Aside from a handful of edge cases, every application that you build has some form of user interface. On Android, this is accomplished through the use of View
and ViewGroup
objects. In this article, you learn about some of the more commonly used View
components available for displaying content and you are introduced to how they are used.
Do you find it easier to learn with video? Why not check out our course:
1. Views
View
objects are used specifically for drawing content onto the screen of an Android device. While you can instantiate a View
in your Java code, the easiest way to use them is through an XML layout file. An example of this can be seen when you create an simple "Hello World" application in Android Studio. The layout file is the one named activity_main.xml, looking something like this.
<TextView android:id="@+id/hello_world" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" />
The above example shows a type of View
that will be displayed on the screen. The layout_width
and layout_height
attributes state that View
should only take up as much room on the screen as needed to display the text "Hello World!". The id
attribute is how that View
can be referenced in your Java code like this:
setContentView(R.layout.activity_main); TextView textView = (TextView) findViewById(R.id.hello_world);
While you can set attributes for a View
in XML, you can also change attributes in your Java code, such as changing the text of the above TextView
.
textView.setText("This is a changed View");
The above code updates the text of the TextView
to "This is a changed View". This is what that looks like when you run the app.
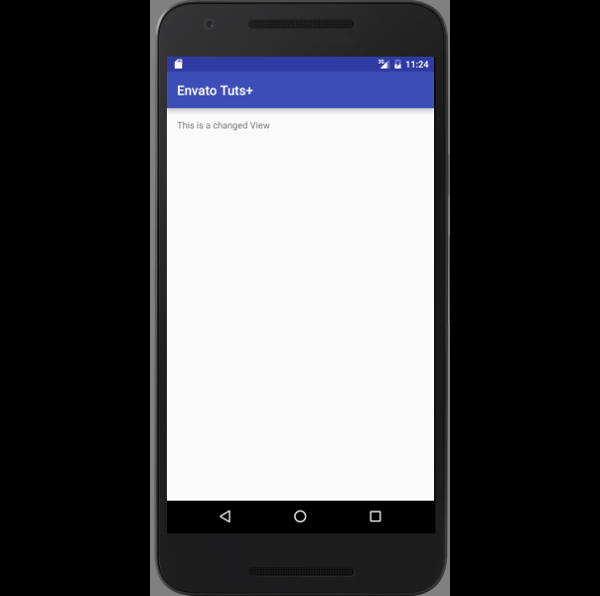
Now that you know how to work with basic View
objects, let's go over some of the different types that are available to you in the Android SDK.
Display Views
TextView
This is the View
we used in the above example. The main job of the TextView
is to display text on the screen of an Android app. While this may seem like a simple task, the TextView
class contains complex logic that allows it to display marked up text, hyperlinks, phone numbers, emails, and other useful functionality.
ImageView
As the name implies, the ImageView
is designed specifically to display images on the screen. This can be used for displaying resources stored in the app or for displaying images that are downloaded from the internet.
Input and Controls
Some View
objects are used for more than just displaying content to users. Sometimes you need to receive some sort of input to control your applications. Luckily, Android provides several View
subclasses specifically for this purpose.
Button
The Button
class is one of the most basic controls available in an app. It listens for clicks from the user and calls a method in your code so that you can respond appropriately.
Switch
and CheckBox
The Switch
and CheckBox
classes have an active and inactive state that can be toggled between. This is useful for changing settings in an app. Compatible versions with Material Design are available through the AppCompat support library.
EditText
This View
subclass is an extension of the TextView
class and allows users to update the contained text via a keyboard input.
Adapter Based Views
While each of the above View
examples are single items, sometimes you want to display a collection of items. These View
classes require the use of an Adapter
to handle displaying the proper user interface per item in a collection.
ListView
The ListView
class is used for displaying a collection of items in a linear, single-column, scrollable View
. Each individual item can be selected for displaying more details or performing an action related to that item.
GridView
Similar to the ListView
class, the GridView
class takes an Adapter
and displays items in multiple columns on the screen.
Spinner
The final collection View
class you learn about in this tutorial is the Spinner
class. It accepts an Adapter
and displays items in a dropdown menu when the Spinner
is clicked so that an item can be selected by the user.
2. View Groups
A ViewGroup
is an invisible object used to contain other View
and ViewGroup
objects in order to organize and control the layout of a screen. ViewGroup
objects are used for creating a hierarchy of View
objects (see below) so that you can create more complex layouts. That said, the more simple you can keep a layout, the more performant it is.
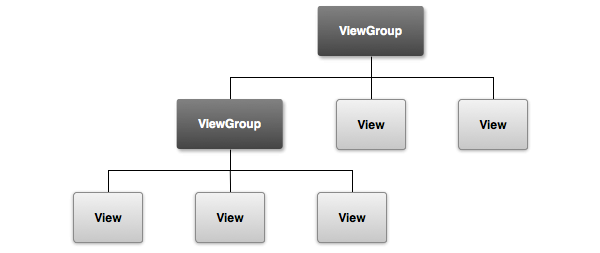
ViewGroup
objects can be instantiated in the same way as standard View
items in XML or in Java code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/hello_world" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" /> <TextView android:id="@+id/hello_world_2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World 2!" /> </LinearLayout>
While all ViewGroup
classes perform a similar task, each ViewGroup
subclass exists for a specific purpose. Let's take a look at some of them.
LinearLayout
The LinearLayout
exists to display items in a stacked order, either horizontally or vertically. Linear layouts can also be used to assign weights to child View
items so that the items are spaced on the screen in a ratio to each other.
RelativeLayout
This ViewGroup
subclass allows you to display items on the screen relative to each other, providing more flexibility and freedom over how your layout appears compared to the LinearLayout
.
FrameLayout
Designed for displaying a single child View
at a time, the FrameLayout
draws items in a stack and provides a simple way to display an item across various screen sizes.
ScrollView
An extension of the FrameLayout
, the ScrollView
class handles scrolling its child objects on the screen.
ViewPager
Used for managing multiple views while only displaying one at a time, the ViewPager
class accepts an Adapter
and allows users to swipe left and right in order to see all available View
items.
RecyclerView
The RecyclerView
class is a ViewGroup
subclass that is related to the ListView
and GridView
classes and that has been made available by Google through the RecyclerView support library for older versions of Android. The RecyclerView
class requires the use of the view holder design pattern for efficient item recycling and it supports the use of a LayoutManager
, a decorator, and an item animator in order to make this component incredibly flexible at the cost of simplicity.
CoordinatorLayout
Recently added to the design support library, the CoordinatorLayout
class uses a Behavior
object to determine how child View
items should be laid out and moved as the user interacts with your app.
3. Custom Views
While there is a wide variety of View
and ViewGroup
classes for you to use within your apps, you sometimes want to create something new to fit your needs. In this case, you can create a new Java class that extends either View
or ViewGroup
, depending on what you need. The act of creating a new custom View
subclass is beyond the scope of this article, but you can find more information in this Envato Tuts+ tutorial.
Conclusion
In this tutorial, you have learned about one of the most basic components of Android, layouts and views. Given how fundamental these components are to Android, you will continuously learn new things about them as you continue to work with the Android platform and you will find new ways to do amazing things using them in your projects.
Comments