A great way to improve your application is by providing an app widget to accompany it. App widgets are simple controls can be placed on places like the Home screen, informing the user of important updates within the application. App widgets remind the user you’re your app exists and help encourage them to use the application more often, among other benefits. In this tutorial, learn to create a simple app widget to enhance to the user’s experience in the Tutlist tutorial application.
App Widgets have been around since API Level 3 (Android 1.5). Over time, features have been added to the app widget framework, but for this tutorial we’ll stick to the basics. For the purposes of this tutorial, we want to design a simple app widget that reminds the user that new tutorials are available to read. Therefore, we will create a widget that displays some information about the latest tutorial. The user can click on the app widget on their Home screen and launch straight into the application.
Step 0: Getting Started
The TutList application is an ongoing reader tutorial project. This tutorial builds the continued series on our TutList application with the most recent tutorial, Android Essentials: ListView Item State Management: A "Read Item" Flag. However, the topic covered, creating an App Widget, could be applied to other apps of your choosing, with some modification. If you have trouble keeping up, feel free to post questions in the comment section -- many folks read and respond, including ourselves. Also, don't forget about the Android SDK reference and more specifically, the App Widgets framework documentation.
The final sample code that accompanies this tutorial is available for browsing and download as open-source from the Google code hosting.
This code listings for the tutorial assumes you will start coding where the previous tutorial in the series, Android Essentials: ListView Item State Management: A "Read Item" Flag, left off. You can download that code and work from there or you can download the code for this tutorial and follow along. If you do work from the previous code, please note that we occasionally make changes outside the scope of any tutorial. Your final result may not look or behave exactly the same. Either way you choose, though, get ready by downloading one or the other project and importing it into Eclipse if you haven't done so already.
Step 1: Create an App Widget Definition
The first thing we need to do is configure an app widget definition file for the app widget. App widget definition files are special XML files that can be stored within the /res/xml project directory and are referenced by the application’s Android manifest file.
Add a file called /res/xml/tutlist_appwidget.xml to your project.
<?xml version="1.0" encoding="utf-8"?> <appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android" android:minWidth="220dp" android:minHeight="72dp" android:updatePeriodMillis="86400000" android:initialLayout="@layout/tutlist_appwidget_layout"> </appwidget-provider>
This app widget configuration file is pretty simple. It defines an app widget (a provider), sets its size (3x1 cells), how often it will update (new articles are released daily, so an interval of 24 hours is reasonable), and specifies the layout resource that defines the content of the app widget.
Note: App widgets should be designed in particular sizes. For more information on app widget design, see the best practices guide on the Android developer website.
Step 2: Designing the App Widget Layout File
Next, we need to create the /layout/titlist_appwidget_layout.xml file we just referenced in the configuration file, and give our app widget some controls. App widgets do not run within the application process, but run remotely. Therefore, app widgets use the RemoteViews class as a basis for their UI so that the controls can be displayed in another process. The RemoteViews class only supports a subset of all view controls you may be used to using within your normal applications, although more controls may be added in the future. Layout controls like FrameLayout, LinearLayout and RelativeLayout are supported. Controls like Button, ImageView, and TextView are supported.
Our simple app widget will include text and an image, so we’re good to go. There’s nothing special about this layout file. It’s simply a RelativeLayout with an ImageView control for the logo and two TextView controls for the tutorial title and excerpt.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/full_widget" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@android:color/white"> <ImageView android:id="@+id/logo" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/tuticon" android:adjustViewBounds="true" android:scaleType="fitXY" android:layout_alignParentLeft="true" android:layout_toRightOf="@id/logo" android:layout_centerHorizontal="true" android:maxWidth="100dp" android:minHeight="100dp" /> <TextView android:id="@+id/label" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_toRightOf="@id/logo" android:textStyle="bold" android:text="@string/appwidget_label" android:textColor="@android:color/black" android:textSize="16dp" android:padding="3dp" /> <TextView android:id="@+id/title" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_toRightOf="@id/logo" android:layout_below="@id/label" android:textSize="14dp" android:padding="3dp" android:textColor="@android:color/black" android:textStyle="italic" /> </RelativeLayout>
Step 3: Implementing the App Widget Provider Class
Now we need to add a class to our application to control the behavior of the app widget. This class needs to extend the AppWidgetProvider class. The main callback of interest is the onUpdate() method. This method needs to grab the latest tutorial title out of the application’s database and update the TextView in the RemoteViews object.
public class TutWidgetProvider extends AppWidgetProvider { public static final String DEBUG_TAG = "TutWidgetProvider"; @Override public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) { try { updateWidgetContent(context, appWidgetManager); } catch (Exception e) { Log.e(DEBUG_TAG, "Failed", e); } } public static void updateWidgetContent(Context context, AppWidgetManager appWidgetManager) { String strLatestTitle = context.getString(R.string.appwidget_no_latest); String [] projection = {TutListDatabase.COL_TITLE}; Uri content = TutListProvider.CONTENT_URI; Cursor cursor = context.getContentResolver().query(content, projection, null, null, TutListDatabase.COL_DATE + " desc LIMIT 1"); if (cursor.moveToFirst()) { strLatestTitle = cursor.getString(0); } cursor.close(); RemoteViews remoteView = new RemoteViews(context.getPackageName(), R.layout.tutlist_appwidget_layout); remoteView.setTextViewText(R.id.title, strLatestTitle); Intent launchAppIntent = new Intent(context, TutListActivity.class); PendingIntent launchAppPendingIntent = PendingIntent.getActivity(context, 0, launchAppIntent, PendingIntent.FLAG_UPDATE_CURRENT); remoteView.setOnClickPendingIntent(R.id.full_widget, launchAppPendingIntent); ComponentName tutListWidget = new ComponentName(context, TutWidgetProvider.class); appWidgetManager.updateAppWidget(tutListWidget, remoteView); } }
The code to update the app widget is pretty straightforward. First we query the application database for the latest title. Then we load up a RemoteViews object from our widget layout, set the TextView control contents to the newest title data. We set the onClick handler for the entire widget (the RelativeLayout) such that clicking it launches the TutList app. Finally, we use call the updateAppWidget() method to commit our changes to the app widget.
In the case of the TutList app, we also want to be able to force an update of the widget contents whenever we download new content from the server (in case the app is out of date). Therefore, we extract most of the widget update code and make it into a public static method that can be called from within the TutListDownloaderService, as needed. You’ll find a simple call to do this in the onPostExecute() method of the DownloaderTask class within TutListDownloaderService.
TutWidgetProvider.updateWidgetContent(context, AppWidgetManager.getInstance(context));
Step 5: Registering the App Widget in the Manifest File
Finally, you need to register the app widget in your application Manifest file. To do this, you will use the tag. This block of XML should be placed inside the tag at the same level as tags.
<receiver android:name="com.mamlambo.tutorial.tutwidget.TutWidgetProvider"> <intent-filter> <action android:name="android.appwidget.action.APPWIDGET_UPDATE" /> </intent-filter> <meta-data android:name="android.appwidget.provider" android:resource="@xml/tutlist_appwidget" /> </receiver>
Note that the receiver name is the name of your app widget provider class implementation. You add an intent filter for the APPWIDGET_UPDATE event such that your widget will update at regular intervals. Finally, you specify the XML configuration file where you configured your app widget settings.
If you run your app, you will now have an app widget! From the Home screen, press and hold an open area so that the menu pops up, then choose Widgets, TutList. The app widget will then be added to your Home screen, as shown in the figure below.
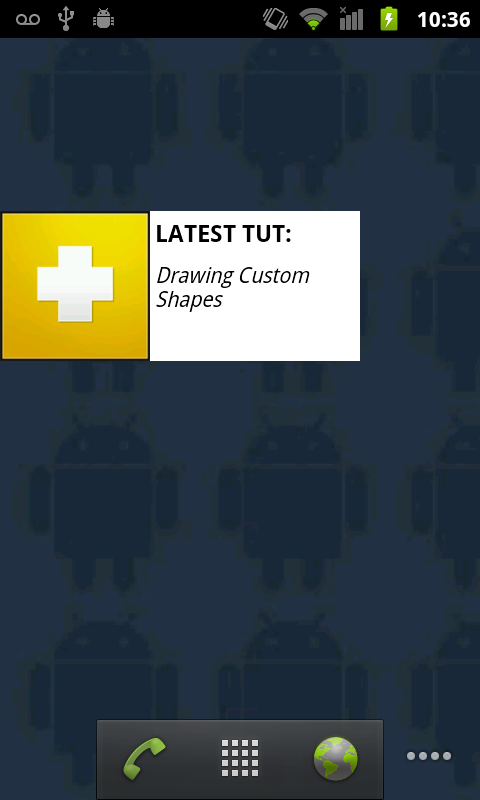
Step 6: New App Widget Features, Now Available for Honeycomb Devices
The latest Android SDK, Honeycomb (API Level 12), introduced a number of interesting new app widget features.
Many of the UI widgets were redesigned for Android 3.0. This enabled more types of controls to be compatible with Remote Views, and therefore capable of living within app widgets. Developers can now include lists, grids and other more complex controls in their app widgets. Another exciting feature introduced in Android 3.1 is the ability for users to resize app widgets both horizontally and vertically, instead of relying on fixed size controls. This is a powerful feature—but developers must update their widgets to support this feature.
Conclusion
In this tutorial, you added a simple app widget to the existing TutList application. You learned create and configure a simple app widget that is compatible with all Android devices currently available to consumers. Also, there are a number of compelling new features recently added the Android SDK’s app widget framework that are beyond the scope of this tutorial, as they are compatible only with the newest Android devices.
As always, we look forward to your feedback.
About the Authors
Mobile developers Lauren Darcey and Shane Conder have coauthored several books on Android development: an in-depth programming book entitled Android Wireless Application Development, Second Edition and Sams Teach Yourself Android Application Development in 24 Hours, Second Edition. When not writing, they spend their time developing mobile software at their company and providing consulting services. They can be reached at via email to [email protected], via their blog at androidbook.blogspot.com, and on Twitter @androidwireless.
Comments