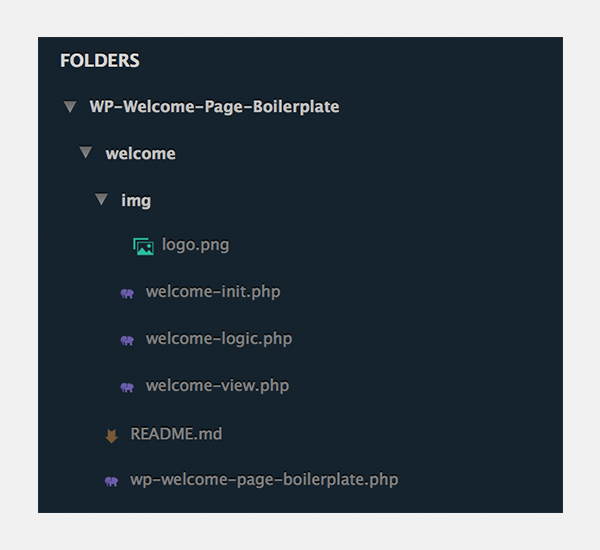
Plugin Workflow
The plugin works in the following way:
- Adds a transient on plugin activation.
- Deletes a transient on plugin deactivation.
- Safe redirect to the welcome page.
Standard Plugin Base File
Let's commence our discussion with the contents of the base file, which is wp-welcome-page-boilerplate.php
. Here is the complete code:
<?php /** * Plugin Name: WP Welcome Page Boilerplate * Plugin URI: http://code.tutsplus.com/articles/building-a-welcome-page-for-your-wordpress-product-introduction--cms-26013 * Description: Welcome page boilerplate for WordPress plugins. * Version: 1.0.0 * Author: mrahmadawais, WPTie * Author URI: http://AhmadAwais.com/ * License: GPL-3.0+ * License URI: http://www.gnu.org/licenses/gpl-3.0.html * Domain Path: /lang * Text Domain: WPW */ if ( ! defined( 'WPINC' ) ) { die; }
The PHPDoc header section of the plugin tells WordPress that a file is a plugin. The defined parameters tell how the data is handled. At a minimum, a header can contain just a Plugin Name, but several pieces can—and usually should—be included. You can read about the header requirements in the WordPress Plugin Developer Handbook.
The next set of code performs a security check. If anyone tries to access this file directly, it runs the ABSPATH
check, which terminates the script if accessed from outside WordPress.
After that, the code looks like this:
// Plugin version. if ( ! defined( 'WPW_VERSION' ) ) { define( 'WPW_VERSION', '1.0.0' ); } // Plugin folder name. if ( ! defined( 'WPW_NAME' ) ) { define( 'WPW_NAME', trim( dirname( plugin_basename( __FILE__ ) ), '/' ) ); } // Plugin directory, including the folder. if ( ! defined( 'WPW_DIR' ) ) { define( 'WPW_DIR', WP_PLUGIN_DIR . '/' . WPW_NAME ); } // Plugin url, including the folder. if ( ! defined( 'WPW_URL' ) ) { define( 'WPW_URL', WP_PLUGIN_URL . '/' . WPW_NAME ); } // Plugin root file. if ( ! defined( 'WPW_PLUGIN_FILE' ) ) { define( 'WPW_PLUGIN_FILE', __FILE__ ); }
I have defined a few global constants which define details about the plugin's version, root folder, URL, and the plugin's main file.
Each of these contains an if( ! defined() )
statement, which helps avoid any errors due to the redefinition of a global constant. I recommend defining the global constants with your package name as a prefix. The package name in this plugin is WPW
, i.e. WordPress Welcome. So, every constant has a prefix of WPW_
.
The defined plugin constants are:
-
WPW_VERSION
: Plugin Version -
WPW_NAME
: Plugin Folder Name -
WPW_DIR
: Plugin Directory -
WPW_URL
: Plugin URL -
WPW_PLUGIN_FILE
: Plugin Root File
Once all these constants are defined, we'll begin with the contents of our welcome file.
Finally, I required the welcome initializer and the welcome logic.
if ( file_exists( WPW_DIR . '/welcome/welcome-init.php' ) ) { require_once( WPW_DIR . '/welcome/welcome-init.php' ); } if ( file_exists( WPW_DIR . '/welcome/welcome-logic.php' ) ) { require_once( WPW_DIR . '/welcome/welcome-logic.php' ); }
I like keeping my code minimal, precise, and well documented. So, instead of adding every code block in a single file, I prefer making individual files, each with one purpose only. That is why we have an initializer file which will initialize everything related to the welcome page.
The require_once()
statement requires the welcome-init.php
file, but before requiring a file, I always check it with the file_exists()
PHP function to avoid any fatal errors in case the file gets deleted.
The same principles apply for the welcome logic, which we'll get to later in the next part of the series.
You can check the final code of the wp-welcome-page-boilerplate.php
file on GitHub.
Welcome Initializer
To manage all the welcome page related files, I created a separate folder named welcome and added a welcome-init.php
file. The entire code of this file controls the initialization of the welcome page.
Let's study the complete code of this file:
<?php /** * Welcome Page Init * * Welcome page initializer. * * @since 1.0.0 */ if ( ! defined( 'WPINC' ) ) { die; } /** * Activates the welcome page. * * Adds transient to manage the welcome page. * * @since 1.0.0 */ function wpw_welcome_activate() { // Transient max age is 60 seconds. set_transient( '_welcome_redirect_wpw', true, 60 ); } register_activation_hook( WPW_PLUGIN_FILE, 'wpw_welcome_activate' ); /** * Deactivates welcome page * * Deletes the welcome page transient. * * @since 1.0.0 */ function wpw_welcome_deactivate() { delete_transient( '_welcome_redirect_wpw' ); } register_deactivation_hook( WPW_PLUGIN_FILE, 'wpw_welcome_deactivate' );
The code begins with inline documentation about the file and an ABSPATH
check. Now I needed a way to add and delete the transient when the plugin is activated and deactivated respectively.
Luckily, WordPress provides us with two hooks for this exact purpose.
-
register_activation_hook( string $file, callable $function )
: This hook is fired when the plugin gets activated. It takes the plugin's main $file and a callable $function as parameters. -
register_deactivation_hook( string $file, callable $function )
: This hook is fired when the plugin gets deactivated. It takes the plugin main $file and a callable $function as parameters.
So, now we need to make use of these hooks. I created a transient for the welcome page. The set_transient()
function is called inside the custom wpw_welcome_activate()
function. In the previous article, we learned that the set
operation takes a key, value and an expiration time as its parameters.
Therefore, the key _welcome_redirect_wpw
is set to a value true
for 60
seconds. This defines the maximum age after which the transient expires.
Next is the activation hook, and as we know this hook runs only when a plugin is activated. It adds our transient to the database. Once the transient is in the database, it means we can check it and redirect the user to our welcome page. How to redirect the user? That's what we will study in the next article.
After that, I wrote the code which runs upon deactivation of the plugin. We want a functionality where the transient gets deleted when a user deactivates the plugin. To do so, I called the delete_transient()
function, which takes the key _welcome_redirect_wpw
as its parameter. Then there is the wpw_welcome_deactivate()
function, which is added to the plugin deactivation hook.
This part is optional, but I want my users to see the welcome page whenever they activate the plugin. If you don't, you can most definitely ignore the deletion of your transient.
So far, I have discussed the part of the code which is necessary to create and delete a transient. But the code for welcome logic is still missing, which would redirect the users to the welcome page. We'll tackle that next.
Conclusion
This is it for today. So, we are halfway through. The base file and the initializer for our welcome page are ready. Now we need to create the logic and view files, which is the plan for the next article.
Finally, you can catch all of my courses and tutorials on my profile page, and you can follow me on my blog and/or reach out on Twitter @mrahmadawais where I write about development workflows in the context of WordPress.
As usual, don't hesitate to leave any questions or comments below, and I'll aim to respond to each of them.
Comments