This tutorial will teach you how to implement an options menu in any of your Android SDK applications. Read on!
In Android apps, you can make use of three standard menus supported within the platform: the context menu, the options menu, and submenus. The options menu appears when the user presses the menu button on their Android device. This is a common feature in almost all apps, so your users will be used to the menu appearing in this way. The options menu is typically used for providing additional info about an app, as well as linking to a help and settings sections. To implement an options menu for an Activity in an Android app, a few fairly straightforward steps are required.
Step 1: Open an Activity Class
The options menu you create will work with one or more Activity classes, so choose an Activity and open it in Eclipse. If your app only has one Activity class as its main screen, you can use it. If you want to create a new Activity for the purposes of this tutorial, feel free to do so. Select your application package and choose "File", "New", then "Class" and enter a name of your choice. Remember to make your class extend the Activity class and add it to the application Manifest.
Step 2: Create a Resources Folder
If you look at your application project in the Eclipse Package Explorer, you will see the various files and directories within it. The "res" folder holds all of your application resources. To create a menu, you need a menu folder, so create one inside the "res" folder by selecting it and choosing "File", "New", then "Folder" and entering "menu" as the name.
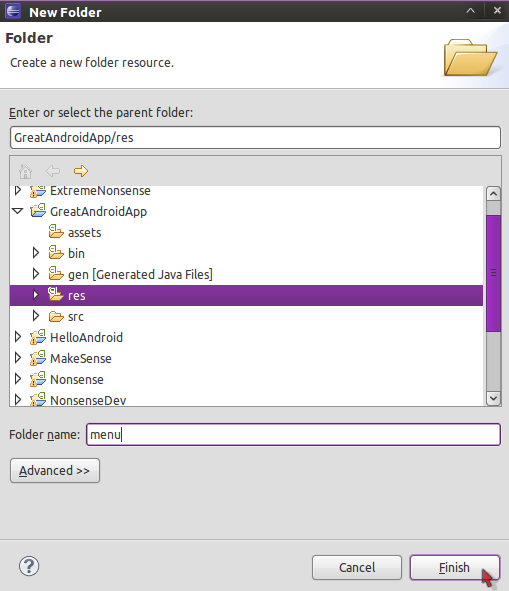
Your new folder will appear within the "res" directory:
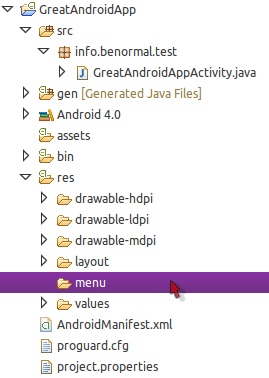
Step 3: Create a Menu XML File
Your options menu will be defined as an XML file inside the new menu folder you created. Choose the folder and create a new file by selecting "File", "New", then "File" and entering a name. You can choose any filename you like, for example "my_options_menu.xml". Eclipse will display error messages when you first create the menu file, but don't worry, these will disappear as you build it.
Open your new XML file and enter the following outline:
<menu xmlns:android="http://schemas.android.com/apk/res/android"> </menu>
Between these opening and closing tags, you will place XML markup for each item in your options menu.
Step 4: Add Items to Your Menu
You can add one or more items to your options menu depending on the needs of your own project. Add an item for each menu option using the following syntax:
<item android:id="@+id/about" android:title="About" /> <item android:id="@+id/help" android:title="Help" />
This defines two menu items, one for an "About" section and one for "Help" information. Each menu has an ID attribute and a title. The ID attribute allows your application code to refer to the item and the title defines the text users will see when the menu appears.
Step 5: Create Icons for Your Menu Items
You do not need to create icons for your options menu items, but you can do so if you wish. Use a graphic design or image editing program of your choice to create your icons as PNG files and place them in your application drawable folders. By default, Eclipse creates three drawable folders, for low, medium, and high resolution user devices. You can copy image files into these folders by browsing to your workspace, then locating your Android project directory and finding the "res/drawable" folders from there.
The official Android guidelines recommend a maximum of 72px squared for high resolution, 48px for medium and 36px for low. Once you have your icons in their folders, you can alter your menu item XML to include them as follows:
<item android:id="@+id/about" android:icon="@drawable/about" android:title="About" /> <item android:id="@+id/help" android:icon="@drawable/help" android:title="Help" />
Each drawable reference uses the filename minus its extension.
Step 6: Inflate Your Menu Resource
To instruct Android to use your options menu, open the Activity class you want it to appear with. Add the following method to your Java code, inside the class declaration and after the "onCreate" method:
public boolean onCreateOptionsMenu(Menu menu) { MenuInflater inflater = getMenuInflater(); inflater.inflate(R.menu.my_options_menu, menu); return true; }
Alter the "my_options_menu" section if you saved your menu XML file with a different name.
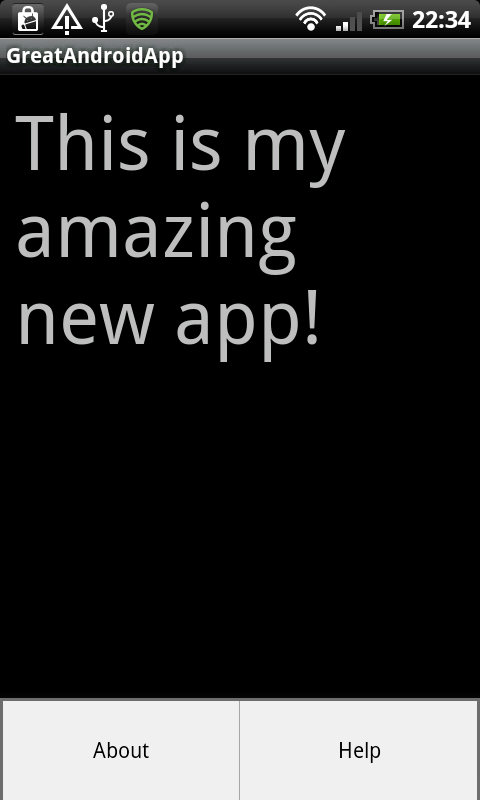
Step 7: Detect User Interaction
To respond to user interaction with your menu, you first need to detect it within your Activity class. Add the following method outline after the "onCreateOptionsMenu" method:
public boolean onOptionsItemSelected(MenuItem item) { //respond to menu item selection }
Inside this method, which returns a boolean value, you can add code to respond to each particular item. The system will automatically call the "onOptionsItemSelected" method when the user chooses any of the options menu items.
Step 8: Respond to Menu Item Selection
Before your code can respond appropriately to user interaction with the menu, you need to work out which item was selected. Add a switch statement to your method using the following sample syntax:
switch (item.getItemId()) { case R.id.about: startActivity(new Intent(this, About.class)); return true; case R.id.help: startActivity(new Intent(this, Help.class)); return true; default: return super.onOptionsItemSelected(item); }
Add a case statement for each item in your menu. This sample code starts new Activity screens for each item chosen. If you opt to do this, you will need to add an Activity class for each option in your application Java code as well as in the Manifest file.
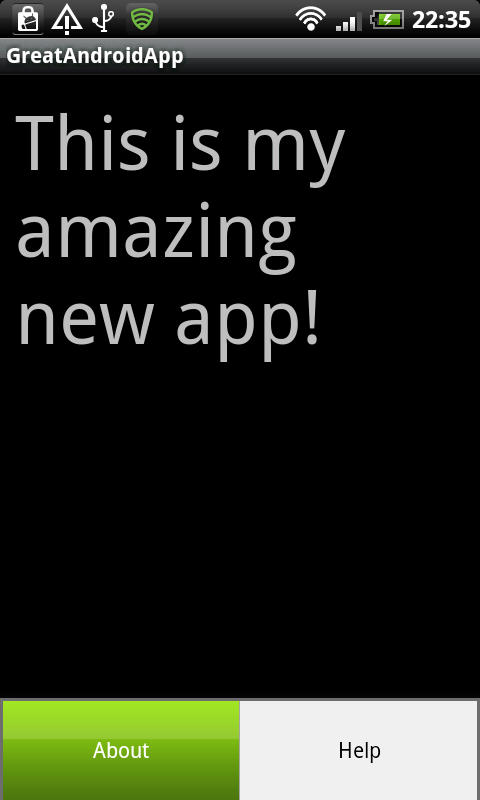
Conclusion
Your Activity should now display the options menu when users press their device menu button. The complete Activity code follows, for an options menu used with the main Activity class:
import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; public class GreatAndroidAppActivity extends Activity { public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); } public boolean onCreateOptionsMenu(Menu menu) { MenuInflater inflater = getMenuInflater(); inflater.inflate(R.menu.my_options_menu, menu); return true; } public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case R.id.about: startActivity(new Intent(this, About.class)); return true; case R.id.help: startActivity(new Intent(this, Help.class)); return true; default: return super.onOptionsItemSelected(item); } } }
Eclipse typically adds the import statements automatically as you enter your Java code.
As with any development project, your apps will be more usable if they exploit the type of interaction and functionality users expect as standard. Using the options menu is a good way to achieve this when providing informative sections.
Comments