In this series we are learning about Android development. So far we installed the development tools and became acquainted with them. In this tutorial, we will explore the structure and content of an Android project, using the simple project we created previously. We will look at the source directory and the project resources. We will also begin looking briefly at the project manifest.
Introduction
This tutorial will primarily involve exploration, but we will begin development tasks in the next few tutorials, when we look at building user interfaces, responding to user interaction, and using Java to code application logic. We will focus on the project ingredients that you are most likely to interact with when you start to develop for Android, but you will find other elements already inside your application structure and many more are possible. We will not go into too much detail about any particular element in this tutorial, but will get to know some of the basic building blocks of an Android app.
1. Source
Step 1
Open Eclipse and expand the Package Explorer folder for the project we created. Inside the "src" folder you should see the package you named when you set the project up. Inside the package should be your Activity class file, which should also be open in the editor. The source folder holds all of the Java files you work on when you develop Android apps.
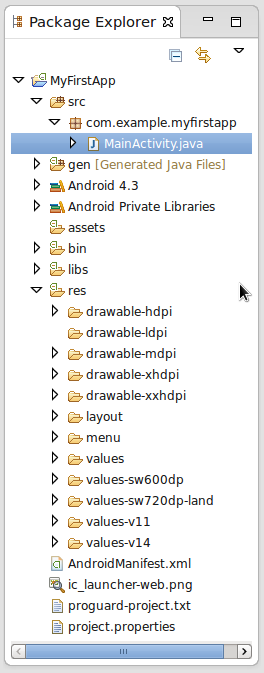
Each time you create a project you will create a package with your Java class files in it. An app may have more than one package in it and each package may hold multiple class files. The class files contain the processing code that presents your app to the user, responds to user interaction, and carries out any necessary processing. Essentially, the class files divide up the code involved in the application according to the Object Oriented conceptual model.
We will cover more about Java concepts and practices later in this series. For now, just understand that a Java application splits the various processing tasks between a number of objects. Each object is defined by a class declaration, which is typically a single file in an application, but which can also be nested inside another class file. An object is basically a chunk of code which carries out some part of the functionality involved in the app. The code in a class file can refer to the other classes in the application or in any package within the application.
When you start developing apps, you need to add Java classes to your package(s) in the source folder. A typical Android app that presents a user interface to the user will have at least one Activity file in it, with extra Activity classes for each screen in the app. There are other types of app such as those involving widgets and services, which can adopt a different structure. It's best to learn about the Activity-focused UI type of app to begin with and learn about the others later.
Step 2
Have a look at the Activity class file in your new app. We will explore more of the Activity code later on in this series, so don't worry too much about the details. This is your app's main Activity, which starts when the app launches. Your app may then launch other Activities on user interaction. When you created your project, Eclipse sets the app up to use this as the main class. It is listed as the main Activity in the project Manifest, which we will look at soon.
Inside the main Activity class, you will see the onCreate method, which contains the code that will execute when the Activity is created, i.e. when the app is launched. Inside the method you will see the following line of code:
setContentView(R.layout.activity_main);
This line specifies the layout file we created when we started the project, telling Android to use it as content view. This means that whatever is in the layout file will be what users see when this Activity is on the screen.
We will look more at this later but for the moment notice the "R.layout.activity_main" syntax. This is how your Java code refers to the resources in the app. We will use similar syntax to refer to resources by their ID value, as well as referring to other types of resources such as images and data values. The "R" represents the app resources and what follows specifies the item type, in this case a layout, stored inside the "res/layout" directory. The resource is finally identified using its name - in the case of the layout this is the filename. The syntax is therefore "R.type.name". You will get used to this when we start to code.
Later in this series we will add code to the Activity class file to handle user interaction. Expand your app "res" folder now. Inside it you will see a number of sub-folders. These are the folders Eclipse and ADT create by default when you start a new Android project, but there are a number of other possible directories you can also add for different types of resources.
2. Layout Resources
As we have already seen, the layout file formed when the project was created appears in the "res/layout" folder. If an app has multiple Activity screens, it will typically have a layout file for each. You may also use layout files for individual UI items. When you create the class file for an Activity, you set the layout using setContentView as we saw above. You can alternatively define a layout in Java code, in which case it is built dynamically when the app executes. However, the advantage to using XML is that you can see a visual representation of the layout while you design it.
Inside the main layout file for your app, which should be open in the editor, you will see XML structures. Don't worry if you have no XML experience, we will run over the basics later on in this series. For now, just understand that XML is a markup language, similar to HTML if you have tried Web development before. XML files model data within a tree structure. Typically a layout file has a root layout element modeling a particular type of layout, with child elements inside it for the UI items such as buttons, images, and text.
3. Drawable Resources
You will see multiple folders in the resources directory with "drawable" in the name. These store the image files your app uses. These image files can be digital image files you prepare outside Eclipse, with formats such as PNG or JPEG. Alternatively, you can define certain drawables using XML code to describe shapes, color, and appearance. Once you have a file in your drawable folders you can refer to it in the app layout files or in Java code. This allows you to build images into your app's UI.
The resource directory includes drawable folders for each density bucket. The density buckets are generalized categories for the the different screen densities on devices running Android. The generalized categories are for low, medium, high, extra high, and extra extra high density. Using these allows you to simplify the process of supporting multiple screen densities by catering for each of these categories. This means that when you include image files in your projects, you can include versions of them in each density folder, tailoring the images to the densities in each case.
4. Data Resources
In your "res" directory you will see some folders with "values" in the title. These are for data values you wish to use within your app. Such values can include text strings and numbers. The values folders contain XML files in which one or more values are listed. Each listing includes a name and the value in question. Other files in the app, such as Java class or layout files, can refer to the values using their names. A typical use for such a value would be to store a text string to display within a UI element such as a button.
The different values files in the app are designed to allow you to tailor values to particular screen sizes and API levels. If the same value can be used across devices, it can be saved in the plain "values" folder.
5. The Manifest
If you look in the main folder for your app, you will see the project Manifest file. Open it in the editor by double-clicking it. You will see a graphical interface to its content. Click the "AndroidManifest.xml" tab at the bottom of the editor window to see the XML code. This file defines multiple aspects of the app as a whole. Eclipse and ADT build certain elements into the Manifest when you create the app, basing these on the settings you chose during project creation. You can add other elements to the Manifest manually. For example, if you add other Activities to your app.
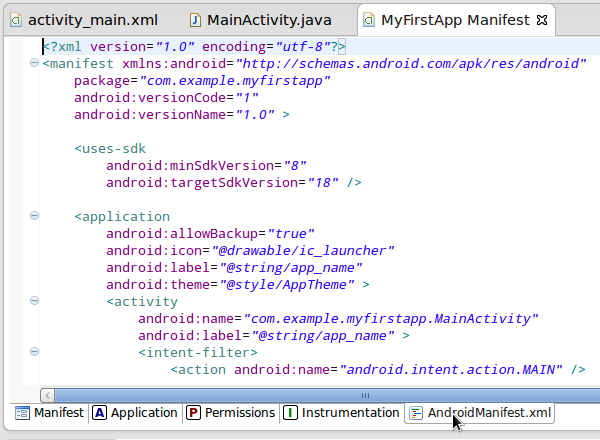
We will run through some of the main elements to understand the Manifest, but there are many other elements you can include. Listed in the Manifest for your new app project you will see the uses-sdk element, in which we indicate minimum and target API levels. The application element contains attributes indicating the launcher and app name. Inside the application element is an activity element, listed as the main Activity to launch when the app runs via the intent-filter element. When you add new Activities to an app you will include a new activity element for each.
Other elements you may need to add to the Manifest include the uses-permission element in which you list permissions the app requires - the user sees a list of these before installing the app. Permissions include actions such as fetching data over the Internet, writing to storage, or accessing other features of the device such as the camera. The Manifest also lists data regarding which devices the app supports, as well as lists other app components such as background services.
6. Other Files
So far we have covered the main aspects of an Android app project structure that you need to know for your first few apps. We will work with these files as we learn the skills involved in Android development. There are several other files and directories in the project as you can see in Eclipse, but for the most part you can ignore them for now.
As we saw above, you can refer to resources using the "R." syntax. Eclipse and the ADT manage the system that refers to the resources in your app from Java. When you add or edit the resources in your project, Eclipse writes to the "R.java" file, which in turn allows you to refer to the resources using "R.". When you start working in your Java files, you will see that Eclipse will prompt you with suggestions when you refer to R, making it easier to manage the resources in your app.
The "R.java" file is stored in the "gen" folder. Do not attempt to edit this file directly, it automatically generates when you edit the resources in your project. The system manages this process by allocating a unique integer ID to each resource in your app.
Conclusion
In this tutorial we explored the basics of an Android project structure. Feel free to spend more time exploring the other files and folders in your project to get to know its overall structure. In the following sections of this series we will build user interface elements and handle user interaction with our app. We will also look at some of the essential features of Java programming to understand it in order to get the best out of our Android development project.
Comments